In this tutorial, we will learn about String in Python and we will also cover different examples related to PythonString. And, we will cover these topics.
- Python String
- Creating String in Python
- Strings indexing and splitting
- Reassigning Strings
- Deleting the String
- String Operators
- Python String Formatting
- The format() method
- Python String functions
Python String
Python String is the group of characters enclosed in form of single quotes, double quotes, and triple quotes. We know that the computer does not understand the human language or the characters that we assigned it converts those characters into the binary form which is 0’s and 1’s.
Every character is encoded into the ASCII and UNICODE character through this we can also say that the python string is the collection of the Unicode characters.
Syntax:
str = "Welcome to Pythontpoint !"
In Python, strings serve as the sequence of characters where python does not support the character datatype, alternatively, if any single character is written ‘p’ then it will count as the length 1.
Revise: Python Datatypes
Creating String in Python
Python string is created by enclosing the character by using the single or the double-quotes it also provides the string to use the triple quotes which are used in multi-line docstrings.
Example:
#Using single quotes
str = 'Hello User'
print(str)
#Using double quotes
str1 = "Welcome to Pythontpoint"
print(str1)
#Using triple quotes
str2 = '''In Python string Triple quotes are generally used for
represent the multiline or
docstring'''
print(str2)
Output:
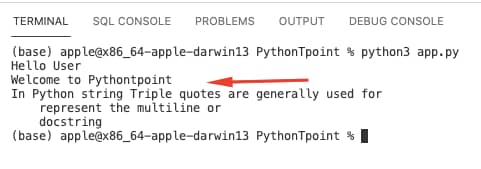
Strings indexing and splitting
String indexing in python starts from 0 like the other programming language. The string Python is indexed in the following way:
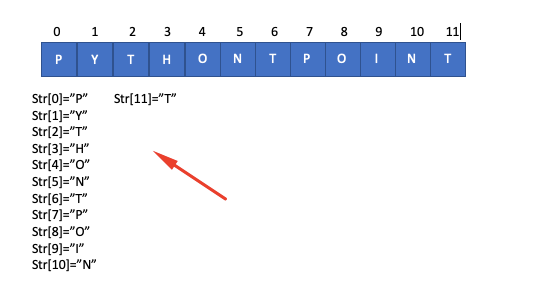
Code:
str = "PYTHONTPOINT"
print(str[0])
print(str[1])
print(str[2])
print(str[3])
print(str[4])
print(str[5])
print(str[6])
print(str[7])
print(str[8])
print(str[9])
print(str[10])
print(str[11])
# It returns the IndexError because 12th index doesn't exist
print(str[12])
Output:

In python, we use the slice operator [ ] that is used to access the individual characters of the string. We can use the colon(: ) operator in python to access the substring from the given string. We can consider the following example:

In the above following example, we can see that the upper range in the slice is always exclusive. if str=’HELLO’, is given str [1:3] will always include str[1] = ‘E’, str[2] = ‘L’ and nothing else.
Code:
# Given String
str = "PYTHONTPOINT"
# Start Oth index to end
print(str[0:])
# Starts 1th index to 4th index
print(str[1:5])
# Starts 2nd index to 3rd index
print(str[2:4])
# Starts 0th to 2nd index
print(str[:3])
#Starts 4th to 6th index
print(str[4:7])
Output:

Reassigning Strings
In python string, it is also easy to update the string as similar to assigning the new string. The strings are immutable and we can change them by making a copy of the string.
Code:
str = "HELLO"
str[0] = "h"
print(str)
Output:

The string str can be assigned completely to new content as specified in the following example.
Example 2:
Code:
str = "HELLO"
print(str)
str = "hello"
print(str)
Output:

Deleting the String
We know that the String is immutable and we can not delete or remove the character in the string but we can delete the whole string using the del keyword.
Example:
str = "PYTHONTPOINT"
del str[1]
Output:

Example 2:
str1 = "PYTHONTPOINT"
del str1
print(str1)
Output:

String Operators
Operator | Description |
---|---|
+ | It is known as the concatenation operator used to join the strings given on either side of the operator. |
* | It is known as the repetition operator. It concatenates multiple copies of the same string. |
[] | It is known as the slice operator. It is used to access the sub-strings of a particular string. |
[:] | It is known as a range slice operator. It is used to access the characters from the specified range. |
in | It is known as a membership operator. It returns if a particular substring is present in the specified string. |
not in | It is also a membership operator and does the exact reverse of in. It returns true if a particular substring is not present in the specified string. |
r/R | It is used to specify the raw string. Raw strings are used in the cases where we need to print the actual meaning of escape characters such as “C://python”. To define any string as a raw string, the character r or R is followed by the string. |
% | It is used to perform string formatting. It makes use of the format specifiers used in C programming like %d or %f to map their values in python. We will discuss how formatting is done in python. |
We can understand more about the python operators using the following examples.
str = "Hello"
str1 = " world"
print(str*3)
print(str+str1)
print(str[4])
print(str[2:4]);
print('w' in str)
print('wo' not in str1)
print(r'C://python37')
print("The string str : %s"%(str))

Python String Formatting
Escape Sequence
In Python string, Let’s consider we need to write the text as – They said, “Hello what’s going on?”- the given statement can be written in single quotes or double quotes but it will raise the SyntaxError as it contains both single and double-quotes.
str = "They said, "Hello what's going on?""
print(str)

Sr. | Escape Sequence | Description | Example |
---|---|---|---|
1. | \newline | It ignores the new line. | print(“Python1 \ Python2 \ Python3″) Output: Python1 Python2 Python3 |
2. | \\ | Backslash | print(“\\”) Output:\ |
3. | \’ | Single Quotes | print(‘\”) Output:‘ |
4. | \\” | Double Quotes | print(“\””) Output:“ |
5. | \a | ASCII Bell | print(“\a”) |
6. | \b | ASCII Backspace(BS) | print(“Hello \b World”) Output: Hello World |
7. | \f | ASCII Formfeed | print(“Hello \f World!”) Hello World! |
8. | \n | ASCII Linefeed | print(“Hello \n World!”) Output: Hello World! |
9. | \r | ASCII Carriege Return(CR) | print(“Hello \r World!”) Output: World! |
10. | \t | ASCII Horizontal Tab | print(“Hello \t World!”) Output: Hello World! |
11. | \v | ASCII Vertical Tab | print(“Hello \v World!”) Output: Hello World! |
12. | \ooo | Character with octal value | print(“\110\145\154\154\157”) Output: Hello |
13 | \xHH | Character with hex value. | print(“\x48\x65\x6c\x6c\x6f”) Output: Hello |
The format() method
In python string, the format method is useful in formating the specified value and inserting that value into the string placeholder.
# Using Curly braces
print("{} and {} both are the best friend".format("Akshat","Achin"))
#Positional Argument
print("{1} and {0} best players ".format("Virat","Dhoni"))
#Keyword Argument
print("{a},{b},{c}".format(a = "James", b = "Van", c = "Root"))

