In this python tutorial, we will learn how to draw the different shapes with the help of a circle in a Python turtle and we will also cover different examples related to the Turtle circle. And, we will cover these topics.
- Python turtle circle
- Python turtle circle code
- Python turtle circle clockwise
- Python turtle circle spiral
- Python turtle circle fill color
- Python turtle circle arc
- Python turtle circle method
- Python turtle circle spirograph
Python turtle circle
In python turtle, we use turtle from drawing different shapes, pictures, and making designs. Here turtle is working as a pen that can draw pictures on the screen and here screen works as a drawing board. We can also draw a circle shape with the help of a turtle on the screen.
Code:
In this code, we will import the turtle module for drawing the shape of the circle. The turtle() method is used to make shapes.
- turtl.title(“Pythontpoint”) is used to give the title of the window.
- turtl.circle(100) is used to draw the circle with a radius of 100 pixels.
from turtle import *
import turtle as turtl
turtl.title("Pythontpoint")
turtl.circle(100)
turtl.done()
Output:
After running the above code we get the following output in which we can see that the shape of the circle is drawn on the screen.

Also, check: Python Turtle speed
Python turtle circle code
As we know turtle can draw any type of shape on the screen. Here we can draw a circle shape with the help of turt.circle() function.We can put the radius in the argument to draw the shape of a circle.
Code:
In the following code, we will import the turtle module from which we can draw the different shapes on the screen.
- turt.title(“Pythontpoint”) is used to give the title to the window.
- turt.circle(90) is used to draw the circle of radius 80 pixels.
from turtle import *
import turtle as turt
turt.title("Pythontpoint")
# draw circle of radius
# 80 pixel
turt.circle(90)
turt.done()
Output:
After running the above code we get the following output in which we can see that a circle is drawn with the help of tur.circle() function.

Python turtle circle clockwise
Turtle in python is used to perform different methods. Here it can use for drawing different shapes it works as a pen. As we can draw write, draw, color with the help of a pen, like a pen turtle also can do all the things.
Here we can draw a shape of a circle whose moving in the clockwise direction with the help of turt.circle() function.
Code:
In the following code, we will import the turtle library from which we can draw a clockwise circle on the screen.
- turt.title(“Pythontpoint”) is used to give the title to the window.
- turt.circle(-120) is used to draw the shape of a circle . Here -120 is used to move the circle in a clockwise direction.
from turtle import *
import turtle as turt
turt.title("Pythontpoint")
turt.circle(-120)
turt.done()
Output:
After running the above code we get the following output in which we can see that the circle is over in the clockwise direction with the help of the turtle.

Python turtle circle spiral
A Spiral is defined as a circular thread that can be rounded over and over and took the form of a spiral. In Python, a turtle spiral is drawn with the help of a turtle on the screen. Here the screen works as a drawing board where our turtle circle spiran is drawn.
Code:
In the following code, we will import the turtle module from which we draw the spiral circle on the screen.
- The turtle() method is used o make objects.
- turt.title(“Pythontpoint”) is used to give the title to the window.
- tur.circle(radius + i, 45) is used to draw the shape of the circle with the help of a turtle.
from turtle import *
import turtle as turt
turt.title("Pythontpoint")
tur = turt.Turtle()
radius = 15
for i in range(150):
tur.circle(radius + i, 45)
Output:
After running the above code we get the following output in which we can see that the spiral circle is drawn on the screen with the help of a turtle.
