In this tutorial, we will learn How to draw Netflix Logo in Python turtle and we will also cover the different examples related to the Python turtle. Besides this, we will also cover the whole code related to How to create Netflix Logo in Python turtle.
How to Draw Netflix Logo in Python Turtle
In this section we will learn how to draw netflix logo in Python Turtle. The netflix logo has black square background and the red color N is placed inside the background.
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of Code:
In this block of code, we will import the turtle library as import turtle and also import sleep from time.
# How to Draw Netflix Logo in Python Turtle
# Importing library
import turtle
from time import sleep
Block of Code:
Here we are initializing the module and we are giving the speed to the turtle and after that give the white background to the screen and then give the title to the window.
# Initialize the module
tur = turtle.Turtle()
tur.speed(4)
turtle.bgcolor("white")
tur.color("white")
turtle.title('PythonTpoint')
Block of Code:
Here we are drawing the black square background inside which we can draw our netflix logo and the n logo is of red color.
- tur.fillcolor(“black”) is used to fill the black color for background.
- tur.forward(200) is used to move the turtle in the forward direction.
- tur.goto(-80,50) is used to move the turtle in the x and y position.
# Drawing the black background
tur.up()
tur.goto(-80,50)
tur.down()
tur.fillcolor("black")
tur.begin_fill()
tur.forward(200)
tur.setheading(270)
t = 360
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(180)
t = 270
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(200)
t = 180
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(180)
t = 90
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(30)
tur.end_fill()
Block of Code:
Here we are drawing the N shape logo of the netflix on the screen with the help of the turtle and here pen is work as a turtle.
- tur.color(“black”) is used to give the black color to the turtle.
- tur.forward(240) is used to move the turtle in the forward direction.
- tur.down() is used to move the turtle in the down direction.
- tur.begin_fill() is used to start filling color inside the shape.
- tur.end_fill() is used to stop the end filling color.
# Drawing the N shape
tur.up()
tur.color("black")
tur.setheading(270)
tur.forward(240)
tur.setheading(0)
tur.down()
tur.color("red")
tur.fillcolor("#E50914")
tur.begin_fill()
tur.forward(30)
tur.setheading(90)
tur.forward(180)
tur.setheading(180)
tur.forward(30)
tur.setheading(270)
tur.forward(180)
tur.end_fill()
tur.setheading(0)
tur.up()
tur.forward(75)
tur.down()
tur.color("red")
tur.fillcolor("#E50914")
tur.begin_fill()
tur.forward(30)
tur.setheading(90)
tur.forward(180)
tur.setheading(180)
tur.forward(30)
tur.setheading(270)
tur.forward(180)
tur.end_fill()
tur.color("red")
tur.fillcolor("red")
tur.begin_fill()
tur.setheading(113)
tur.forward(195)
tur.setheading(0)
tur.forward(31)
tur.setheading(293)
tur.forward(196)
tur.end_fill()
tur.hideturtle()
sleep(10)
Code:
Hereafter splitting the code and explaining how to create netflix logo in Python Turtle, now we will see how the output look like after running the whole code.
# How to Draw Netflix Logo in Python Turtle
# How to Draw Netflix Logo in Python Turtle
# Importing library
import turtle
from time import sleep
# Initialize the module
tur = turtle.Turtle()
tur.speed(4)
turtle.bgcolor("white")
tur.color("white")
turtle.title('PythonTpoint')
# Drawing the black background
tur.up()
tur.goto(-80,50)
tur.down()
tur.fillcolor("black")
tur.begin_fill()
tur.forward(200)
tur.setheading(270)
t = 360
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(180)
t = 270
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(200)
t = 180
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(180)
t = 90
for x in range(9):
t = t - 10
tur.setheading(t)
tur.forward(10)
tur.forward(30)
tur.end_fill()
# Drawing the N shape
tur.up()
tur.color("black")
tur.setheading(270)
tur.forward(240)
tur.setheading(0)
tur.down()
tur.color("red")
tur.fillcolor("#E50914")
tur.begin_fill()
tur.forward(30)
tur.setheading(90)
tur.forward(180)
tur.setheading(180)
tur.forward(30)
tur.setheading(270)
tur.forward(180)
tur.end_fill()
tur.setheading(0)
tur.up()
tur.forward(75)
tur.down()
tur.color("red")
tur.fillcolor("#E50914")
tur.begin_fill()
tur.forward(30)
tur.setheading(90)
tur.forward(180)
tur.setheading(180)
tur.forward(30)
tur.setheading(270)
tur.forward(180)
tur.end_fill()
tur.color("red")
tur.fillcolor("red")
tur.begin_fill()
tur.setheading(113)
tur.forward(195)
tur.setheading(0)
tur.forward(31)
tur.setheading(293)
tur.forward(196)
tur.end_fill()
tur.hideturtle()
sleep(10)
Output:
After running the whole code we get the following output in which we can see that the netflix logo is drawn on the screen with the help of Python Turtle on the screen.
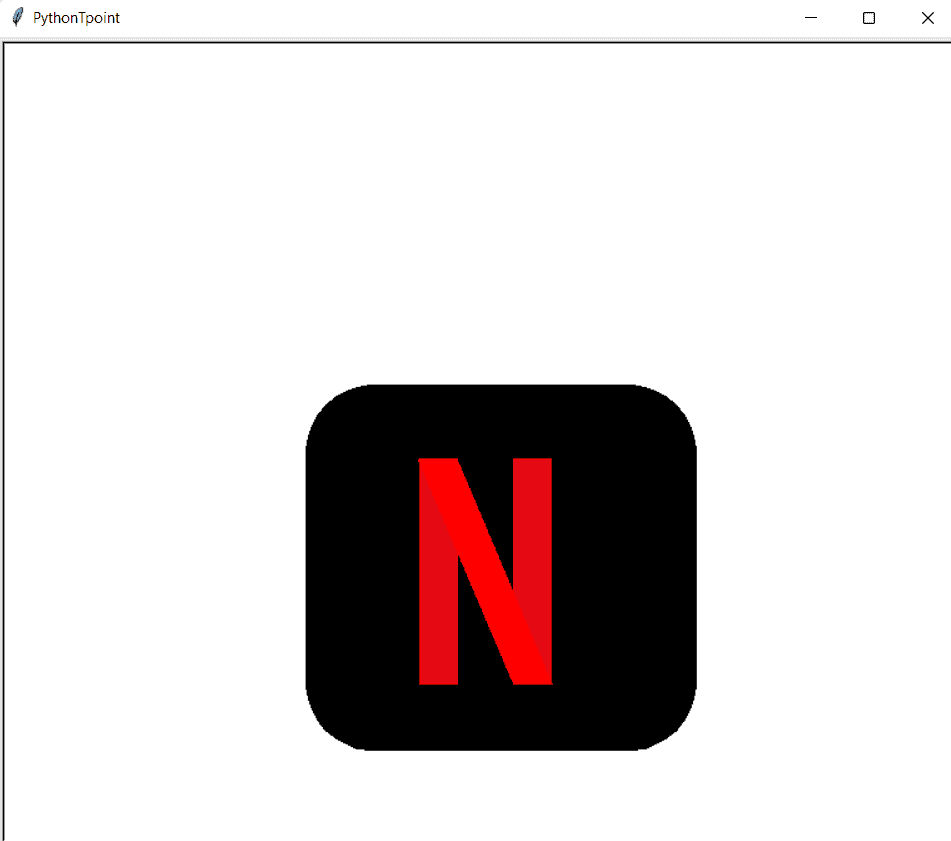
So, in this tutorial, we have illustrated How to draw Netflix Logo in Python Turtle. Moreover, we have also discussed the whole code used in this tutorial.
