In this Python tutorial we will learn about Python Arrays and we will also cover different examples related to Python Sets.
- Python array
- Creating an array
- Adding elements to a array
- Accessing elements from the array
- Removing elements from the array
Python array
In Python array is a group of items supply the neighbouring memory location. It can store the multiple items of the identical type. The array can make it simple to compute the position of each element.
Array can hold in python by simply using a module called as an array. It can be very helpful when we have to operate only a particular data type values.
So, with this, we have learned about the array which can be done in the python.
Creating an array
Array can be created in python by simply imported an array module. The array() function is used to create an array with data type in its argument.
Example:
In the following example we are creating an array and the array is created by simply using an array() function.
# Python program to demonstrate Create of Array
# Import array library
import array as arr
# creating an array with an integer type
m = arr.array('i', [2, 4, 6])
# printing an original array
print ("New created array is : ", end =" ")
for i in range (0, 3):
print (m[i], end =" ")
print()
# creating an array with double type
n = arr.array('d', [3.5, 4.2, 4.3])
# printing original array
print ("New created array is : ", end =" ")
for i in range (0, 3):
print (n[i], end =" ")
Output:
After running the above code we get the following output in which we can see that the after using the array() function array is created.
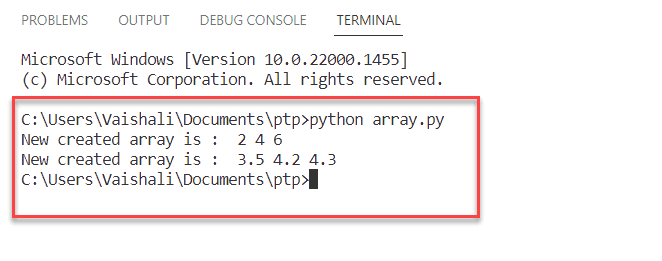
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Adding elements to a array
In python the elements can be added by simply using the insert() function. Insert function is used to add one or more data elements to an array.
Example:
In the following example we are adding the elements to an elements by simply using the insert() function.
- m = arr.array(‘i’, [2, 4, 6,8]) is used as array with the int type.
- print (“Array before insertion : “, end =” “) is used to print the array before insertion.
- m.insert(2, 5) is used to inserting an array using insert() function.
- n = arr.array(‘d’, [3.5, 4.2, 5.3,6.4]) is used an array with float type.
- n.append(5.4) is used as adding an array using append() function.
# Python program to demonstrate Add elements to an Array
# importing an "array" for array creations
import array as arr
# array with int type
m = arr.array('i', [2, 4, 6,8])
print ("Array before insertion : ", end =" ")
for i in range (0, 4):
print (m[i], end =" ")
print()
# inserting array using insert() function
m.insert(2, 5)
print ("Array after insertion : ", end =" ")
for x in (m):
print (x, end =" ")
print()
# array with float type
n = arr.array('d', [3.5, 4.2, 5.3,6.4])
print ("Array before insertion : ", end =" ")
for x in range (0, 4):
print (n[x], end =" ")
print()
# adding an element using append()
n.append(5.4)
print ("Array after insertion : ", end =" ")
for x in (n):
print (x, end =" ")
print()
Output:
In the following output you can see that the array is inserting using the insert() function and append() function.

Read: Python Sets
Accessing elements from the array
In Python the elements of the array can be access to the index number. The index[] operator is used to access an item in an array.
Example:
In the following code we will accessing the elements from the array by using the index[] operator.
- m = ary.array(‘i’, [2, 4, 6, 8, 10, 12]) is used an array with int type.
- print(“Accessing the element is: “, m[1]) is used to print an accessing elements of array.
- n = ary.array(‘d’, [3.5, 4.2, 5.3,6.7]) is used as an array with float type.
# accessing of element from list and importing array module
import array as ary
# array with int type
m = ary.array('i', [2, 4, 6, 8, 10, 12])
# accessing element of array
print("Accessing the element is: ", m[1])
# accessing element of array
print("Accessing the element is: ", m[4])
# array with float type
n = ary.array('d', [3.5, 4.2, 5.3,6.7])
# accessing element of array
print("Accessing the element is: ", n[2])
# accessing element of array
print("Accessing the element is: ", n[3])
Output:
After running the above code we get the following output in which we can see that the elements of an array can be access to the index number.