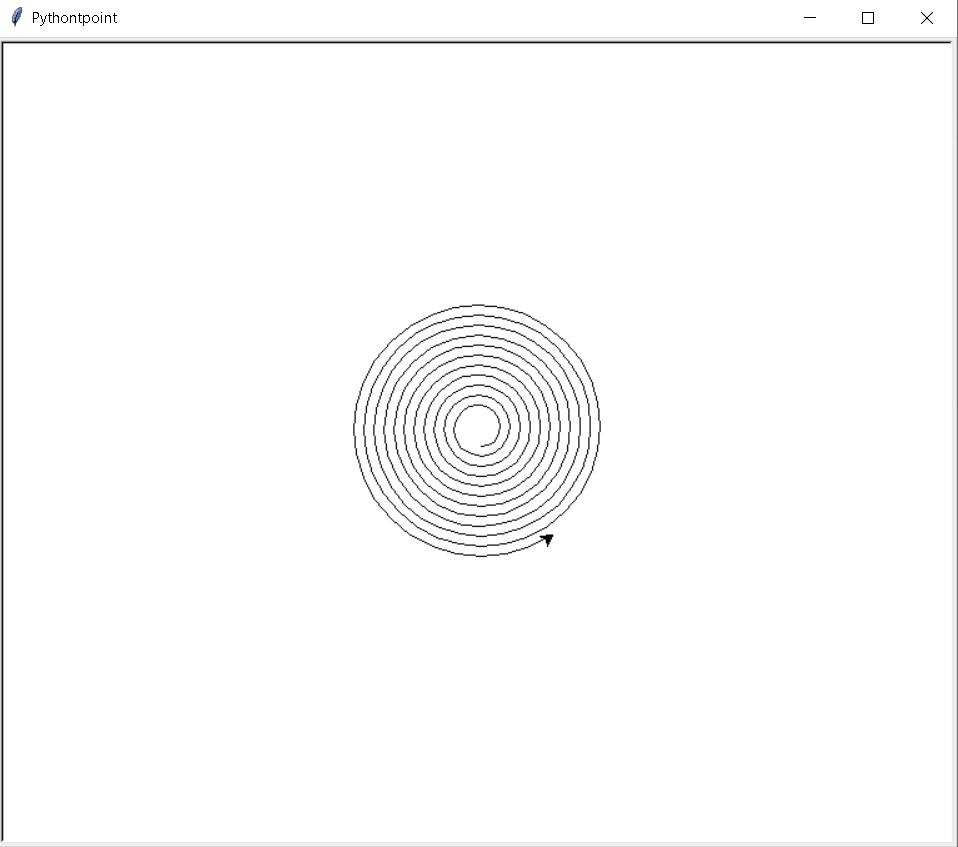
Python turtle circle fill color
In Python turtle, the turtle is used to draw the different shapes and we can also fill colors inside the shapes from which our shape looks very beautiful and attractive. Here we can draw the circle with the help of a turtle and also fill color inside the circle with the help of turt.fillcolor() function.
Code:
In the following code, we will import the turtle module from which we can draw the shape and also fill the color inside the shape.
- The turtle() method is used to make objects.
- turt.fillcolor(“light blue”) is used to fill the color inside the circle.
- turt.begin_fill() is used to start filling color.
- turt.circle(120) is used to draw the shape of a circle.
- turt.end_fill() is used to stop filling color.
from turtle import *
import turtle as turtl
turtl.title("Pythontpoint")
turt = turtl.Turtle()
turt.fillcolor("light blue")
turt.begin_fill()
turt.circle(120)
turt.end_fill()
turtl.done()
Output:
After running the above code we get the following outp[ut in which we can see that a circle shape and a beautiful light blue color is filled inside the circle.

Python turtle circle arc
A circle arc is defined as a part of a segment of the circumference of a circle. Here the turtle is used to draw the arc of a circle on the screen.
Code:
In the following code, we will import the turtle module from which we can draw the circle arc with the help of the turtle.
- turtle.title(“Pythontpoint”) is used to give the title on the screen.
- pencolor(‘blue’) is used to give the color to the pen.
from turtle import *
import turtle
turtle.title("Pythontpoint")
width = 60
r = 360
ystart = -r/2
pensize(width+1)
# Draw blue half-circle
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('blue')
circle(r,180)
# Draw indigo half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('indigo')
circle(r,180)
# Draw light blue half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('light blue')
circle(r,180)
# Draw green half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('green')
circle(r,180)
# Draw yellow half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('yellow')
circle(r,180)
# Draw orange half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('orange')
circle(r,180)
# Draw red half-circle
r -= width
penup()
setposition(r, ystart)
setheading(90)
pendown()
pencolor('red')
circle(r,180)
# Finish
hideturtle()
turtle.done()
Output:
After running the above code we get the following output in which we can see that beautiful arcs are drawn on the screen with the help of a turtle.

Python turtle circle method
In the python turtle we can draw different shapes with the help of the turtle we can also draw the shape of a circle with the help of the turtle. We can draw the circle shape with the help turt.circle() method we can put the radius inside the argument to draw the circle.
