In this tutorial, we will learn about Python GUI programming and we will cover different examples related to Python GUI. And, we will cover these topics.
- How to Download & Install Tkinter in python
- What are widgets in Python Tkinter
- What are the Geometry Managements in Python Tkinter
- How to create our first simple project in Python Tkinter
How to Download & Install Tkinter in python
In this section, we will learn about how to download or install Tkinter in Python. Are you a Python developer and you want to develop an app that supports Graphical User Interfaces.
Here we will learn how to create a Graphical User Interface using Python Tkinter. The work Tkinter as we pronounced as “T-kin-ter”.
If you know how to install Python, then you check the Python installation and download steps.
If you don’t know how to install and the window does not appear then follow the following steps for installation.
Step1:
Firstly check the version of python installed in the system, check by this command python –version.

Step2:
If you want to download then to python.org and download the same version of python. I have downloaded the Python 10 of 64 bit.
Step3:
After clicking on the Python.org URL we directly come to the page where we want to install our python latest version 3.10.0.
Click on the installation file after clicking on the installation window will appear after that click on Install Now option.

Step4:
After following the above step our setup was successfully completed and we are ready to develop the first application on this platform.

Step5:
If we want to check Python is installed or not simply type python -m tkinter on the command prompt. If the below window appeared that means Python is installed.

What are widgets in Python Tkinter
Widgets are used to make our GUI application more interactive. The Graphical User interface is based on the widget. The widget provides us control over which users interacted with our applications.
- Label
- Text boxes
- Buttons
- List boxes
These all are widgets and all these widgets have unique properties.
Labels: Labels are used before while creating an application.
The label is a very common widget that informs about an application or an object.
The label is also defined as a simple piece of information and a text.
Syntax:
Label(master,option..)
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
label =Label(wd , text ="Enter text")
label.pack()
wd.mainloop()
Output:
After running the above we get the following output in which we can see that the value is assign to the label and label is shown on the screen.

Entry:
The entry widget is used for taking the input from the user. Users can enter anything inside the entry box.
This is the second most important widget that is used n Graphical User Interface.
Syntax:
Entry(master)
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
entry = Entry(wd)
entry.pack()
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that an empty text box where Pythontpoint is written by the user.

Button:
The button is used to move the user to the next page it acts as a trigger, Button takes all commands as an argument and completes the target.
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
def click():
return Label(wd,text ="Hello Pythontpoint Users").pack()
click_meButton = Button(wd,text="Click Me",command=click)
click_meButton.pack()
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that another label is created on clicking on the button and another label is shown just below the button.

Message Box:
- Message Box works as a pop-up box that pops up the message on the screen.
- For using the message box just import the message box module.
There are six types of message prompt:
- showinfo: showinfo is used to show the message on the screen with sound and return Ok.
- showwarning: showwarning is used to show the warning on the screen with sound and return Ok.
- showerror: showerror is used to show the error message on the screen with sound and return Ok.
- askquestion: askquestion return yes or no option and also return yes or no.
- askyesorno: askyesorno return yes or no option.Here yes return 1 and no retirn 0.
- askretrycancel:askretrycancel it return the option retry or cancel.Here return returns 1 and cancel returns 0.
Syntax:
mwssagebox.function(title,message)
Code:
In the following code, we can run all the messages under one function that can be run after clicking on the single button.
from tkinter import *
from tkinter import messagebox
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
def messageinfo():
messagebox.showinfo("showinfo", "Hey Pythontpoint User")
messagebox.showwarning("showwarning", "Hey Pythontpoint User")
messagebox.showerror("showerror", "Hey Pythontpoint User")
messagebox.askquestion("askquestion", "Hey Pythontpoint User")
messagebox.askyesno("askyesno", "Hey Pythontpoint User")
messagebox.askretrycancel("askretrycancel", "Hey Pythontpoint User")
Button(wd, text="Click Me!", command=messageinfo).pack()
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that when the click Me! button is pressed all the message boxes appear in the sequence.







What are the Geometry Managements in Python Tkinter
There are Three types of Geometry Management in Python:
- Pack
- Grid
- Place
Pack:
- The pack is a manager of geometry.
- It fill all the space from the center.
- It is very useful for small applications.
- Pack does not require any arguments.
Syntax:
widget.pack()
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
from tkinter import *
Label(wd, text="Place Any").pack()
Label(wd, text="Text ").pack()
Label(wd, text="using ").pack()
Label(wd, text="Pack is Just a Fun").pack()
wd.mainloop()
After running the above code we get the following output in which we can see that the pack place itself in the center of the screen.

Grid:
- Grid store all the data in a tabular form.
- Grid need two arguments rows and columns.
- Here is the rows and columns.

Here we can see the grid in which data is stored in the tabular form the standing part of grid is called row and the slanting part is called column.
Syntax:
widget.grid(row= value,column= value)
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
Button(wd, text="These all buttons").grid(row=0, column=1)
Button(wd, text="are positioned").grid(row=0, column=2)
Button(wd, text="by Grid").grid(row=0, column=3)
Button(wd, text="This is ").grid(row=2, column=1)
Button(wd, text="another line").grid(row=2, column=2)
Button(wd, text="using Grid").grid(row=2, column=3)
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that these all buttons are display with the help of grid.