Read some more tutorials related to Python Turtle.
- Python Turtle Snake Game
- Python Turtle Chess Game
- How to Make a Dog Face Using Python Turtle
- How to Write Happy Birthday using Python Turtle
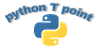
It was impossible for me to leave your website without expressing my gratitude for the excellent knowledge you give your visitors. Without a doubt, I’ll be checking back frequently to see what updates you’ve made.
Hello, i feel that i saw you visited my web site thus i got here to “go back the desire”.I’m trying to to find things to improve my web site!I guess its ok to make use of some of your ideas!!
mexican pharmaceuticals online: cmq pharma mexican pharmacy – medicine in mexico pharmacies
buying prescription drugs in mexico
http://cmqpharma.com/# reputable mexican pharmacies online
medicine in mexico pharmacies
Good post. I be taught one thing more challenging on totally different blogs everyday. It is going to at all times be stimulating to read content material from other writers and follow slightly something from their store. I’d choose to make use of some with the content material on my weblog whether or not you don’t mind. Natually I’ll provide you with a link on your web blog. Thanks for sharing.
india pharmacy mail order: world pharmacy india – online pharmacy india
mexican pharmacy: mexican online pharmacies prescription drugs – mexican pharmacy
https://canadapharmast.online/# canadian pharmacy tampa
mexican mail order pharmacies: medication from mexico pharmacy – buying prescription drugs in mexico
https://canadapharmast.com/# pharmacy canadian superstore
world pharmacy india online shopping pharmacy india pharmacy website india
canadian pharmacies comparison canadian pharmacy 365 buy prescription drugs from canada cheap
best online pharmacies in mexico: mexico pharmacy – reputable mexican pharmacies online
https://canadapharmast.com/# canadian pharmacy meds review
indian pharmacies safe: world pharmacy india – top 10 pharmacies in india
mexican pharmaceuticals online: best online pharmacies in mexico – purple pharmacy mexico price list
canadian pharmacy victoza canadian online pharmacy reviews canadian pharmacy 24h com
best online pharmacies in mexico: mexican drugstore online – medication from mexico pharmacy
pharmacies in mexico that ship to usa: mexico drug stores pharmacies – reputable mexican pharmacies online
best canadian pharmacy online: safe canadian pharmacies – best rated canadian pharmacy
medicine in mexico pharmacies: reputable mexican pharmacies online – mexico pharmacies prescription drugs
http://foruspharma.com/# pharmacies in mexico that ship to usa
mexican pharmacy mexican online pharmacies prescription drugs best online pharmacies in mexico
http://foruspharma.com/# mexico pharmacies prescription drugs
canadian drugs pharmacy canadian pharmacy meds canadian online drugstore
indian pharmacy online: Online medicine order – indian pharmacies safe
order amoxicillin online: amoxicillin 500 mg for sale – amoxicillin medicine over the counter
http://ciprodelivery.pro/# ciprofloxacin 500 mg tablet price
https://clomiddelivery.pro/# where to buy cheap clomid without rx
http://doxycyclinedelivery.pro/# doxycycline generic cost
antibiotics cipro buy cipro online canada cipro
doxycycline 10mg cost: doxycycline tablets online india – doxycycline 50
https://paxloviddelivery.pro/# paxlovid pill
https://ciprodelivery.pro/# ciprofloxacin generic price
https://clomiddelivery.pro/# can i buy cheap clomid tablets
cost cheap clomid pills where buy clomid without dr prescription can i buy clomid without rx
paxlovid pill: paxlovid pill – paxlovid pharmacy
https://clomiddelivery.pro/# how to get cheap clomid pills
https://amoxildelivery.pro/# amoxicillin 500mg capsule
https://clomiddelivery.pro/# can you buy cheap clomid no prescription
doxycycline price comparison where to buy doxycycline doxycycline 200mg price in india
https://doxycyclinedelivery.pro/# doxycycline 100mg tablets for sale
how to get generic clomid price: can you get generic clomid tablets – where buy clomid online
https://amoxildelivery.pro/# amoxicillin discount
https://clomiddelivery.pro/# where buy cheap clomid without rx
where can i get clomid no prescription cost of clomid without dr prescription cheap clomid pills
http://clomiddelivery.pro/# how to buy cheap clomid no prescription
paxlovid buy: Paxlovid over the counter – Paxlovid buy online
https://doxycyclinedelivery.pro/# doxy 100
https://clomiddelivery.pro/# can i get clomid without prescription
https://doxycyclinedelivery.pro/# buy doxycycline over the counter
buying generic clomid without prescription generic clomid without a prescription can i get cheap clomid without prescription
doxycycline price usa: doxycycline tablets for sale – doxycycline online purchase
http://ciprodelivery.pro/# ciprofloxacin
https://ciprodelivery.pro/# ciprofloxacin 500 mg tablet price
https://amoxildelivery.pro/# buy cheap amoxicillin online
Paxlovid over the counter п»їpaxlovid п»їpaxlovid
order doxycycline 100mg: doxycycline 100 mg tablet cost – doxycycline 60 mg
https://clomiddelivery.pro/# how can i get cheap clomid without prescription
http://doxycyclinedelivery.pro/# where can i buy doxycycline without prescription
http://doxycyclinedelivery.pro/# doxycycline 1000mg best buy
buy generic clomid no prescription how can i get generic clomid without dr prescription how can i get clomid for sale
where buy generic clomid prices: can you buy generic clomid for sale – can i order cheap clomid without a prescription
http://amoxildelivery.pro/# how to get amoxicillin over the counter
https://clomiddelivery.pro/# where to get clomid without dr prescription
Temp Mail I truly appreciate your technique of writing a blog. I added it to my bookmark site list and will
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
pharmacy prices for doxycycline: doxycycline 100 mg tablet – odering doxycycline
mexican pharmacy buying from online mexican pharmacy pharmacies in mexico that ship to usa