Code:
In the following code, we will import the turtle module from which we can draw the shape of a circle on the screen.
- turt.title(“Pythontpoint”) is used to give the title to the window.
- turt.circle(50) is used to draw the shape of a circle on the screen.
from turtle import *
import turtle as turt
turt.title("Pythontpoint")
turt.circle(50)
turt.done()
Output:
After running the above code we get the following output in which we can see the circle is drawn on the screen with the help of turt.circle() function.
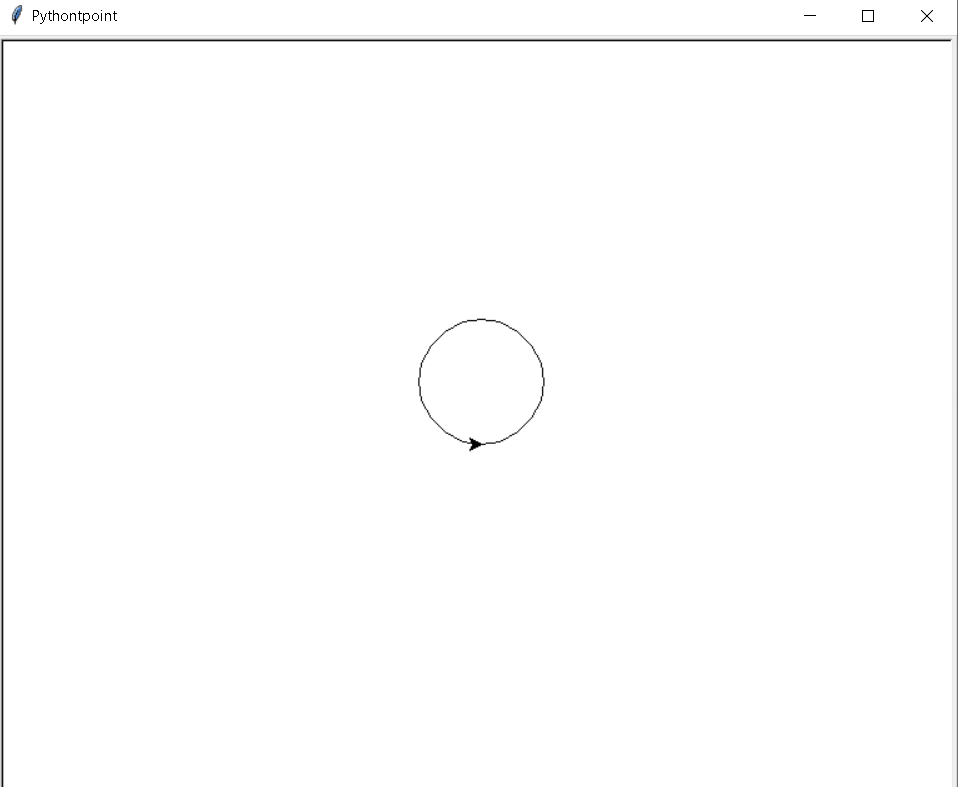
Python turtle circle spirograph
Spirograph is used to draw a beautiful designer curved shaped circle. In science language, it is an instrument used to record the movement of breathing. Here we can draw the circle spirograph with the help of a turtle on the screen.
Code:
In the following code, we will import the turtle module from which we can draw the circle spirograph with the help of the turtle. The turtle() method is used to make objects.
- turt.title(“Pythontpoint”) is used to give the title to the window.
- turt.bgcolor(‘black’) is used to give the color to the background.
- turt.pensize(6) is used to give the size to the pen.
- turt.circle(150) is used to draw the shape of a circle on the screen.
- turt.left(12) is used to move the turtle in the left direction.
from turtle import *
import turtle as turt
turt.title("Pythontpoint")
turt.bgcolor('black')
turt.pensize(6)
turt.speed(10)
for i in range(6):
for color in ('cyan', 'yellow', 'pink',
'red', 'green', 'blue',
'purple'):
turt.color(color)
turt.circle(150)
turt.left(12)
turt.hideturtle()
Output:
After running the above code we get the following output in which we can see that a beautiful colored spirograph is drawn on the screen.

