In this Python tutorial, we will learn about how to create a Stone Paper Scissor game using Python Tkinter. This game is played by two players it is a hand game and players can form any of the three shapes with the help of their hand.

Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Stone Paper Scissor using Python Tkinter
Block of code:
In this Python Tkinter block of code, we will import the turtle library from which we can make a game Stone Paper Scissor.
- wd = Tk() is used to create an object.
- wd.geometry(“500×300”) is used to give the width and height to the window.
- wd.title(“Pythontpoint”) is used to give the title to the window.
# Import Required Library
from tkinter import *
import random as rand
# Create Object
wd = Tk()
# Set geometry
wd.geometry("500x300")
# Set title
wd.title("Pythontpoint")
Read:
Block of code:
In this Python Tkinter block of code, we give the value to the computer from which the computer plays this game with the player who comes and wants to play this game.
# Computer Value
computervalue = {
"0":"Stone",
"1":"Paper",
"2":"Scissor"
}
Block of code:
In this Python Tkinter block of code, we define a reset game function from which we can reset the game after one turn is completed.
# Reset The Game
def reset_game():
button1["state"] = "active"
button2["state"] = "active"
button3["state"] = "active"
label1.config(text = "Player ")
label3.config(text = "Computer")
label4.config(text = "")
Block of code:
In this Python Tkinter block of code if the player selects the stone and the computer randomly selects the stone then match draw is shown in the result.
label4.config(text = matchresult) is used to give the label that config the match result.
# If player selected Stone
def isStone():
cv = computervalue[str(rand.randint(0,2))]
if cv == "Stone":
matchresult = "Match Draw"
elif cv=="Scissor":
matchresult = "Player Win"
else:
matchresult = "Computer Win"
label4.config(text = matchresult)
label1.config(text = "Stone ")
label3.config(text = cv)
button_disable()
Block of code:
In this Python Tkinter block of code, we see if the player selects paper, and at the same time the computer selects paper then a match is drawn elif the computer selects the scissor at the same time the computer win else player wins the game.
# If player selected paper
def ispaper():
cv = computervalue[str(rand.randint(0, 2))]
if cv == "Paper":
matchresult = "Match Draw"
elif cv=="Scissor":
matchresult = "Computer Win"
else:
matchresult = "Player Win"
label4.config(text = matchresult)
label1.config(text = "Paper ")
label3.config(text = cv)
button_disable()
Block of code:
In this Python Tkinter block of code, we create a label to give the perfect information about the thing.
- Label(wd, text = “Stone Paper Scissor”,font = “normal 22 bold”,fg = “blue”).pack(pady = 20) is used to give the information about the game.
- frame = Frame(wd) is used to create a frame on the screen.
Label(wd,
text = "Stone Paper Scissor",
font = "normal 22 bold",
fg = "blue").pack(pady = 20)
frame = Frame(wd)
frame.pack()
label1 = Label(frame,
text = "Player ",
font = 12)
label2 = Label(frame,
text = "VS ",
font = "normal 12 bold")
label3 = Label(frame, text = "Computer", font = 12)
label1.pack(side = LEFT)
label2.pack(side = LEFT)
label3.pack()
label4 = Label(wd,
text = "",
font = "normal 25 bold",
bg = "white",after playe
width = 15 ,
borderwidth = 2,
relief = "solid")
label4.pack(pady = 20)
Block of code:
In this Python Tkinter block of code, we create buttons from which we reset the game after completing one turn simply using the reset button.
button1 = Button(frame1, text = "Stone",
font = 10, width = 7,
command = isStone)
button2 = Button(frame1, text = "Paper ",
font = 10, width = 7,
command = ispaper)
button3 = Button(frame1, text = "Scissor",
font = 10, width = 7,
command = isscissor)
button1.pack(side = LEFT, padx = 10)
button2.pack(side = LEFT,padx = 10)
button3.pack(padx = 10)
Button(wd, text = "Reset Game",
font = 10, fg = "red",
bg = "black", command = reset_game).pack(pady = 20)
Code:
In the following code, we create a game of Stone Paper Scissor using Python Tkinter which is played between two-player one is a player and one is a computer. The player can select one of the three things from their mind and the computer randomly select the thing and the game starts in between the both.