http://mexicandeliverypharma.com/# reputable mexican pharmacies online
mexican pharmaceuticals online: mexican mail order pharmacies – mexican mail order pharmacies
mexican drugstore online: mexico drug stores pharmacies – medication from mexico pharmacy
mexican border pharmacies shipping to usa pharmacies in mexico that ship to usa mexican rx online
buying prescription drugs in mexico online: mexican drugstore online – buying prescription drugs in mexico online
http://mexicandeliverypharma.com/# buying prescription drugs in mexico online
mexican pharmacy best online pharmacies in mexico reputable mexican pharmacies online
buying prescription drugs in mexico online: mexican border pharmacies shipping to usa – buying prescription drugs in mexico online
https://mexicandeliverypharma.online/# buying prescription drugs in mexico online
reputable mexican pharmacies online: medicine in mexico pharmacies – buying from online mexican pharmacy
medicine in mexico pharmacies mexican pharmaceuticals online medicine in mexico pharmacies
buying prescription drugs in mexico online: mexican rx online – medication from mexico pharmacy
reputable mexican pharmacies online: pharmacies in mexico that ship to usa – buying prescription drugs in mexico online
best online pharmacies in mexico: mexico drug stores pharmacies – reputable mexican pharmacies online
mexico pharmacies prescription drugs mexican pharmacy mexican online pharmacies prescription drugs
buying from online mexican pharmacy: buying prescription drugs in mexico online – medicine in mexico pharmacies
best online pharmacies in mexico: mexico drug stores pharmacies – purple pharmacy mexico price list
mexican mail order pharmacies: mexican online pharmacies prescription drugs – mexican border pharmacies shipping to usa
mexico pharmacies prescription drugs mexican pharmaceuticals online mexican rx online
medicine in mexico pharmacies: mexican border pharmacies shipping to usa – medication from mexico pharmacy
reputable mexican pharmacies online: mexico drug stores pharmacies – best online pharmacies in mexico
reputable mexican pharmacies online п»їbest mexican online pharmacies buying prescription drugs in mexico online
mexican pharmaceuticals online: pharmacies in mexico that ship to usa – medicine in mexico pharmacies
buying prescription drugs in mexico: buying prescription drugs in mexico online – п»їbest mexican online pharmacies
purple pharmacy mexico price list: mexican rx online – purple pharmacy mexico price list
mexican mail order pharmacies mexican drugstore online medicine in mexico pharmacies
mexican mail order pharmacies: mexican rx online – mexico pharmacies prescription drugs
pharmacies in mexico that ship to usa: п»їbest mexican online pharmacies – mexican online pharmacies prescription drugs
Vitazen Keto Good post! We will be linking to this particularly great post on our site. Keep up the great writing
Temp Mail Pretty! This has been a really wonderful post. Many thanks for providing these details.
medicine in mexico pharmacies: reputable mexican pharmacies online – mexican rx online
mexican rx online mexican drugstore online п»їbest mexican online pharmacies
pharmacies in mexico that ship to usa: buying prescription drugs in mexico – pharmacies in mexico that ship to usa
buying from online mexican pharmacy: purple pharmacy mexico price list – medication from mexico pharmacy
buying from online mexican pharmacy: best online pharmacies in mexico – purple pharmacy mexico price list
mexican mail order pharmacies: mexican mail order pharmacies – reputable mexican pharmacies online
mexican online pharmacies prescription drugs: medication from mexico pharmacy – mexico drug stores pharmacies
mexico pharmacies prescription drugs mexico drug stores pharmacies mexican border pharmacies shipping to usa
mexican pharmaceuticals online: mexico pharmacies prescription drugs – mexican online pharmacies prescription drugs
mexican border pharmacies shipping to usa: mexican rx online – best online pharmacies in mexico
mexican border pharmacies shipping to usa: pharmacies in mexico that ship to usa – purple pharmacy mexico price list
mexican online pharmacies prescription drugs medication from mexico pharmacy pharmacies in mexico that ship to usa
mexico drug stores pharmacies: buying prescription drugs in mexico – buying prescription drugs in mexico online
mexico pharmacies prescription drugs: buying prescription drugs in mexico online – buying prescription drugs in mexico online
п»їbest mexican online pharmacies: mexico pharmacies prescription drugs – medication from mexico pharmacy
purple pharmacy mexico price list: mexican drugstore online – medication from mexico pharmacy
purple pharmacy mexico price list mexican mail order pharmacies medicine in mexico pharmacies
mexico drug stores pharmacies: mexican online pharmacies prescription drugs – mexico pharmacies prescription drugs
mexican online pharmacies prescription drugs: buying from online mexican pharmacy – buying from online mexican pharmacy
mexican rx online: mexican border pharmacies shipping to usa – medicine in mexico pharmacies
reputable mexican pharmacies online: mexican rx online – mexican drugstore online
mexico drug stores pharmacies: pharmacies in mexico that ship to usa – pharmacies in mexico that ship to usa
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I have been browsing online more than three hours today yet I never found any interesting article like yours It is pretty worth enough for me In my view if all website owners and bloggers made good content as you did the internet will be a lot more useful than ever before
pharmacies in mexico that ship to usa: buying prescription drugs in mexico – medicine in mexico pharmacies
I was just as enthralled by your work as you were. Your sketch is elegant, and your written content is sophisticated. However, you seem concerned about potentially delivering something questionable soon. I’m confident you’ll resolve this issue quickly and return to your usual high standards.
