In this Python tutorial, we will learn about how to create Python Literal in Python and we will also cover different examples related to Python Literal. And, we will cover these topics.
- Python Literals
- String Literals
- Numeric Literals
- Boolean Literals
- Special Literals
- Literal Collections
Python Literals
Before moving forward we should have some piece of knowledge about literals. Literals are the items that represent the fixed value in the code. It is also defined as a raw value or data that is given in a variable or constant.
Examples:
In the following example, we create a raw value that is given in a variable and constant.
a = 28
b = 25.3
c = 4+7j
print(a, b, c)
Output:
After running the above code we get the following output in which we can see the data we get in the form of variables.
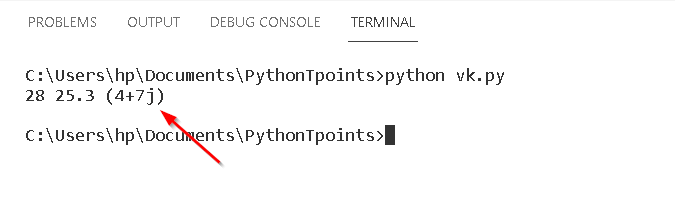
String Literals
String literals can produce by enclosing the text in a single(‘ ‘), double(” “), and triple quotes(”’ ”’).By using the triple quotes(”’ ”’) the text can be written in multiple lines.
Examples:
In the following example, we creating string literals in which text are enclosed in single(‘ ‘),double(” “), and triple(”’ ”’) quotes.
a = 'Pythontpoint'
b = "Pythontpoint"
c = '''Python
t
points'''
print(a)
print(b)
print(c)
Output:
After running the above code we get the following output in which we can see string literals is shown on the command prompt.

Numeric Literals
There are three types of numerical literals
- Integer
- Float
- Complex
Integer
An integer is defined as both positive or negative numbers including 0. It does not include any fractional number.
Example:
In the following example, we create integer literals.
- i= 0b101001 this is binary literal.
- j = 30 this is decimal literal.
- k = 0o350 this is octal literal.
- l = 0x15b this is hexadecimal literal.
i= 0b101001
j = 30
k = 0o350
l = 0x15b
print(i, j, k, l)
Output:
After running the above code we get the following output in which we can see integer literal is formed.
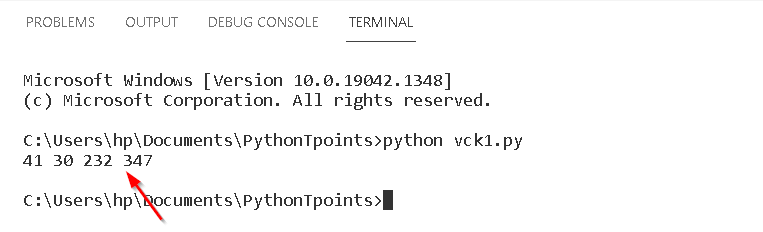
Float
Float literal is defined as real numbers which contain both integer or fractional values.
Examples:
i = 20.8
j = 40.0
print(i, j)
Output:
After running the above code we get the following output in which we can see that float literals are created.
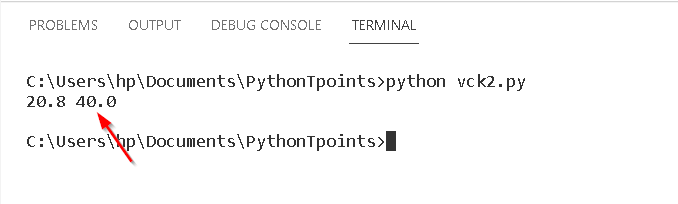
Complex:
Complex literals are defined as in which the numerical consist of both real and complex parts. Complex literal is in the form of a+bi where a is the real part and b is the complex part.
Example:
In this example, we created complex literals which consist of both real and complex parts.
a = 2 + 3j in this 2 is the real part and 3 is the complex part.
b = 7j here real part is 0 and only complex part is present.
a = 2 + 3j
# real part is 0 here.
b = 7j
print(a, b)
Output:
After running the above code we get the following output in which we can see that complex literal is formed.
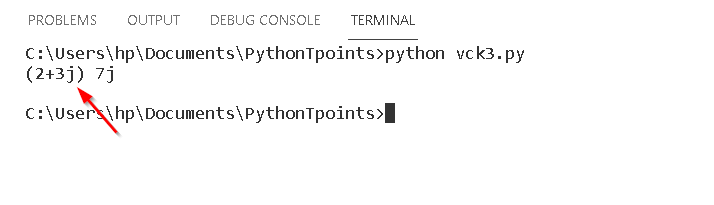
Boolean Literals
Boolean literals are defined as a binary number that has one or two possible values. They are true or false.
