In this tutorial, we will learn about the python keywords which bring a special meaning to the compiler. In this, each keyword has its special meaning and performs a special operation and this keyword can be used as a variable.
List of Keywords
- True
- False
- None
- and
- or
- not
- assert
- def
- class
- break
- if
- else
- elif
- del
- try
- finally
- import
- from
- as
- pass
- return
- global
- with
True
The true keyword represents the boolean true where it checks the condition is true and returns “true”.In this non-zero value is treated as zero.
False
The false keyword represents the boolean false where it checks the condition is false and returns “false”.In this zero value is treated as false.
None
In this keyword, it is treated as a null or a void value if there is an empty list or zero then it can’t be treated as ” none “.
and
It is a logical operator where it checks the condition where both A and B values are true and then it returns the true if anyone’s value is false the result will come up in false. we can follow the truth table:
A | B | A and B |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
or
It is a logical operator where it checks the condition where both A or B values are true and then it returns the true condition it only gets false when both the conditions are false. we can follow the following truth table:
A | B | A or B |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
not
It is a logical operator where if the condition is true it results like false and if the condition is false it results like true we can follow the following truth table for better understanding.
A | Not A |
---|---|
True | False |
False | True |
assert
In python, the assert keyword is used as debugging tool where it checks the errors inside the code if it raises an assertion error it will print the error message on the screen.
Code:
In this code, we have assigned the two variables p and y where p=20 and y=0 we use the assert keyword which will help to check the whole code and raises an error on the screen.
p = 20
y = 0
print('p is dividing by Zero')
assert y != 0
print(p / y)
Output:
After running the following code we get the following output where assertion error is here on a console.
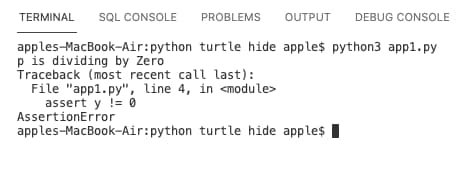
def
In python def keyword is used to declare the function it is declared as the def keyword with the function name.
Code:
In this code, we have declared the function with the name fun() after we have assigned the variables wherein variable “t” we have given an action to add two numbers as we can see “t=p+y” and with fun(23,8) we have assigned the values to the fun(p,y).
def fun(p,y):
t = p+y
print(t)
fun(23,8)
Output:
After running the following output we can see the following output where the fun(p,y) is adding the value and we can see the results inside the console.
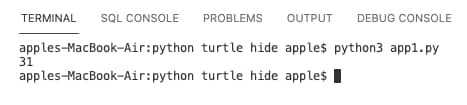
class
In python, class is a blueprint of an object class keyword represent the class in python and it is a set of collections of variables and methods.
Syntax Example:
class example:
#Variables……..
def fun(self):
#statements………
break
In python, the break keyword helps to terminate the loop and transfers the control to the end of the loop.
Example:
for p in range(5):
if(p==3):
break
print(p)
print("End of execution")

if
The ” If ” statement is represented as the conditional statement it helps to execute the particular block which is decided by the if statement.
Example:
i = 18
if (1 < 12):
print("I am less than 18")
Output:

else
The ” else ” statement is used when the if statement returns the false statement then the else block execution is processed in the code.
Example:
p = 19
if(p%2 == 0):
print("The Number is Even")
else:
print("The Number is odd")
Output:

elif
In python, the elif keyword helps to check the multiple conditions, and if the previous statement is false it checks the condition of the next statement and works until the condition gets to be true. It is short of the else-if.
Example:
score = int(input("Enter the score:"))
if(score>=90):
print("Excellent")
elif(score<90 and score>=75):
print("Very Good")
elif(score<75 and score>=60):
print("Good")
else:
print("Average")
Output:

del
In Python, we use the del keyword to delete the variable we use the following syntax to delete the variable.
Syntax:
del<variable_name>
Code:
In the following code, we have assigned the value to “p” and after that, we delete “p” using the del keyword and we get the name error where it shows that the name ‘p’ is not defined.
# Assigning a value to p
p = 2308
print(p)
# deleting a variable.
del p
print(p)
Output:
After running the following code we get the following output where we can see an error is here where it shows that name ‘p’ is not defined.

try, expect
The try, expect is used to handle the exceptions and these exceptions are the run-time errors.
Example:
p = 0
try:
y = 1/p
except Exception as exe:
print(exe)
Output:

finally
In python, the finally keyword is used to create the block of code that is always used to execute where it does not matter that where else block raises any error or not we can consider the following example:
Example:
p=0
y=4
try:
t = y/p
print(t)
except Exception as exe:
print(exe)
finally:
print('Finally always executed')
Output:

import
In python, the import keyword is used to import the modules and inside that module, it contains the runnable python code.
Example:
import math
print(math.sqrt(100))
Output:

from
In python, the from keyword is used to import the specific function into the present python code or a script.
Example:
from math import sqrt
print(sqrt(45))
Output:

as
In python, the as keyword is used as a name alias. It helps us to provide the user-define name while when we import any modules.
Example:
import calendar as cal
print(cal.month_name[6])
Output:

pass
In python, the pass keyword is used when we do nothing or we create a placeholder for us in under processed code. If we declare an empty class it will through an error where here is the use of pass keyword which helps to declare an empty class or a function.
Syntax Example:
class new_class:
pass
def fun():
pass
return
In python, the return keyword is used to return the result value or none to the called function.
Example:
def sum(p,y):
t = p+y
return t
print("The sum is:",sum(25,15))
Output:

global
In python global keyword is used to define the global variable inside the function if we don’t use this it will serve the function as a local variable.
Example:
def fun():
global p
p = 10
y = 20
t = p+y
print(t)
fun()
def function():
print(p)
function()
Output:

with
In python, the with keyword is used while handling the exception it helps to make code cleaner and more readable. The main advantage of the with is that we don’t need to call the close(). We can consider the following example for better understanding.
Example:
with open('file_location', 'w') as file:
file.write('hello Pythontpoint !')
So in this tutorial, we have learned about the python keywords where we choose some main keywords and the list of the explained keywords are mentioned below:
- True
- False
- None
- and
- or
- not
- assert
- def
- class
- break
- if
- else
- elif
- del
- try
- finally
- import
- from
- as
- pass
- return
- global
- with
Do follow:

I simply wanted to write down a message to express gratitude to you for these superb hints you are placing on this website. My time consuming internet investigation has finally been honored with professional content to write about with my friends and family. I would mention that we website visitors actually are very much endowed to exist in a fine network with so many marvellous individuals with good basics. I feel really happy to have seen your entire web pages and look forward to really more brilliant times reading here. Thank you again for all the details.