medicine in mexico pharmacies: best online pharmacies in mexico – buying prescription drugs in mexico
mexican drugstore online: reputable mexican pharmacies online – medication from mexico pharmacy
mexico drug stores pharmacies: п»їbest mexican online pharmacies – mexican border pharmacies shipping to usa
buy cheap propecia without a prescription: cheap propecia price – get generic propecia without dr prescription
http://cytotecbestprice.pro/# order cytotec online
https://prednisonebestprice.pro/# 200 mg prednisone daily
buy cytotec: buy cytotec over the counter – cytotec online
https://cytotecbestprice.pro/# Misoprostol 200 mg buy online
https://zithromaxbestprice.pro/# zithromax for sale 500 mg
cytotec pills buy online: buy cytotec over the counter – п»їcytotec pills online
https://cytotecbestprice.pro/# cytotec online
http://zithromaxbestprice.pro/# zithromax 500 mg
order cheap propecia no prescription: how cЙ‘n i get cheap propecia pills – buy cheap propecia tablets
I loved as much as you will receive carried out right here The sketch is tasteful your authored subject matter stylish nonetheless you command get got an edginess over that you wish be delivering the following unwell unquestionably come further formerly again as exactly the same nearly very often inside case you shield this hike
Misoprostol 200 mg buy online: buy misoprostol over the counter – buy cytotec over the counter
cost of cheap propecia online: buy propecia pills – propecia pill
prednisone tablets canada: by prednisone w not prescription – prednisone 5mg capsules
certainly like your website but you need to take a look at the spelling on quite a few of your posts Many of them are rife with spelling problems and I find it very troublesome to inform the reality nevertheless I will definitely come back again
propecia online: buy propecia without dr prescription – generic propecia without a prescription
https://cytotecbestprice.pro/# buy cytotec in usa
prednisone 50 mg tablet canada: prednisone 40 mg daily – prednisone otc price
order zithromax over the counter: buy zithromax 1000mg online – zithromax 500
comprare farmaci online all’estero: avanafil 100 mg prezzo – farmacie online affidabili
http://farmait.store/# farmacia online
Farmacie online sicure Avanafil compresse top farmacia online
migliori farmacie online 2024: Farmacia online piu conveniente – farmaci senza ricetta elenco
viagra online spedizione gratuita: viagra generico – viagra 50 mg prezzo in farmacia
top farmacia online: super kamagra – acquisto farmaci con ricetta
viagra 100 mg prezzo in farmacia viagra online in 2 giorni or viagra generico recensioni
https://member.findall.co.kr/stipulation/stipulation.asp?targetpage=http://m.findall.co.kr&basehost=viagragenerico.site gel per erezione in farmacia
alternativa al viagra senza ricetta in farmacia le migliori pillole per l’erezione and viagra online in 2 giorni cialis farmacia senza ricetta
acquisto farmaci con ricetta: Avanafil 50 mg – comprare farmaci online all’estero
farmaci senza ricetta elenco: Tadalafil generico migliore – comprare farmaci online all’estero
cerco viagra a buon prezzo pillole per erezione immediata or viagra subito
https://sandbox.google.com/url?q=https://viagragenerico.site viagra prezzo farmacia 2023
siti sicuri per comprare viagra online viagra 50 mg prezzo in farmacia and viagra naturale in farmacia senza ricetta viagra online spedizione gratuita
acquisto farmaci con ricetta: Cialis generico 20 mg 8 compresse prezzo – Farmacie online sicure
cialis soft tabs: Generic Tadalafil 20mg price – cialis shipping
canadian pharmacy online cialis: Buy Tadalafil 20mg – cialis 5mg price
https://sildenafil.llc/# viagra generic
costa rica cialis sale Generic Cialis without a doctor prescription cialis store in qatar
https://tadalafil.auction/# cialis vs viagra canadian pharmacy
use cialis promise coupon orlando fl: Generic Tadalafil 20mg price – cialis without prescription overnight
cialis one a day with dapoxetine canada: Generic Tadalafil 20mg price – cialis online mastercard
http://sildenafil.llc/# 100mg viagra without a doctor prescription
cialis 200 mg price cialis without a doctor prescription super cialis best price
buy cialis with paypal: Buy Cialis online – cialis canada org doc
viagra online buy viagra professional or viagra without a doctor prescription usa
https://images.google.tk/url?sa=t&url=https://sildenafil.llc blue pill viagra
100 mg viagra lowest price viagra cost and generic viagra 100mg buy viagra generic
how much is a cialis prescription with no insurance cheapest cialis in australia or free trial cialis
https://cse.google.cd/url?q=https://tadalafil.auction viagra cialis
when will generic cialis be available in the usa cialis 20mg online and viagra and cialis generic cialis 20 mg
buy generic viagra online: buy sildenafil online canada – free viagra
https://sildenafil.llc/# viagra canada
how does viagra work Viagra online price cheap viagra
https://sildenafil.llc/# buy generic viagra online
viagra side effects: Buy Viagra online in USA – generic viagra overnight
п»їover the counter viagra: Cheap Viagra online – buy viagra online without a prescription
http://sildenafil.llc/# ed pills that work better than viagra
viagra coupon Buy Viagra online cheap viagra without a doctor prescription
http://edpillpharmacy.store/# pills for ed online
erectile dysfunction meds online
http://mexicopharmacy.win/# mexican pharmaceuticals online
mexico drug stores pharmacies: Best online Mexican pharmacy – best online pharmacies in mexico
https://edpillpharmacy.store/# low cost ed meds online
get ed meds today
erectile dysfunction online: online ed prescription same-day – cheap ed pills
https://indiapharmacy.shop/# online pharmacy india
india online pharmacy Best Indian pharmacy top 10 pharmacies in india
http://indiapharmacy.shop/# Online medicine order
cheapest erectile dysfunction pills
mexican online pharmacies prescription drugs: buying prescription drugs in mexico – mexican mail order pharmacies
http://mexicopharmacy.win/# mexican rx online
mexican border pharmacies shipping to usa: Medicines Mexico – п»їbest mexican online pharmacies
erectile dysfunction medications online ed medications online or buy erectile dysfunction treatment
https://www.manacomputers.com/redirect.php?blog=B8B2B8%99B884B8%ADB89EB8%B4B880B8%95B8A3B9%8C&url=http://edpillpharmacy.store%20 edmeds
low cost ed pills ed pills cheap and best ed pills online buy ed meds online