Examples:
i = (1 == True)
j = (1 == False)
k = True + 5
l = False + 9
print("i is", i)
print("j is", j)
print("k:", k)
print("l:", l)
Output:
After running the above code we get the following output in which we can see the variable i is true and variable j is false. In boolean true is represented by 1 and false is represented by 0.
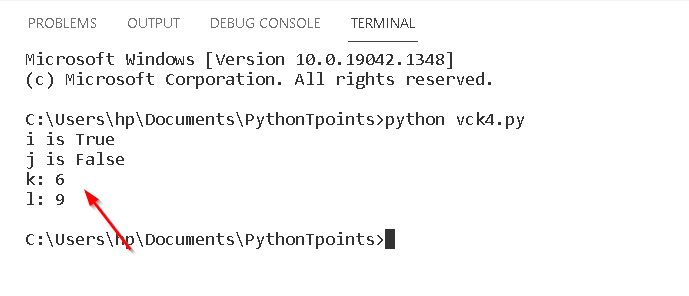
Special Literals
Python has a special literal called ‘None’.None is used for defining the null values. None return a false value.
Examples:
special_literal = None
print(special_literal)
Output:
After running the above code we get the following output in which we can see a special literal is shown on the command prompt.
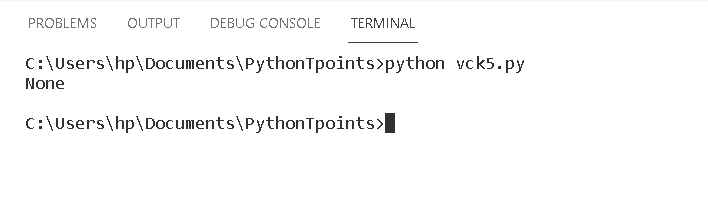
Literal Collections
Literal collections are of for types:
- List Literals
- Dictionary Literals
- Tuple literals
- Set Literals
List Literals
List are mutable and contain all items of different data types. It separated all items by commas (,)and enclose them with square brackets ( [] ).
Example
numbers = [1, 2, 3, 4, 5]
names = ['Michael M Duckett', 'Charles S Jewell', 'Mark B Williams', 5]
print(numbers)
print(names)
Output:
After running the above code we get the following output in which we can list containing the item separated by commas and enclosed with square brackets.
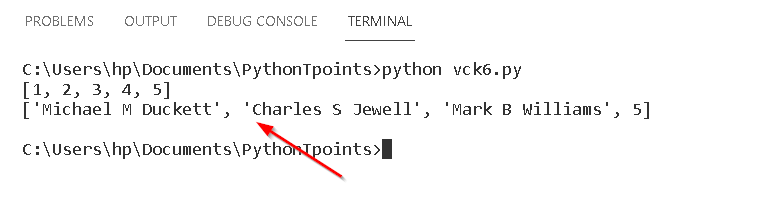
Dictionary Literals
Dictionary contains the data in the form of key pair. The item present in the dictionary is separated by commas(,) and enclosed by curly braces.
Example
alphabet = {'a': 'Dog', 'b': 'Ant', 'c': 'Mango'}
personal_information = {'name': 'Brad D Green', 'age': 25, 'ID': 25}
print(alphabet)
print(personal_information)
Output
After running the above code we get the following output in which we can see the data is in the form of key-pair separated with commas and enclosed with curly braces.
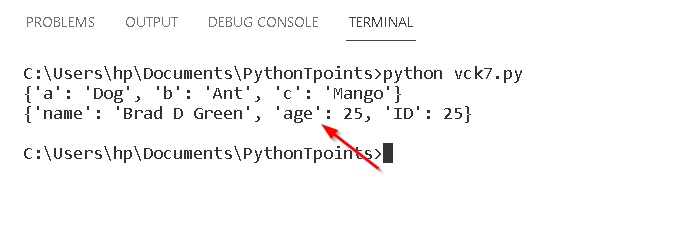
Set Literals
Set is defined as the collection of unordered data set. It is the same as a dictionary which separate the item by commas(,) and enclosed the item with curly braces ( { } ).
Example
vowels = {'a', 'e', 'i', 'o', 'u'}
fruits = {"orange", "grapes", "mango"}
print(vowels)
print(fruits)
Output
After running the above code we get the following output in which we can show the set is created.The item present inside the set separate by commas and enclosed with square bracket( { } ).
