In this tutorial, we will learn How to Write Happy Birthday using a python turtle and we will also cover the different examples related to the python turtle. Besides this, we will also cover the whole code related to How to write happy birthday using python turtle.
How to Write Happy Birthday using Python Turtle
In this section we will learn how to write happy birthday using Python turtle. The turtle is work as a pen from which we can write happy birthday on the screen.
Block of Code:
In this block of code, we will import the turtle library as from turtle import *, and import turtle as t from which we can write the happy birthday code.
#How to Write Happy Birthday using Python Turtle
# Importing turle library
from turtle import *
import turtle as t
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of Code:
In this block of code, we are giving the title to the window as PythonTPoint and give width, color, speed to turtle.
- turtle.width(8) is used to give the width to the turtle.
- turtle.color(“cyan”) is used to give the cyan color to the turtle.
- turtle.speed(10) is used to give the fast and slow speed to the turtle.
- new.bgcolor(“cyan”) is used to give the background color to the screen.
- turtle.left(180) is used to move the turtle into the left direction.
- turtle.forward(300) is used to move the turtle in the forward direction.
- turtle.right(90) is used to move the turtle in the right direction.
# How to Write Happy Birthday using Python Turtle
turtle=t.turtle()
t.title("PythonTPoint")
turtle.width(8)
turtle.color("cyan")
new=t.getscreen()
turtle.speed(10)
new.bgcolor("cyan")
turtle.left(180)
turtle.penup()
turtle.forward(300)
turtle.right(90)
turtle.forward(100)
turtle.pendown()
Block of Code:
In this block of code, we displaying the letter H with the help of turtle. Here we are using the left and forward directions.
# Display H
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.left(90)
turtle.penup()
turtle.forward(50)
turtle.left(90)
turtle.pendown()
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
Block of Code:
In this block of code, we displaying the letter A with the help of turtle. Here we are using the left, right and forward directions.
# Display A
turtle.penup()
turtle.left(90)
turtle.forward(15)
turtle.pendown()
turtle.left(70)
turtle.forward(110)
turtle.right(70)
turtle.right(70)
turtle.forward(110)
turtle.left(180)
turtle.forward(55)
turtle.left(70)
turtle.forward(38)
turtle.left(70)
turtle.penup()
turtle.forward(55)
turtle.left(110)
turtle.forward(100)
Block of Code:
In this block of code, we displaying the letter P with the help of turtle. Here we are using the left, right and forward directions.
# Display P
turtle.left(90)
turtle.pendown()
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(40)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.left(90)
turtle.penup()
turtle.forward(100)
Block of Code:
In this block of code, we displaying the letter Y with the help of turtle. Here we are using the left and forward directions.
# Display Y
turtle.forward(20)
turtle.pendown()
turtle.left(90)
turtle.forward(50)
turtle.left(30)
turtle.forward(60)
turtle.backward(60)
turtle.right(60)
turtle.forward(60)
turtle.backward(60)
turtle.left(30)
Block of Code:
In this block of code, we will go to home with the help of turtle. We are using the blue background color and for moving backward we are using the turtle.backward() method.
# go to Home
turtle.penup()
turtle.home()
turtle.color("yellow")
new.bgcolor("blue")
# setting second row
turtle.backward(300)
turtle.right(90)
turtle.forward(60)
turtle.left(180)
Block of Code:
In this block of code, we displaying the letter A with the help of turtle. Here we are using the left and forward directions.
# Print D
turtle.backward(150)
turtle.right(90)
turtle.forward(60)
turtle.pendown()
turtle.backward(100)
turtle.right(90)
turtle.forward(10)
turtle.backward(70)
turtle.left(180)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(88)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(70)
turtle.penup()
turtle.home()
How to Write Happy Birthday using Python Turtle
Hereafter splitting the code and explaining how to a write Happy Birthday using Python Turtle, now we will see how the output look like after running the whole code.