indian pharmacy online top 10 pharmacies in india or reputable indian pharmacies
https://www.google.ws/url?q=https://indiapharmacy.shop::: india pharmacy
buy prescription drugs from india reputable indian pharmacies and mail order pharmacy india Online medicine home delivery
mexico drug stores pharmacies: Certified Mexican pharmacy – buying from online mexican pharmacy
https://edpillpharmacy.store/# erectile dysfunction medications online
mexican rx online Mexico pharmacy online buying from online mexican pharmacy
http://mexicopharmacy.win/# mexico drug stores pharmacies
cheap boner pills where can i buy erectile dysfunction pills or buy ed pills
http://letssearch.com/?domainname=edpillpharmacy.store online erectile dysfunction medication
erection pills online cheapest ed pills and online ed meds best online ed pills
https://mexicopharmacy.win/# buying prescription drugs in mexico online
buying prescription drugs in mexico online: Purple pharmacy online ordering – mexican pharmacy
http://mexicopharmacy.win/# best online pharmacies in mexico
online shopping pharmacy india Online pharmacy indian pharmacy paypal
online shopping pharmacy india indian pharmacy online or mail order pharmacy india
https://www.google.ms/url?sa=t&url=https://indiapharmacy.shop india online pharmacy
online shopping pharmacy india cheapest online pharmacy india and top 10 online pharmacy in india indian pharmacy paypal
https://mexicopharmacy.win/# best online pharmacies in mexico
mexico pharmacies prescription drugs: Medicines Mexico – medication from mexico pharmacy
http://mexicopharmacy.win/# buying prescription drugs in mexico online
india pharmacy Top online pharmacy in India indian pharmacy paypal
https://indiapharmacy.shop/# india pharmacy mail order
http://indiapharmacy.shop/# pharmacy website india
http://mexicopharmacy.win/# mexican drugstore online
http://mexicopharmacy.win/# buying prescription drugs in mexico online
buy cytotec online http://cytotec.pro/# cytotec pills buy online
lasix 100mg
https://cytotec.pro/# cytotec pills buy online
cytotec buy online usa https://tamoxifen.bid/# raloxifene vs tamoxifen
lasix furosemide 40 mg
lasix pills lasix medication furosemide 100mg
https://lisinopril.guru/# buy lisinopril 20 mg without prescription
buy cytotec online fast delivery http://lipitor.guru/# lipitor rx cost
generic lasix
Cytotec 200mcg price http://furosemide.win/# lasix furosemide
furosemida
https://lipitor.guru/# lipitor pill
buy generic lipitor canada lipitor 10 mg tablet or best price lipitor
https://images.google.com.ag/url?sa=t&url=https://lipitor.guru lipitor india
lipitor 10mg generic lipitor india generic and lipitor price in india lipitor cost
buy lipitor 40 mg Atorvastatin 20 mg buy online lipitor generic
buy cytotec online: buy misoprostol tablet – buy cytotec online
cytotec online buy cytotec online or buy misoprostol over the counter
https://hc-sparta.cz/media_show.asp?type=1&id=729&url_back=https://cytotec.pro Misoprostol 200 mg buy online
Abortion pills online cytotec pills buy online and Abortion pills online Cytotec 200mcg price
buy misoprostol over the counter https://lisinopril.guru/# lisinopril 5 mg tablet price in india
lasix dosage
lipitor 20 mg where to buy: buy lipitor 20mg – lipitor 20mg price australia
http://tamoxifen.bid/# where to get nolvadex
buying lisinopril online lisinopril medicine or lisinopril 12.5 20 g
http://forvalour.patternhosting.com/english/exit-eng.php?url=https://lisinopril.guru/ lisinopril 40 mg for sale
where to buy lisinopril without prescription lisinopril 500 mg and lisinopril online usa 2 prinivil
lasix furosemide 40 mg: lasix uses – lasix furosemide
lipitor prescription prices price of lipitor 40 mg or cheap lipitor
https://images.google.rw/url?q=https://lipitor.guru lipitor rx
generic lipitor canada pharmacy can i buy lipitor online and lipitor 10mg tablet price lipitor price in india
https://furosemide.win/# lasix furosemide 40 mg
buy cytotec over the counter п»їcytotec pills online or Cytotec 200mcg price
http://dereferer.org/?http://cytotec.pro/ Abortion pills online
buy cytotec over the counter buy cytotec in usa and buy cytotec in usa buy cytotec online fast delivery
ordering lisinopril without a prescription: cheap lisinopril – best price for lisinopril
https://furosemide.win/# lasix
purchase cytotec buy misoprostol tablet buy cytotec
lipitor generic australia: lipitor medicine price – lipitor atorvastatin
tamoxifen depression: buy tamoxifen citrate – tamoxifen dose
cytotec buy online usa https://lisinopril.guru/# lisinopril 2.5 mg tablet
lasix 40 mg
http://furosemide.win/# generic lasix
rx lisinopril 10mg lisinopril without prescription or lisinopril 420 1g
https://www.kralen.com/counter.php?link=https://lisinopril.guru can i buy lisinopril in mexico
lisinopril 15 mg tablets lisinopril pills 2.5 mg and buy prinivil zestril canada
nolvadex 10mg: buy tamoxifen citrate – tamoxifen lawsuit
cytotec abortion pill п»їcytotec pills online or buy cytotec online
https://www.mfkfm.cz/media_show.asp?type=1&id=156&url_back=https://cytotec.pro п»їcytotec pills online
cytotec buy online usa Cytotec 200mcg price and cytotec pills buy online buy cytotec
buy lisinopril 20 mg without prescription: Lisinopril refill online – rx lisinopril 10mg
https://lisinopril.guru/# lisinopril 20mg 37.5mg
nolvadex for sale amazon nolvadex side effects tamoxifen breast cancer
online lisinopril lisinopril 10 mg cost or lisinopril 20 mg purchase
http://referless.com/?http://lisinopril.guru/ lisinopril 20 25 mg
lisinopril 10mg tablet lisinopril 104 and zestril cost 30mg lisinopril
tamoxifen alternatives premenopausal: Purchase Nolvadex Online – tamoxifen rash
https://lipitor.guru/# generic lipitor price
buy cytotec buy misoprostol tablet Misoprostol 200 mg buy online
Ny weekly I like the efforts you have put in this, regards for all the great content.
buy lisinopril online india zestril 40 mg tablet or lisinopril 7.5 mg
https://cse.google.com.ng/url?sa=t&url=https://lisinopril.guru zestril tablet price
generic for zestril prinivil lisinopril and lisinopril 10mg tablet cheap lisinopril
http://mexstarpharma.com/# purple pharmacy mexico price list
https://mexstarpharma.com/# buying prescription drugs in mexico
http://mexstarpharma.com/# buying prescription drugs in mexico
Mygreat learning I like the efforts you have put in this, regards for all the great content.