Place:
- It is used to fixed the position of widget.
- It provides control to the user.
- x and y plue are provided with the anchor tag.
- There are 8 types of anchor tags:
- E :East
- W:West
- N:North
- S:South
- NE:North-East
- NW:North-West
- SE:South-East
- SW:South-West
Syntax:
widget.place(x = value, y = value, anchor = location )
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
Button(wd, text="North-West").place(x=70, y=40, anchor="center")
Button(wd, text="North").place(x=150, y=40, anchor="center")
Button(wd, text="North-South").place(x=270, y=40, anchor="center")
Button(wd, text="East").place(x=70, y=150, anchor="center")
Button(wd, text="West").place(x=250, y=148, anchor="center")
Button(wd, text="Center").place(x=148, y=148, anchor="center")
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that the six button is placed on the screen.

How to create our first simple project in Python Tkinter
Let us understand more about Python GUI programming and creating our first program in python tkinter.
Code:
from tkinter import *
wd = Tk()
wd.title("Pythontpoint")
wd.geometry("400x200")
wd.configure(bg="black")
def welc():
name = nameTf.get()
return Label(wd, text=f'Welome {name}', pady=15, bg='cyan').grid(row=2, columnspan=2)
nameLabel = Label(wd, text="Enter Your Name", pady=15, padx=10, bg='cyan')
nameTf = Entry(wd)
welButton = Button(wd, text="ClickMe!", command=welc)
nameLabel.grid(row=0, column=0)
nameTf.grid(row=0, column=1)
welButton.grid(row=1, columnspan=2)
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that a label,entry box and button is placed on the screen.In entry box user can enter their name in this box and there name is shown below the button after clicking on them.

So, in this tutorial, we discussed Python GUI Programming and we have also covered different examples related to its implementation. Here is the list of examples that we have covered.
- How to Download & Install Tkinter in python
- What are widgets in Python Tkinter
- What are the Geometry Managements in Python Tkinter
- How to create our first simple project in Python Tkinter
Do follow the following tutorials also:
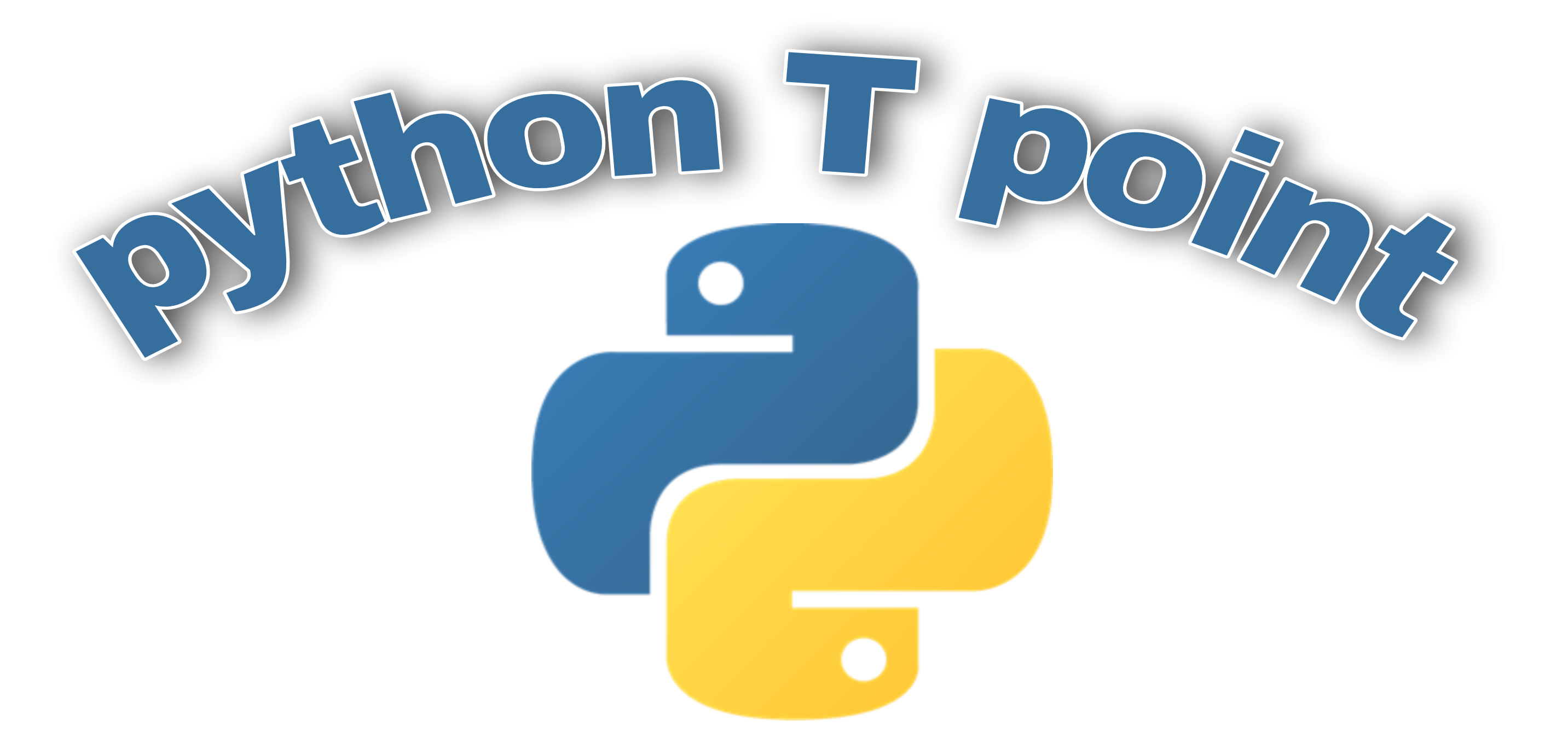