In this tutorial, we are learning about python variables where we will learn about the variables and their identifiers, name, reference creating a variable, and deleting the variable. Python is a highly object-oriented programming language. Variables are the name that we used to refer to the memory location.
Python Variables are used to hold the value and we can also call it an Identifier. We do not require to give any specific type of variable in python because python is smart enough to get its variable type.
Python Variable name can consist of both letter and digit, but it should need to begin with the underscore( _ ) or a letter.
We recommend using the lower case to assign the variable taking an example PYTHON and python both are different variables.
- Python Object Reference
- Python Object Identity
- Python Variable Name
- Python Multiple Assignment
- Python Variable Types
- Deleting a Variable in Python
Python Object Reference
Object Reference is that where the memory location for an object is stored. It is necessary to understand how the interpreter works in python to declare the variable.
print("PythonTPoint")
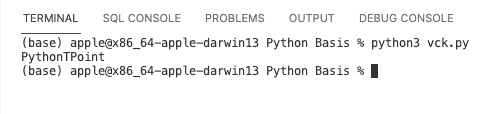
In the above output, we can see that the python object has created an integer object and displayed the output of an integer on the screen.
Python Object Identity
In python, every object has its unique identity. Python takes the responsibility to make sure that there is no same identifier in two objects it uses the built-in function id() which use to identify the uniqueness of an object.
Code:
In the following code, we have given an example of python object identity where we have given the value of p=20 and assigned the same value to y and we reassigned the same variable p and gave a new object identifier.
p = 20
y = p
print(id(p))
print(id(y))
# Reassigned variable p
p = 100
print(id(p))
In the above code, we have assigned p=y where we have given the value of p=20 and assigned the same value to y and we reassigned the same variable p and gave a new object identifier.
Output:
After running the following code we get the following output in which we can see that to the variables p=20 and p=y we get the same object ID and to reassigned variable p we get the different object ID.
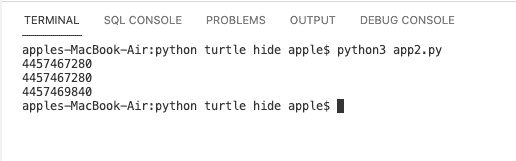
Python Variable Name
In the above topics, we have learned about how we can declare a valid variable. The variable name can be very lengthy and can be of both uppercase and lowercase (A-Z, a-z).
Code:
In the below code we will explain how we can assign the valid variable and give the assigned value to the variable.
pythontpoint = "P"
Pythontpoint = "Y"
pythonTpoint = "T"
PYTHONTPOINT = "H"
p_y_t_h_o_n_t_p_o_i_n_t= "O"
_pythontpoint= "N "
pythontpoint_ = "T -"
_pythontpoint_ = "P"
python23t08point = "O"
PYTHON="I"
T= "N"
point="T"
print(pythontpoint,Pythontpoint,pythonTpoint,PYTHONTPOINT,p_y_t_h_o_n_t_p_o_i_n_t,_pythontpoint,pythontpoint_,_pythontpoint_,python23t08point,PYTHON,T,point)
Output:
In the following example, we have declared the different valid variables some of which are pythontpoint_, _pythontpoint, p_y_t_h_o_n_t_p_o_i_n_t, and python23t08point but when we read the code it brings confusion and it is difficult to understand so we should make that variable name should be properly described and easy to understand.
We can even create the multi-keywords by using the following methods:
- Camel Case: In the Camel case, each word in the middle begins with the capital letter. For example (pythonTPOint)
- Snake Case: In the Snake case, each words are separated by using the underscore. For example (Python_T_Point)

Multiple Assignment
In Python it is allowed that we can assign the same value to multiple variables using a single statement, this process is known as Multiple Assignment.
We can assign this by using the two different ways one is by assigning the single value to multiple variables and the other is by assigning the multiple values to the multiple variables.
Code:
In the following code, we have created an example of single multiple assignments that will explain to us how we can assign the single value to multiple variables.
p=t=P=2308
print(p)
print(t)
print(P)
Output:
After running the above code we get the following output in which we can see that we get the single value for the three different variables.

Code:
In the following code, we have created an example of multiple assignments that will explain to us how we can assign multiple values to multiple variables.
p,t,P=10,20,50
print (p)
print (t)
print (P)
Output:
After running the above code we get the following output in which we can see that we get the multiple values using the multiple variables.

Python Variable Type
In Python, Variables are of two types which we will explain with an example.
- Local variable
- Global variable
Local Variable:
The Variables that are declared inside the function and their scope are within the function are known as local variables.
Code:
In the following code, we will declare a function of the addition of two numbers and find the sum of two numbers using the local variable where they have scope only within a function.
# Declare a function
def addition():
# local variables Scope only within a function
p = 20
y = 30
sum = p + y
print("The sum is:",sum )
# Call a function
addition()
Output:
After running the following code we get the following output for the sum of two numbers using the addition function.

Global Variable:
Global Variable can be used on both inside and outside of the function by default it is declared outside the function. In python global keyword is used to define the global variable inside the function if we don’t use this it will serve the function as a local variable.
Code:
In the following code, we have declared the global variable “p” and after that, we have defined a function with the name fun(), and under that, we have printed a global variable after this we modified the value and assigned the string value to “p”.
# Declare a variable and initialize it
p = 2308
# Global variable in function
def fun():
# printing a global variable
global p
print(p)
# modifying a global variable
p = 'Welcome To PythonTpoint'
print(p)
fun()
print(p)
Output:
After running the following code we get the following output where we have defined a function with the name fun(), and under that, we have printed a global variable after this we modified the value and assigned the string value to “p”.

Deleting a Variable in Python
In Python, we use the del keyword to delete the variable we use the following syntax to delete the variable.
del<variable_name>
Code:
In the following code, we have assigned the value to “p” and after that, we delete “p” using the del keyword and we get the name error where it shows that the name ‘p’ is not defined.
# Assigning a value to p
p = 2308
print(p)
# deleting a variable.
del p
print(p)
Output:
After running the following code we get the following output where we can see an error is here where it shows that name ‘p’ is not defined.

So In this tutorial, we have discussed the Python Variables and under that, we have covered the following sub-topics using its examples.
- Object Reference
- Object Identity
- Variable Name
- Multiple Assignment
- Python Variable Types
- Deleting a Variable in Python
Do follow

Lovely website! I am loving it!! Will come back again. I am bookmarking your feeds also.