How to Write Happy Birthday using Python Turtle
How to Write Happy Birthday using Python Turtle
# Importing turle library
from turtle import *
import turtle as t
turtle=t.Turtle()
t.title("Pythontpoint")
turtle.width(8)
turtle.color("cyan")
new=t.getscreen()
turtle.speed(10)
new.bgcolor("cyan")
turtle.left(180)
turtle.penup()
turtle.forward(300)
turtle.right(90)
turtle.forward(100)
turtle.pendown()
# Display H
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.left(90)
turtle.penup()
turtle.forward(50)
turtle.left(90)
turtle.pendown()
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
# Display A
turtle.penup()
turtle.left(90)
turtle.forward(15)
turtle.pendown()
turtle.left(70)
turtle.forward(110)
turtle.right(70)
turtle.right(70)
turtle.forward(110)
turtle.left(180)
turtle.forward(55)
turtle.left(70)
turtle.forward(38)
turtle.left(70)
turtle.penup()
turtle.forward(55)
turtle.left(110)
turtle.forward(100)
# Display P
turtle.left(90)
turtle.pendown()
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(40)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.left(90)
turtle.penup()
turtle.forward(100)
# Display P
turtle.left(90)
turtle.pendown()
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(40)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(50)
turtle.left(90)
turtle.forward(50)
turtle.left(90)
turtle.penup()
turtle.forward(100)
# Display Y
turtle.forward(20)
turtle.pendown()
turtle.left(90)
turtle.forward(50)
turtle.left(30)
turtle.forward(60)
turtle.backward(60)
turtle.right(60)
turtle.forward(60)
turtle.backward(60)
turtle.left(30)
# go to Home
turtle.penup()
turtle.home()
turtle.color("yellow")
new.bgcolor("blue")
# setting second row
turtle.backward(300)
turtle.right(90)
turtle.forward(60)
turtle.left(180)
# Print P
turtle.pendown()
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(40)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(50)
turtle.backward(50)
turtle.left(180)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(40)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(50)
turtle.right(90)
turtle.forward(10)
# go to Home
turtle.penup()
turtle.home()
# setting up
turtle.backward(200)
turtle.right(90)
turtle.forward(10)
turtle.left(90)
turtle.pendown()
turtle.forward(20)
turtle.penup()
turtle.home()
# Print D
turtle.backward(150)
turtle.right(90)
turtle.forward(60)
turtle.pendown()
turtle.backward(100)
turtle.right(90)
turtle.forward(10)
turtle.backward(70)
turtle.left(180)
turtle.right(20)
turtle.forward(20)
turtle.right(70)
turtle.forward(88)
turtle.right(70)
turtle.forward(20)
turtle.right(20)
turtle.forward(70)
turtle.penup()
turtle.home()
# setting up for A
turtle.backward(50)
turtle.right(90)
turtle.forward(65)
turtle.left(90)
# print A
turtle.pendown()
turtle.left(70)
turtle.forward(110)
turtle.right(70)
turtle.right(70)
turtle.forward(110)
turtle.left(180)
turtle.forward(55)
turtle.left(70)
turtle.forward(38)
turtle.left(70)
turtle.penup()
turtle.forward(55)
turtle.left(110)
turtle.forward(100)
# print Y
turtle.pendown()
turtle.left(90)
turtle.forward(50)
turtle.left(30)
turtle.forward(60)
turtle.backward(60)
turtle.right(60)
turtle.forward(60)
turtle.backward(60)
turtle.left(30)
# go to Home
turtle.penup()
turtle.home()
# settig the position
turtle.right(90)
turtle.forward(215)
turtle.right(90)
turtle.forward(200)
turtle.right(90)
# color the letter
turtle.color("light blue")
new.bgcolor("yellow")
# setup
turtle.penup()
turtle.left(90)
turtle.forward(80)
turtle.left(90)
turtle.forward(7)
turtle.forward(100)
# design
#design pattern
turtle.home()
turtle.forward(200)
turtle.pendown()
turtle.color("orange")
turtle.width(3)
turtle.speed(0)
def squre(len, ang):
turtle.forward(len)
turtle.right(ang)
turtle.forward(len)
turtle.right(ang)
turtle.forward(len)
turtle.right(ang)
turtle.forward(len)
turtle.right(ang)
squre(80, 90)
for i in range(36):
turtle.right(10)
squre(80, 90)
t.mainloop()
Output:
After running the whole code we get the following output in which we can see the Happy Birthday is written on the screen with the help of Python Turtle on the screen.

