In this tutorial, we will learn about how to draw a Python Turtle circle mania with the help of a turtle in python.
Here we can draw a circle abnormally and we get the beautiful design that is drawn on the screen with the help of a turtle
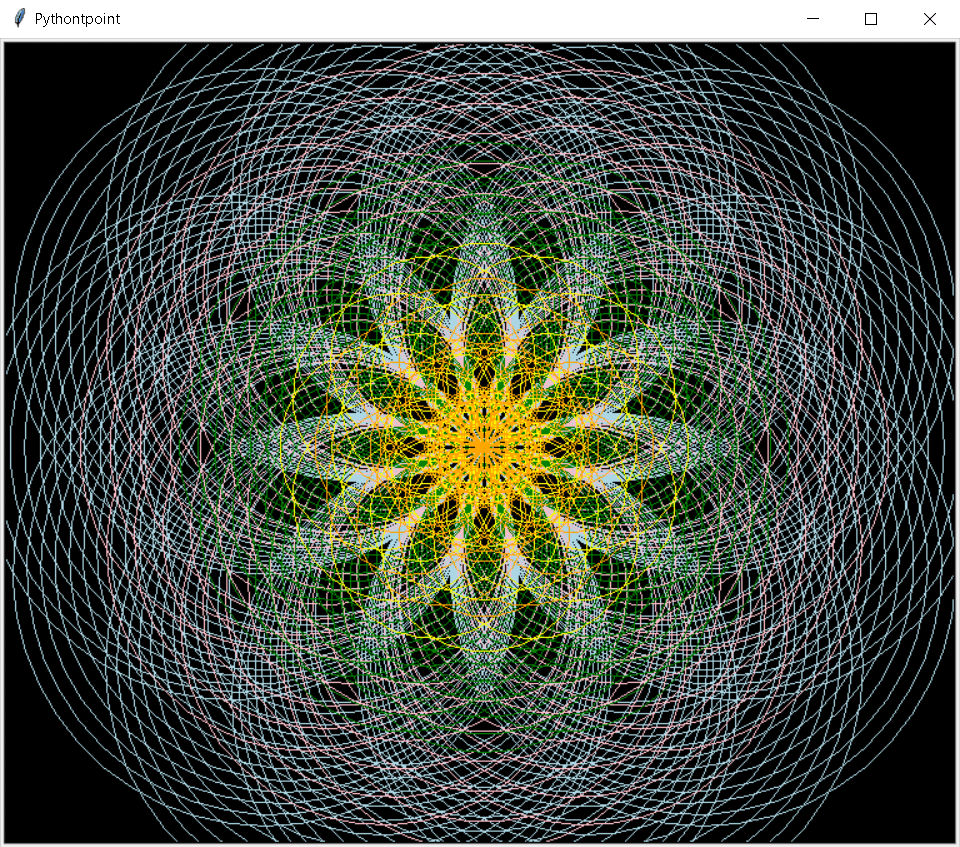
Python Turtle circle
Block of code:
In this block of code, we are importing the turtle module from which we can draw the circle mania.
- ptp = turtle.Screen() is used to create the screen.
- ptp.bgcolor(‘black’) is used to five the black background color to the screen.
- ws = turtle.Turtle() is used to make the objects.
- ws.speed(‘fastest’) is used to increase the speed of the turtle.
- ws.color(‘light blue’) is used to give the light blue color to the turtle.
from turtle import *
import turtle
ptp = turtle.Screen()
ptp.bgcolor('black')
ws = turtle.Turtle()
ws.speed('fastest')
ws.color('light blue')
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of code:
In this block of code, we are splitting the code and start creating the new circle with the help of different colors.
- tur.circle(siz) is used to draw the circle.
- circlemania(tur,siz) is used to create circle mania.
- tur.right(360/repet) is used to move the turtle in the right direction.
- tur1 = turtle.Turtle() is used to make the objects.
- tur1.speed(0) is used to change the turtle speed.
- tur1.color(‘pink’) is used to give the pink color to the turtle.
def circlemania(tur,siz):
for i in range(12):
tur.circle(siz)
siz=siz-6
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(ws,202,12)
tur1 = turtle.Turtle()
tur1.speed(0)
tur1.color('pink')
Read:
Block of code:
In this block of code, we are start to draw the new circle with the help of a green color turtle.
- tur.circle(siz) is used to draw the circle on the screen.
- tur.right(360/repet) is used to move the turtle in the right direction.
- tur2= turtle.Turtle() is used to make the objects.
- tur2.color(‘green’) is used to give the green color to the turtle.
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-12
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur1,162,12)
tur2= turtle.Turtle()
tur2.speed(0)
tur2.color('green')
Block of code:
In this block of code, we are drawing the new circle to form a circle mania.
- tur.circle(siz) is used to draw the circle.
- tur.right(360/repet) is used to move the turtle in the right direction.
- tur3 = turtle.Turtle() is used to make the objects.
- tur3.color(‘yellow’) is used to give the yellow color to the turtle.
rotate=int(80)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-7
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur2,122,12)
tur3 = turtle.Turtle()
tur3.speed(0)
tur3.color('yellow')
Block of code:
In this block of code, we are splitting the code are draw the new circle for completing the circle mania.
- tur.circle(siz) is used to draw the circle.
- tur.right(360/repet) is used to move the turtle in the right direction.
- tur4= turtle.Turtle() is used to make the objects.
- tur4.color(‘orange’) is used to give the orange color to the turtle.
rotate=int(90)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-21
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur3,82,12)
tur4= turtle.Turtle()
tur4.speed(0)
tur4.color('orange')
Block of code:
In this block of code, we draw the new circle from which we can create the circle mania.
- tur.circle(siz) is used to draw the circle.
- tur.right(360/repet) is used to move the turtle in the right direction.
rotate=int(90)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-22
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur4,42,12)
Code:
Hereafter splitting the whole code and explaining how we can make a circle mania using python turtle now we see what the output looks like after running the whole code.
from turtle import *
import turtle
ptp = turtle.Screen()
ptp.bgcolor('black')
ws = turtle.Turtle()
ws.speed('fastest')
ws.color('light blue')
rotate=int(180)
def circlemania(tur,siz):
for i in range(12):
tur.circle(siz)
siz=siz-6
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(ws,202,12)
tur1 = turtle.Turtle()
tur1.speed(0)
tur1.color('pink')
rotate=int(90)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-12
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur1,162,12)
tur2= turtle.Turtle()
tur2.speed(0)
tur2.color('green')
rotate=int(80)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-7
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur2,122,12)
tur3 = turtle.Turtle()
tur3.speed(0)
tur3.color('yellow')
rotate=int(90)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-21
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur3,82,12)
tur4= turtle.Turtle()
tur4.speed(0)
tur4.color('orange')
rotate=int(90)
def circlemania(tur,siz):
for x in range(6):
tur.circle(siz)
siz=siz-22
def ptp1(tur,siz,repet):
for x in range (repet):
circlemania(tur,siz)
tur.right(360/repet)
ptp1(tur4,42,12)
Output:
After running the whole code we get the following output in which we can see that the beautiful python turtle circle mania is drawn on the screen.
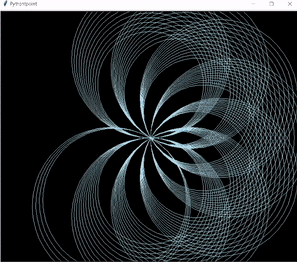

top online pharmacy