# Import Required Library
from tkinter import *
import random as rand
# Create Object
wd = Tk()
# Set geometry
wd.geometry("500x300")
# Set title
wd.title("Pythontpoint")
# Computer Value
computervalue = {
"0":"Stone",
"1":"Paper",
"2":"Scissor"
}
# Reset The Game
def reset_game():
button1["state"] = "active"
button2["state"] = "active"
button3["state"] = "active"
label1.config(text = "Player ")
label3.config(text = "Computer")
label4.config(text = "")
# Disable the Button
def button_disable():
button1["state"] = "disable"
button2["state"] = "disable"
button3["state"] = "disable"
# If player selected Stone
def isStone():
cv = computervalue[str(rand.randint(0,2))]
if cv == "Stone":
matchresult = "Match Draw"
elif cv=="Scissor":
matchresult = "Player Win"
else:
matchresult = "Computer Win"
label4.config(text = matchresult)
label1.config(text = "Stone ")
label3.config(text = cv)
button_disable()
# If player selected paper
def ispaper():
cv = computervalue[str(rand.randint(0, 2))]
if cv == "Paper":
matchresult = "Match Draw"
elif cv=="Scissor":
matchresult = "Computer Win"
else:
matchresult = "Player Win"
label4.config(text = matchresult)
label1.config(text = "Paper ")
label3.config(text = cv)
button_disable()
# If player selected scissor
def isscissor():
cv = computervalue[str(rand.randint(0,2))]
if cv == "Stone":
matchresult = "Computer Win"
elif cv == "Scissor":
matchresult = "Match Draw"
else:
matchresult = "Player Win"
label4.config(text = matchresult)
label1.config(text = "Scissor ")
label3.config(text = cv)
button_disable()
# Add Labels, Frames and Button
Label(wd,
text = "Stone Paper Scissor",
font = "normal 22 bold",
fg = "blue").pack(pady = 20)
frame = Frame(wd)
frame.pack()
label1 = Label(frame,
text = "Player ",
font = 12)
label2 = Label(frame,
text = "VS ",
font = "normal 12 bold")
label3 = Label(frame, text = "Computer", font = 12)
label1.pack(side = LEFT)
label2.pack(side = LEFT)
label3.pack()
label4 = Label(wd,
text = "",
font = "normal 25 bold",
bg = "white",
width = 15 ,
borderwidth = 2,
relief = "solid")
label4.pack(pady = 20)
frame1 = Frame(wd)
frame1.pack()
button1 = Button(frame1, text = "Stone",
font = 10, width = 7,
command = isStone)
button2 = Button(frame1, text = "Paper ",
font = 10, width = 7,
command = ispaper)
button3 = Button(frame1, text = "Scissor",
font = 10, width = 7,
command = isscissor)
button1.pack(side = LEFT, padx = 10)
button2.pack(side = LEFT,padx = 10)
button3.pack(padx = 10)
Button(wd, text = "Reset Game",
font = 10, fg = "red",
bg = "black", command = reset_game).pack(pady = 20)
# Execute Tkinter
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that a stone Paper Scissor using Python Tkinter game is created in which the player clicks on the Stone Paper Scissor button after clicking on the button the selected option show on the screen after that the computer randomly selects the option and the winner name is highlighted on the screen. After one turn the player clicks on the reset button and the game will reset.

So, in this tutorial, we have illustrated how to create a Stone Paper Scissor game using Python Tkinter. Moreover, we have also discussed the whole code used in this tutorial.
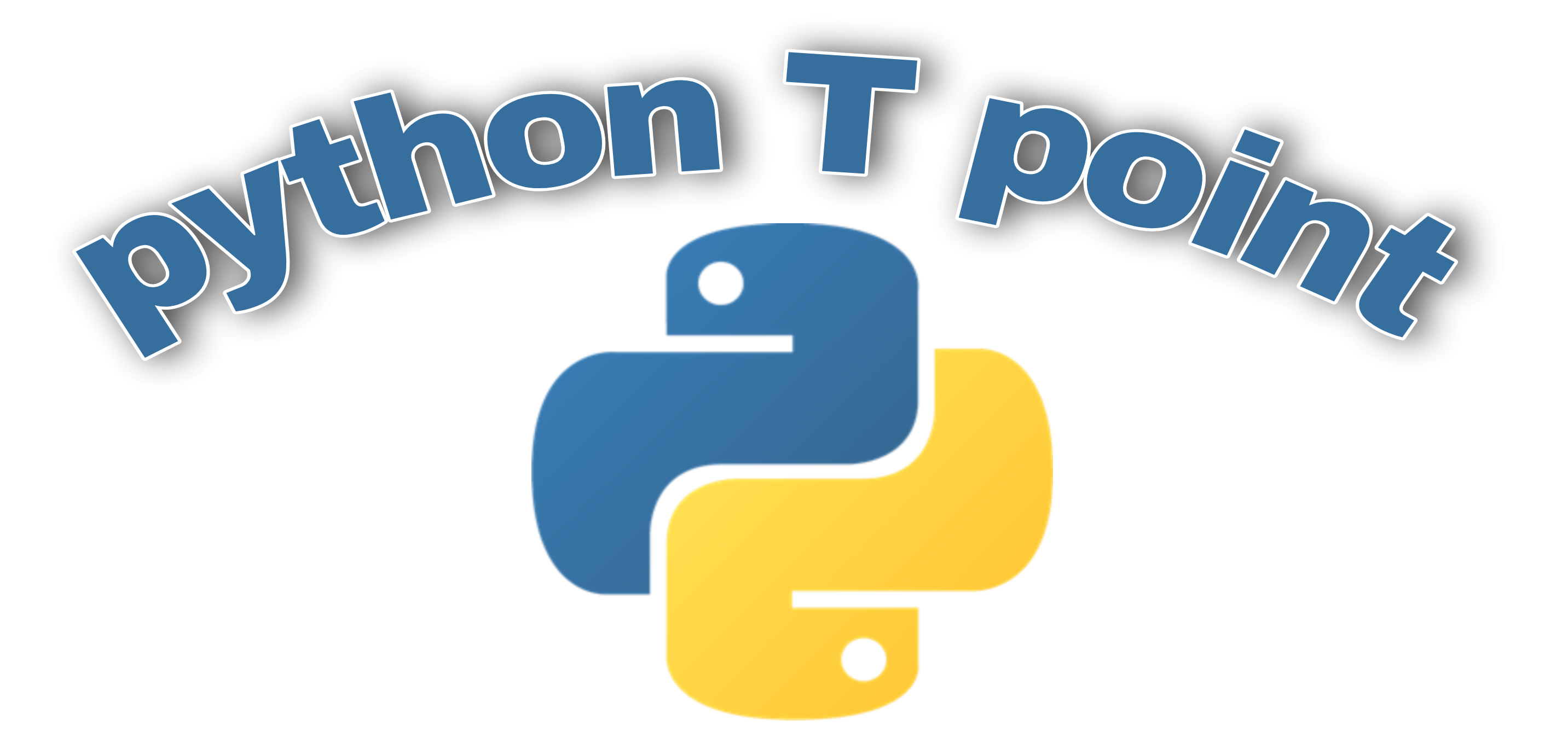
1 thought on “Stone Paper Scissor using Python Tkinter”
Comments are closed.