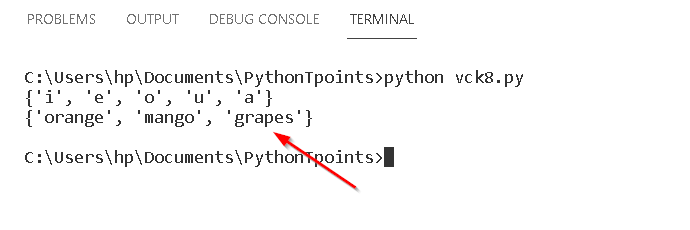
So, in this tutorial, discussed Python Literals and we have also covered different examples related to its implementation. Here is the list of examples that we have covered.
- Python Literals
- String Literals
- Numeric Literals
- Boolean Literals
- Special Literals
- Literal Collections
Do Follow:
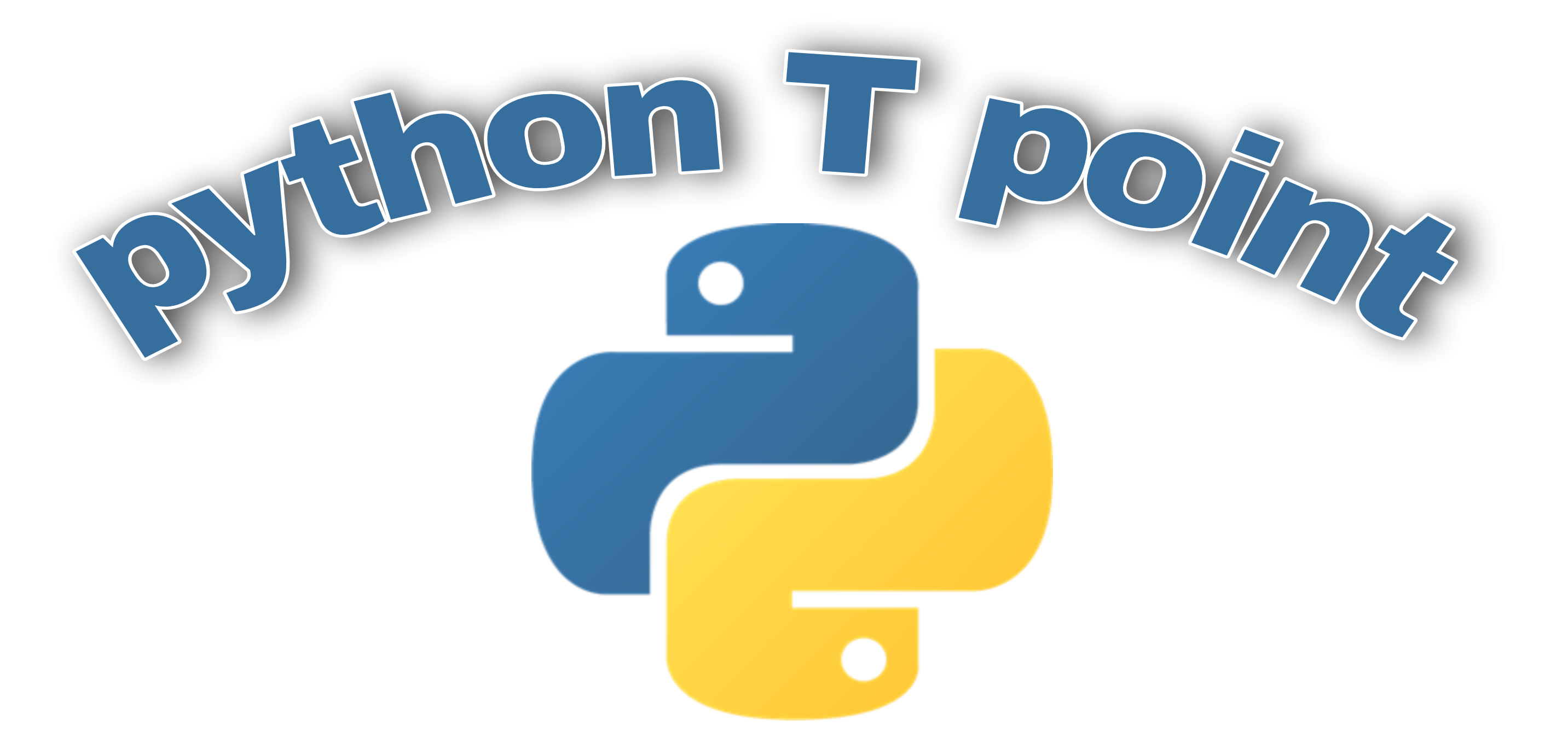