https://easyrxindia.shop/# indian pharmacies safe
Techno rozen Awesome! Its genuinely remarkable post, I have got much clear idea regarding from this post . Techno rozen
http://easyrxindia.com/# india pharmacy mail order
pharmacy wholesalers canada best canadian online pharmacy reviews or canadian pharmacy scam
https://www.google.pt/url?q=https://easyrxcanada.com precription drugs from canada
best online canadian pharmacy canadian pharmacy scam and canadian compounding pharmacy canadian online drugs
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article. https://www.binance.com/lv/register?ref=B4EPR6J0
п»їbest mexican online pharmacies medication from mexico pharmacy or mexican border pharmacies shipping to usa
http://www.qqfuzhi.com/portal/play.php?url=http://mexstarpharma.com reputable mexican pharmacies online
buying from online mexican pharmacy buying from online mexican pharmacy and buying prescription drugs in mexico mexican drugstore online
http://easyrxcanada.com/# best canadian online pharmacy
http://mexstarpharma.com/# buying prescription drugs in mexico online
Thanks for sharing. I read many of your blog posts, cool, your blog is very good. https://accounts.binance.info/register?ref=P9L9FQKY
http://easyrxindia.com/# reputable indian pharmacies
sweet bonanza demo: pragmatic play sweet bonanza – sweet bonanza indir
https://slotsiteleri.bid/# slot siteleri 2024
bonus veren siteler deneme bonusu bonus veren siteler
slot kumar siteleri: en iyi slot siteleri – en iyi slot siteleri
https://denemebonusuverensiteler.win/# deneme bonusu veren siteler
bonus veren siteler: bahis siteleri – deneme bonusu
deneme bonusu veren siteler: bonus veren siteler – deneme bonusu
https://denemebonusuverensiteler.win/# bahis siteleri
sweet bonanza 100 tl sweet bonanza guncel sweet bonanza hilesi
bonus veren siteler: bahis siteleri – bonus veren siteler
https://denemebonusuverensiteler.win/# deneme bonusu
sweet bonanza 100 tl guncel sweet bonanza or sweet bonanza slot demo
https://images.google.gr/url?sa=t&url=http://sweetbonanza.network sweet bonanza 90 tl
sweet bonanza nas?l oynan?r slot oyunlari and pragmatic play sweet bonanza sweet bonanza free spin demo
sweet bonanza slot: sweet bonanza taktik – sweet bonanza slot demo
https://denemebonusuverensiteler.win/# bahis siteleri
en yeni slot siteleri slot siteleri guvenilir 2024 en iyi slot siteleri
sweet bonanza demo turkce sweet bonanza free spin demo or sweet bonanza demo oyna
http://www.hashiriya.jp/url.cgi?http://sweetbonanza.network/ sweet bonanza taktik
sweet bonanza hilesi sweet bonanza free spin demo and sweet bonanza hilesi sweet bonanza guncel
deneme bonusu veren siteler yasal slot siteleri or bonus veren slot siteleri
https://cse.google.com.bd/url?q=https://slotsiteleri.bid en iyi slot siteler
slot siteleri slot siteleri and 2024 en iyi slot siteleri slot siteleri 2024
slot oyun siteleri: en cok kazandiran slot siteleri – en iyi slot siteler
sweet bonanza indir: sweet bonanza hilesi – sweet bonanza siteleri
bahis siteleri deneme bonusu veren siteler or deneme bonusu veren siteler
http://tsm.ru/bitrix/redirect.php?event1=&event2=&event3=&goto=https://denemebonusuverensiteler.win/ bonus veren siteler
bahis siteleri bahis siteleri and bonus veren siteler bahis siteleri
Your blog is a true hidden gem on the internet. Your thoughtful analysis and engaging writing style set you apart from the crowd. Keep up the excellent work!
BaddieHub Good post! We will be linking to this particularly great post on our site. Keep up the great writing
Simplywall For the reason that the admin of this site is working, no uncertainty very quickly it will be renowned, due to its quality contents.
slot siteleri: en yeni slot siteleri – deneme veren slot siteleri
sweet bonanza yasal site: sweet bonanza giris – sweet bonanza free spin demo
http://slotsiteleri.bid/# bonus veren casino slot siteleri
deneme bonusu bahis siteleri bonus veren siteler
sweet bonanza slot: sweet bonanza indir – sweet bonanza demo
https://sweetbonanza.network/# sweet bonanza guncel
http://denemebonusuverensiteler.win/# bahis siteleri
sweet bonanza yasal site guncel sweet bonanza or sweet bonanza kazanma saatleri
http://www.seb-kreuzburg.de/url?q=https://sweetbonanza.network sweet bonanza free spin demo
sweet bonanza bahis sweet bonanza demo and sweet bonanza demo oyna sweet bonanza giris
sweet bonanza siteleri sweet bonanza demo oyna or sweet bonanza free spin demo
https://www.abcplus.biz/cartform.aspx?returnurl=http://sweetbonanza.network sweet bonanza slot demo
sweet bonanza demo oyna sweet bonanza slot and sweet bonanza yasal site sweet bonanza guncel
sweet bonanza giris: sweet bonanza yasal site – sweet bonanza oyna
https://slotsiteleri.bid/# guvenilir slot siteleri
sweet bonanza indir pragmatic play sweet bonanza sweet bonanza demo turkce
deneme bonusu deneme bonusu veren siteler or bonus veren siteler
https://images.google.bf/url?sa=t&url=http://denemebonusuverensiteler.win bonus veren siteler
bahis siteleri bonus veren siteler and bahis siteleri bahis siteleri
sweet bonanza mostbet: pragmatic play sweet bonanza – sweet bonanza
https://denemebonusuverensiteler.win/# bonus veren siteler
en iyi slot siteleri 2024: slot siteleri 2024 – en iyi slot siteler
sweet bonanza free spin demo: sweet bonanza siteleri – sweet bonanza 100 tl
http://slotsiteleri.bid/# yeni slot siteleri
