In this Python Tkinter tutorial, we will learn about the Python Tkinter temperature converter. In this, there is an interface in which the user can give input the temperature in celsius, and it displays it in Fahrenheit and vice versa.
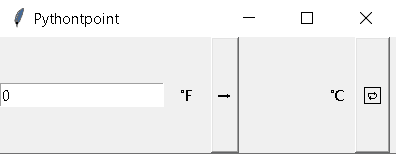
Python Tkinter temperature converter
In this project, we see that there is an interface in which the user can give input the temperature in celsius, and it displays it in Fahrenheit and vice versa.
Block of code:
In the following Python Tkinter block of code, we will import the Tkinter module from which we can convert the temperature from Celsius to Fahrenheit.
from tkinter import *
import tkinter as tk
import ctypes
ctypes.windll.shcore.SetProcessDpiAwareness(1)
Block of code:
In the following Python Tkinter block of code, we will define the converted temperature function.
- labelleft[‘text’]==’\N{DEGREE FAHRENHEIT}’: is used to give the left label.
- tempf = entrytemp.get() is used to get the user input.
def converttemperature():
if labelleft['text']=='\N{DEGREE FAHRENHEIT}':
tempf = entrytemp.get()
tempc= (float(tempf)-32)*5/9
labeltemp['text']=f"{round(tempc, 2)}"
else:
tempc = entrytemp.get()
tempf= (float(tempc)*9/5)+32
labeltemp['text']=f"{round(tempf, 2)}"
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of code:
In the following Python Tkinter block of code, we define the reverse function from which we can reverse Fahrenheit to Celcius.
def reverse():
if labelleft['text']=='\N{DEGREE FAHRENHEIT}':
labelleft['text']="\N{DEGREE CELSIUS}"
labelright['text']='\N{DEGREE FAHRENHEIT}'
else:
labelleft['text']='\N{DEGREE FAHRENHEIT}'
labelright['text']='\N{DEGREE CELSIUS}'
Block of code:
In the following Python Tkinter block of code, we create a Tkinter window from which we can create a temperature converter app.
wd.title(‘Pythontpoint’) is used to give the title to the window.
wd = tk.Tk()
wd.title('Pythontpoint')
Read: Stone Paper Scissor using Python Tkinter
Python Tkinter Simple Calculator
Block of code:
In the following Python Tkinter block of code, we create an entry box in which the user can enter their input.
- entrytemp = tk.Entry(master = wd) is used to create a entry box.
- entrytemp.insert(0,’0′) is used to insert the value inside the entry box.
entrytemp = tk.Entry(master = wd)
entrytemp.pack(side=tk.LEFT)
entrytemp.insert(0,'0')
Block of code:
In the following Python Tkinter block of code, we create the left label and give the text to the label as Degree Fahrenheit from which we can easily understand the value inside the entry box is in Fahrenheit.
labelleft = tk.Label(text='\N{DEGREE FAHRENHEIT}', width=5)
labelleft.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
Block of code:
In the following Python Tkinter block of code, we create a button from which w call the command which we give to it.
button = tk.Button(text="\N{RIGHTWARDS BLACK ARROW}", command=converttemperature)
button.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
Block of code:
In the following Python Tkinter block of code, we create a reverse button from which we can reverse the degree by simply clicking on the button.
button_reverse = tk.Button(text='\N{CLOCKWISE RIGHTWARDS AND LEFTWARDS OPEN CIRCLE ARROWS}', command=reverse)
button_reverse.pack(side=tk.LEFT,fill=tk.BOTH, padx=6, expand=True)
Code:
In the following Python Tkinter block of code, we will import some Tkinter modules from which we can convert the temperature.
from tkinter import *
import tkinter as tk
import ctypes
ctypes.windll.shcore.SetProcessDpiAwareness(1)
def converttemperature():
if labelleft['text']=='\N{DEGREE FAHRENHEIT}':
tempf = entrytemp.get()
tempc= (float(tempf)-32)*5/9
labeltemp['text']=f"{round(tempc, 2)}"
else:
tempc = entrytemp.get()
tempf= (float(tempc)*9/5)+32
labeltemp['text']=f"{round(tempf, 2)}"
def reverse():
if labelleft['text']=='\N{DEGREE FAHRENHEIT}':
labelleft['text']="\N{DEGREE CELSIUS}"
labelright['text']='\N{DEGREE FAHRENHEIT}'
else:
labelleft['text']='\N{DEGREE FAHRENHEIT}'
labelright['text']='\N{DEGREE CELSIUS}'
wd = tk.Tk()
wd.title('Pythontpoint')
entrytemp = tk.Entry(master = wd)
entrytemp.pack(side=tk.LEFT)
entrytemp.insert(0,'0')
labelleft = tk.Label(text='\N{DEGREE FAHRENHEIT}', width=5)
labelleft.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
button = tk.Button(text="\N{RIGHTWARDS BLACK ARROW}", command=converttemperature)
button.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
labeltemp = tk.Label(text='', width=10)
labeltemp.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
labelright = tk.Label(text="\N{DEGREE CELSIUS}")
labelright.pack(side=tk.LEFT,fill=tk.BOTH, expand=True)
button_reverse = tk.Button(text='\N{CLOCKWISE RIGHTWARDS AND LEFTWARDS OPEN CIRCLE ARROWS}', command=reverse)
button_reverse.pack(side=tk.LEFT,fill=tk.BOTH, padx=6, expand=True)
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that the Python Tkinter temperature converter app is shown on the screen. And we can see that the value is entered in the entry box in Fahrenheit and after pressing the button the value is changed to celsius.