Python String functions
Method | Description |
---|---|
capitalize() | It capitalizes on the first character of the String. This function is deprecated in python3 |
casefold() | It returns a version of s suitable for case-less comparisons. |
center(width ,fillchar) | It returns a space padded string with the original string centered with an equal number of left and right spaces. |
count(string,begin,end) | It counts the number of occurrences of a substring in a string between the beginning and end index. |
decode(encoding = ‘UTF8’, errors = ‘strict’) | Decodes the string using a codec registered for encoding. |
encode() | Encode S using the codec registered for encoding. Default encoding is ‘utf-8’. |
endswith(suffix ,begin=0,end=len(string)) | It returns a Boolean value if the string terminates with a given suffix between begin and end. |
expandtabs(tabsize = 8) | It defines tabs in the string to multiple spaces. The default space value is 8. |
find(substring ,beginIndex, endIndex) | It returns the index value of the string where the substring is found between begin index and end index. |
format(value) | It returns a formatted version of S, using the passed value. |
index(subsring, beginIndex, endIndex) | It throws an exception if the string is not found. It works the same as the find() method. |
isalnum() | It returns true if the characters in the string are alphanumeric i.e., alphabets or numbers and there is at least 1 character. Otherwise, it returns false. |
isalpha() | It returns true if all the characters are alphabets and there is at least one character, otherwise False. |
isdecimal() | It returns true if all the characters of the string are decimals. |
isdigit() | It returns true if all the characters are digits and there is at least one character, otherwise False. |
isidentifier() | It returns true if the string is the valid identifier. |
islower() | It returns true if the characters of a string are in lower case, otherwise false. |
isnumeric() | It returns true if the string contains only numeric characters. |
isprintable() | It returns true if all the characters of s are printable or s is empty, false otherwise. |
isupper() | It returns false if characters of a string are in Upper case, otherwise False. |
isspace() | It returns true if the characters of a string are white-space, otherwise false. |
istitle() | It returns true if the string is titled properly and false otherwise. A title string is the one in which the first character is upper-case whereas the other characters are lower-case. |
isupper() | It returns true if all the characters of the string(if exists) is true otherwise it returns false. |
join(seq) | It merges the strings representation of the given sequence. |
len(string) | It returns the length of a string. |
ljust(width[,fillchar]) | It returns the space padded strings with the original string left-justified to the given width. |
lower() | It converts all the characters of a string to Lower case. |
lstrip() | It removes all leading whitespaces of a string and can also be used to remove a particular character from leading. |
partition() | It searches for the separator sep in S and returns the part before it, the separator itself, and the part after it. If the separator is not found, return S and two empty strings. |
maketrans() | It returns a translation table to be used in translate function. |
replace(old,new[,count]) | It replaces the old sequence of characters with the new sequence. The max characters are replaced if max is given. |
rfind(str,beg=0,end=len(str)) | It is similar to find but it traverses the string in backward direction. |
rindex(str,beg=0,end=len(str)) | It is the same as an index but it traverses the string in a backward direction. |
rjust(width,[,fillchar]) | Returns a space padded string having original string right justified to the number of characters specified. |
rstrip() | It removes all trailing whitespace of a string and can also be used to remove a particular character from trailing. |
rsplit(sep=None, maxsplit = -1) | It is the same as split() but it processes the string from the backward direction. It returns the list of words in the string. If Separator is not specified then the string splits according to the white space. |
split(str,num=string.count(str)) | Splits the string according to the delimiter str. The string splits according to the space if the delimiter is not provided. It returns the list of substring concatenated with the delimiter. |
splitlines(num=string.count(‘\n’)) | It returns the list of strings at each line with newline removed. |
startswith(str,beg=0,end=len(str)) | It returns a Boolean value if the string starts with a given str between begin and end. |
strip([chars]) | It is used to perform lstrip() and rstrip() on the string. |
swapcase() | It inverts the case of all characters in a string. |
title() | It is used to convert the string into the title case i.e., The string meEruT will be converted to Meerut. |
translate(table,deletechars = ”) | It translates the string according to the translation table passed in the function. |
upper() | It converts all the characters of a string to Upper Case. |
zfill(width) | Returns original string left padded with zeros to a total of width characters; intended for numbers, zfill() retains any sign given (less one zero). |
So, in this tutorial, we discussed Python String and we have also covered different examples related to its implementation. Here is the list of examples & topics that we have covered.
- Python String
- Creating String in Python
- Strings indexing and splitting
- Reassigning Strings
- Deleting the String
- String Operators
- Python String Formatting
- The format() method
- Python String functions

I will immediately clutch your rss as I can’t to find your e-mail subscription hyperlink or e-newsletter service. Do you’ve any? Kindly allow me understand in order that I may just subscribe. Thanks.
Greetings! I’ve been reading your site for a while now and finally got the courage to go ahead and give you a shout out from Humble Tx! Just wanted to tell you keep up the excellent work!
Thanks, I’ve recently been looking for info about this topic for ages and yours is the greatest I’ve found out so far. But, what about the bottom line? Are you certain about the supply?