bahis siteleri bahis siteleri deneme bonusu veren siteler
bonus veren siteler: deneme bonusu – bonus veren siteler
https://denemebonusuverensiteler.win/# bahis siteleri
http://denemebonusuverensiteler.win/# deneme bonusu veren siteler
bonus veren casino slot siteleri: en iyi slot siteleri – guvenilir slot siteleri
en yeni slot siteleri: guvenilir slot siteleri – yeni slot siteleri
http://denemebonusuverensiteler.win/# bahis siteleri
deneme bonusu bonus veren siteler or deneme bonusu veren siteler
http://toolbarqueries.google.nl/url?q=https://denemebonusuverensiteler.win bonus veren siteler
deneme bonusu deneme bonusu and bahis siteleri deneme bonusu veren siteler
sweet bonanza 90 tl sweet bonanza slot or slot oyunlari
http://www.mameli.com/gb/show.php?q39fae=sweetbonanza.network sweet bonanza hilesi
sweet bonanza demo sweet bonanza free spin demo and sweet bonanza siteleri sweet bonanza indir
http://slotsiteleri.bid/# bonus veren casino slot siteleri
deneme bonusu: deneme bonusu veren siteler – deneme bonusu veren siteler
http://denemebonusuverensiteler.win/# deneme bonusu veren siteler
slot siteleri: oyun siteleri slot – slot oyunlar? siteleri
slot siteleri bonus veren: deneme bonusu veren siteler – deneme bonusu veren siteler
http://sweetbonanza.network/# sweet bonanza giris
https://sweetbonanza.network/# sweet bonanza yasal site
slot oyunlari: sweet bonanza hilesi – sweet bonanza mostbet
http://sweetbonanza.network/# sweet bonanza free spin demo
пинап казино: пин ап зеркало – pin up казино
https://1xbet.contact/# 1xbet официальный сайт
1хбет: 1хбет зеркало – 1xbet скачать
казино вавада vavada online casino vavada online casino
pin up казино: пинап казино – пин ап
vavada online casino vavada casino or казино вавада
https://www.google.com.np/url?q=https://vavada.auction vavada casino
vavada зеркало vavada online casino and вавада рабочее зеркало vavada
1вин 1вин зеркало or 1вин
https://www.google.com.bz/url?sa=t&url=https://1win.directory 1win вход
1вин 1вин зеркало and 1вин официальный сайт 1вин сайт
1win официальный сайт: 1вин зеркало – 1win вход
http://pin-up.diy/# pin up casino
пин ап вход: pin up casino – pin up casino
казино вавада вавада or вавада рабочее зеркало
https://images.google.as/url?sa=t&url=https://vavada.auction vavada online casino
vavada казино вавада казино and вавада vavada casino
1вин 1win зеркало or 1win зеркало
http://www.protvino.ru/bitrix/rk.php?id=20&event1=banner&event2=click&goto=http://1win.directory 1win вход
1вин сайт 1win and 1win 1вин сайт
http://vavada.auction/# vavada online casino
казино вавада: vavada казино – vavada зеркало
pharmacy store design layout: fred meyer pharmacy hours – viagra pharmacy bangkok
https://easydrugrx.com/# schnucks pharmacy
generic lexapro online pharmacy trimix online pharmacy pharmacy generic viagra
online pharmacy no prescription augmentin: pharmacy grade nolvadex – terbinafine online pharmacy
online pharmacy no prescription ambien: Benicar – Zerit
https://onlineph24.com/# ed pills online
online pharmacy painkillers uf pharmacy online refill accredo pharmacy
precision rx pharmacy: how much does percocet cost at the pharmacy – indomethacin capsules pharmacy
https://drstore24.com/# circle rx pharmacy
bupropion pharmacy pharmacy assistant course online online pharmacy programs
best online pharmacy viagra review: tylenol 3 pharmacy – simvastatin kmart pharmacy
https://onlineph24.com/# Female Viagra
rite aid pharmacy allegra legit online pharmacy klonopin viagra pharmacy thailand
can i buy viagra from pharmacy: pharmacy online shopping – risperidone online pharmacy
https://easydrugrx.com/# buy growth hormone online pharmacy
letrozole online pharmacy skip’s pharmacy low dose naltrexone online pharmacy no prescription flagyl
https://drstore24.com/# advair online pharmacy
pharmacy choice acyclovir cold sore cream viagra us pharmacy best online pharmacy levitra
harlem rx pharmacy: cialis online review online pharmacy – correct rx pharmacy services
https://pharm24on.com/# permethrin cream online pharmacy
online pharmacy store usa
uk pharmacy viagra prices pharmacy propecia generic or concerta pharmacy coupons
https://www.google.ht/url?q=https://drstore24.com ca board of pharmacy
guardian pharmacy propecia rx express pharmacy hurley ms and concerta pharmacy prices no prescription online pharmacy ua products percocet
generic levitra online pharmacy: best online pharmacy to buy accutane – doxycycline the generics pharmacy
specialty rx pharmacy boots pharmacy uk propecia or elavil online pharmacy
https://www.google.com.vn/url?sa=t&url=https://onlineph24.com online pharmacy ambien generic
unicare pharmacy artane get cialis online pharmacy and advair pharmacy coupons buspirone online pharmacy
clomid in pharmacy certified pharmacy online viagra or pharmacy rx solutions
https://maps.google.lk/url?q=https://easydrugrx.com evelyn bradley pharmacy artane
tesco pharmacy doxycycline cost mexican pharmacy viagra online and asda pharmacy ventolin top online pharmacy india
overseas pharmacy adipex: river pharmacy topamax – xlpharmacy review viagra
https://pharm24on.com/# ativan no prescription online pharmacy
peoples drug store cialis cheap online pharmacy osco pharmacy
https://drstore24.com/# clopidogrel online pharmacy
top online pharmacy homepage online pharmacy percocet
allegheny county real estate I appreciate you sharing this blog post. Thanks Again. Cool.