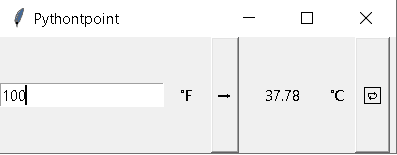
So, in this Python Tkinter tutorial, we have illustrated how to make Python Tkinter Temperature Converter. Moreover, we have also discussed the whole code used in this tutorial.
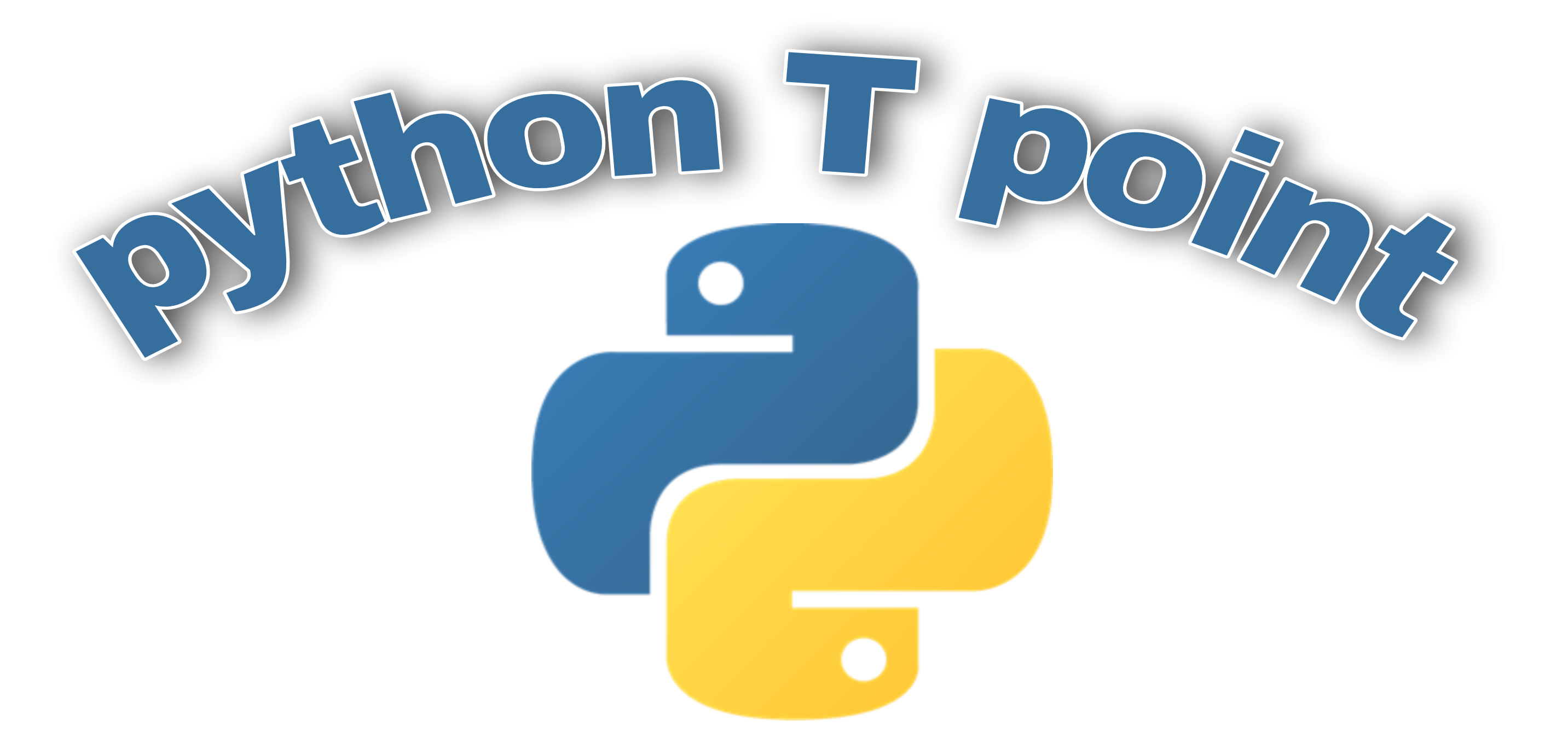
2 thoughts on “Python Tkinter temperature converter”
Comments are closed.