So, in this tutorial, we discussed Python Turtle Circle and we have also covered different examples related to its implementation. Here is the list of examples that we have covered.
- Python turtle circle
- Python turtle circle code
- Python turtle circle clockwise
- Python turtle circle spiral
- Python turtle circle fill color
- Python turtle circle arc
- Python turtle circle method
- Python turtle circle spirograph
Do follow the following tutorials also:
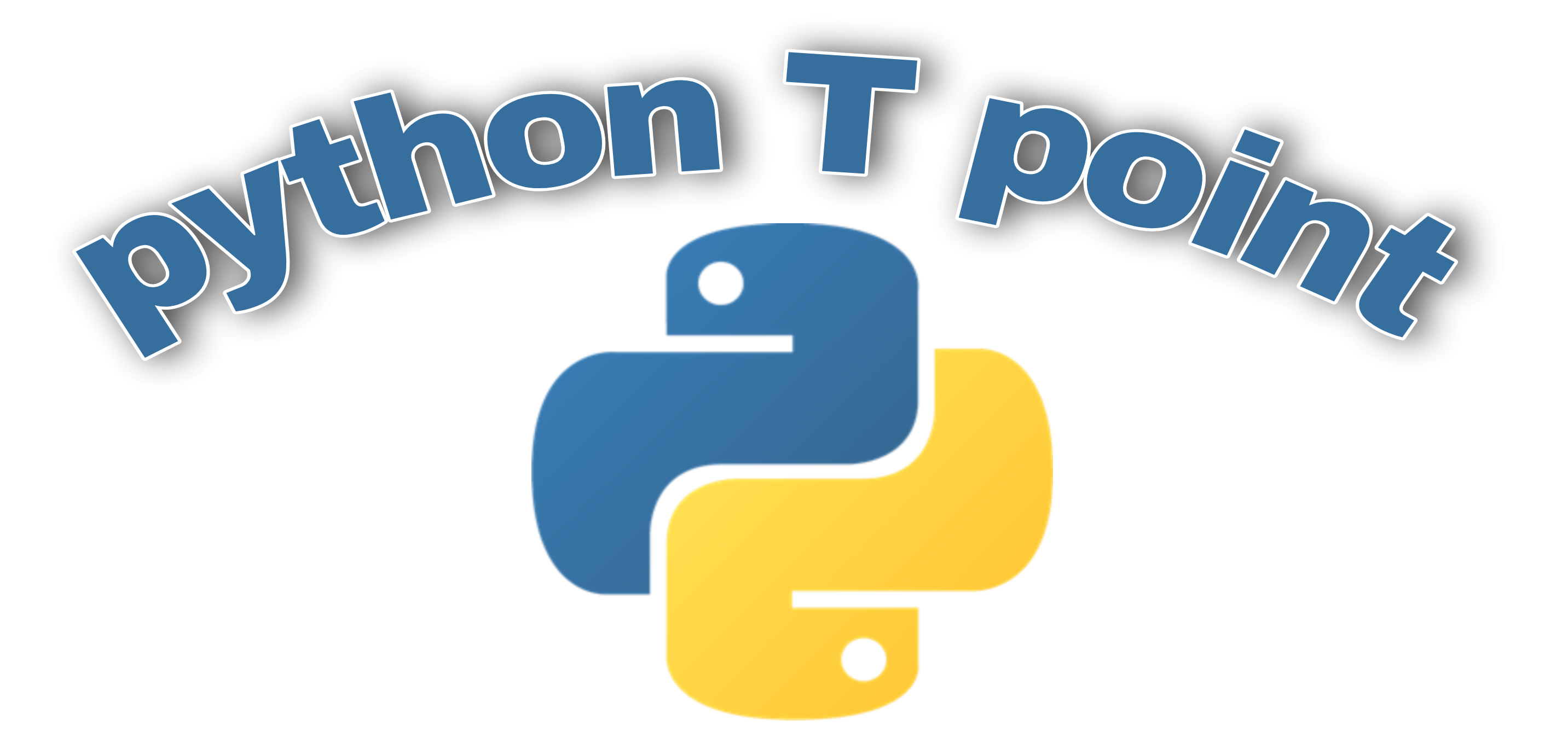
Comments are closed.