So, in this tutorial, we have illustrated How to write Happy Birthday Using Python Turtle. Moreover, we have also discussed the whole code used in this tutorial.
Read some more tutorials related to Python Turtle.
How to Make a Dog Face Using Python Turtle
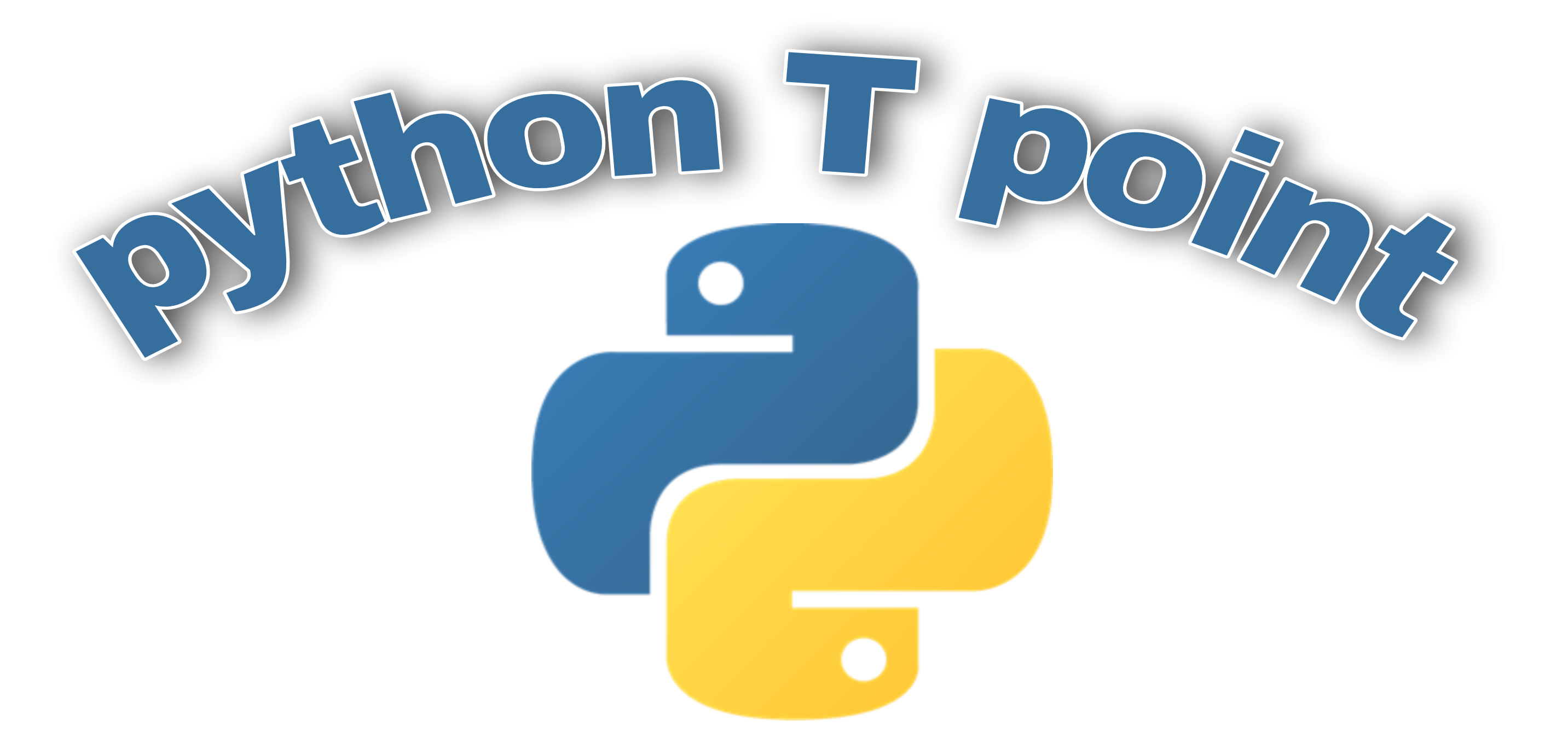