Read:Python Dictionary
Removing elements from the array
In Python for removing the elements the remove() function is used. The remove() only remove one element at a time.
Here the remove() function in the list only remove the second event of the searched element.
Example:
In the following code we will remove the element from the array by using the remove() function.
- m = array.array(‘i’, [2, 4, 6, 8, 10,12]) is used to initializing the array with the array values.
- print (m.pop(3)) is used as to remove element at the 3rd position.
- m.remove(2) is used remove() to remove 2nd occurrence of 2.
# Python program to demonstrate the removal of elements in a Array
# importing "array" for array operations
import array
# initializing array with array values
m = array.array('i', [2, 4, 6, 8, 10,12])
# printing the original array
print ("The new created array is : ", end ="")
for i in range (0, 6):
print (m[i], end =" ")
print ("\r")
# using pop() to remove element at 3rd position
print ("The pop element is : ", end ="")
print (m.pop(3))
# printing array after popping
print ("The array after popping is : ", end ="")
for i in range (0, 5):
print (m[i], end =" ")
print("\r")
# using remove() to remove 2nd occurrence of 2
m.remove(2)
# printing array after removing
print ("The array after removing is : ", end ="")
for i in range (0, 4):
print (m[i], end =" ")
Output:
After running the above code we get the following output in which we can see that the element are popped and then after popping removing the elements from the array.

So, in this tutorial, we have learned about the Python Arrays and we have covered all these topics.
- Python array
- Creating an array
- Adding elements to a array
- Accessing elements from the array
- Removing elements from the array
Do follow the following tutorials also:
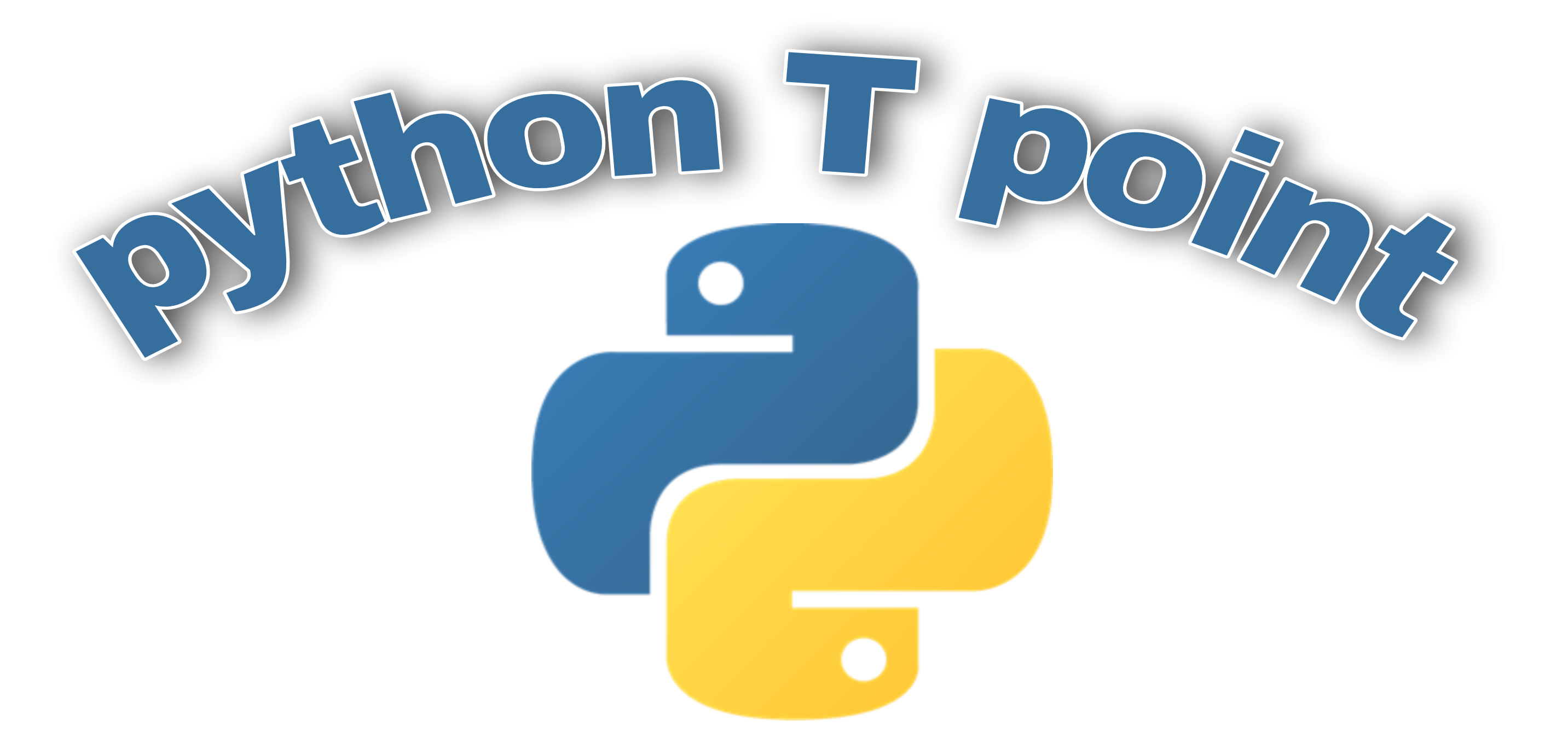
Comments are closed.