online generic pharmacy: benzodiazepines online pharmacy – pharmacy support viagra
generic cialis best pharmacy: schnucks pharmacy buttler hill rd store hours – bay rx pharmacy
ibuprofen pharmacy only: ritalin online pharmacy – online pharmacy pain meds
https://easydrugrx.com/# online pharmacy metronidazole
simvastatin uk pharmacy Flagyl fluconazole mexico pharmacy
https://onlineph24.com/# naltrexone river pharmacy
us pharmacy cialis online best australian online pharmacy caring pharmacy viagra
Tech to Force I very delighted to find this internet site on bing, just what I was searching for as well saved to fav
xenical indian pharmacy: online pharmacy not requiring prescription – med pharmacy
lortab pharmacy prices pharmacy online shopping or online pharmacy degree programs
https://maps.google.com.kw/url?sa=t&url=https://drstore24.com provigil online pharmacy
montelukast pharmacy prozac pharmacy online and misoprostol malaysia pharmacy Retrovir
https://pharm24on.com/# care rx specialty pharmacy
percocet no prescription pharmacy provigil online pharmacy no prescription world pharmacy rx
clozapine online pharmacy: price of percocet at pharmacy – xlpharmacy generic cialis
https://onlineph24.com/# cialis american pharmacy
pharmacy cost viagra meijer pharmacy viagra viagra american pharmacy
veterans online pharmacy terbinafine online pharmacy or rite aid pharmacy store closings
https://cse.google.ht/url?sa=t&url=https://onlineph24.com revive rx pharmacy
eu online pharmacy pharmacy uk and bupropion xl pharmacy priligy in malaysia+pharmacy
generic lexapro online pharmacy: Tofranil – hepatitis c virus (hcv)
pharmacies online: online pharmacy no prior prescription – pharmacy cheap
Normally I do not read article on blogs however I would like to say that this writeup very forced me to try and do so Your writing style has been amazed me Thanks quite great post
mexico drug stores pharmacies mexico pharmacies prescription drugs mexican rx online
mexico drug stores pharmacies: buying prescription drugs in mexico online – purple pharmacy mexico price list
https://pharmbig24.online/# online pharmacy prozac no prescription
reputable mexican pharmacies online: best online pharmacies in mexico – buying prescription drugs in mexico online
buy medicines online in india: indian pharmacy paypal – online shopping pharmacy india
https://pharmbig24.com/# acyclovir pharmacy
us online pharmacy cialis: o reilly pharmacy artane – finpecia swiss pharmacy
http://indianpharmacy.company/# reputable indian online pharmacy
mexico drug stores pharmacies: mexican online pharmacies prescription drugs – mexico drug stores pharmacies
pharmacy website india: best india pharmacy – buy medicines online in india
india pharmacy top online pharmacy india or online shopping pharmacy india
https://clients1.google.cv/url?q=https://indianpharmacy.company india online pharmacy
top online pharmacy india mail order pharmacy india and Online medicine home delivery india online pharmacy
buying from online mexican pharmacy best online pharmacies in mexico buying prescription drugs in mexico online
buying prescription drugs in mexico buying from online mexican pharmacy or best online pharmacies in mexico
https://cse.google.dm/url?q=https://mexicopharmacy.cheap mexico pharmacies prescription drugs
medication from mexico pharmacy mexico drug stores pharmacies and mexican rx online pharmacies in mexico that ship to usa
pharmacy cialis online: pharmacy rx one – flovent online pharmacy
http://mexicopharmacy.cheap/# п»їbest mexican online pharmacies
online pharmacy buy adipex medicine online order or buy zithromax online pharmacy no prescription needed
https://www.google.gg/url?q=https://pharmbig24.com viagra generic online pharmacy
vipps certified online pharmacy list buy provigil online pharmacy and overnight pharmacy priligy mexico online pharmacy
mexican online pharmacies prescription drugs: pharmacies in mexico that ship to usa – buying from online mexican pharmacy
https://mexicopharmacy.cheap/# medicine in mexico pharmacies
mexico pharmacies prescription drugs medication from mexico pharmacy or mexican drugstore online
https://www.avenue-x.com/cgi-bin/gforum.cgi?url=https://mexicopharmacy.cheap best online pharmacies in mexico
mexican mail order pharmacies buying prescription drugs in mexico online and buying prescription drugs in mexico online mexico drug stores pharmacies
world pharmacy india: india pharmacy mail order – reputable indian pharmacies
cialis united pharmacy legal online pharmacy percocet levitra prices pharmacy
lasix online pharmacy: what does rx mean in pharmacy – keflex online pharmacy
http://indianpharmacy.company/# online pharmacy india
propranolol online pharmacy: phenytoin clinical pharmacy – Aebgpaype
https://pharmbig24.com/# can you buy clomid from a pharmacy
mexican online pharmacies prescription drugs: mexican online pharmacies prescription drugs – buying from online mexican pharmacy
reputable mexican pharmacies online reputable mexican pharmacies online reputable mexican pharmacies online
reputable indian online pharmacy top 10 pharmacies in india or mail order pharmacy india
http://www.sellere.de/url?q=https://indianpharmacy.company indian pharmacy online
online shopping pharmacy india world pharmacy india and cheapest online pharmacy india indianpharmacy com
top 10 pharmacies in india: best online pharmacy india – best india pharmacy
non prescription online pharmacy reviews: meijer pharmacy free lipitor – minocycline online pharmacy
https://indianpharmacy.company/# india online pharmacy
online pharmacy metronidazole 500mg online pharmacy c o d or buy cialis cheap us pharmacy
https://toolbarqueries.google.com.eg/url?sa=t&url=https://pharmbig24.com reputable online pharmacy
boot pharmacy store locator giant food store phoenixville pharmacy and cialis cheap online pharmacy target pharmacy levitra
Thanks in favor of sharing such a good thinking, piece of writing is fastidious, thats why i have read it fully
п»їbest mexican online pharmacies mexico pharmacies prescription drugs or pharmacies in mexico that ship to usa
https://smootheat.com/contact/report?url=https://mexicopharmacy.cheap reputable mexican pharmacies online
reputable mexican pharmacies online buying prescription drugs in mexico and mexican border pharmacies shipping to usa best online pharmacies in mexico
This post is priceless. When can I find out more?
indian pharmacy paypal buy prescription drugs from india online shopping pharmacy india
Fine way of describing, and fastidious piece of writing to get facts about my presentation focus,
which i am going to convey in university.
п»їbest mexican online pharmacies: mexico pharmacies prescription drugs – mexican border pharmacies shipping to usa
medicine in mexico pharmacies buying prescription drugs in mexico online or buying from online mexican pharmacy
https://www.google.dj/url?q=https://mexicopharmacy.cheap purple pharmacy mexico price list
mexican mail order pharmacies purple pharmacy mexico price list and pharmacies in mexico that ship to usa п»їbest mexican online pharmacies
http://mexicopharmacy.cheap/# purple pharmacy mexico price list
top 10 online pharmacy in india: indian pharmacy – indian pharmacies safe
п»їbest mexican online pharmacies: п»їbest mexican online pharmacies – reputable mexican pharmacies online
http://mexicopharmacy.cheap/# purple pharmacy mexico price list
pharmacy website india indian pharmacy online Online medicine home delivery
of course like your website but you have to check the spelling on several of your posts A number of them are rife with spelling issues and I in finding it very troublesome to inform the reality on the other hand I will certainly come back again
online pharmacy nizoral: cheapest pharmacy to fill prescriptions with insurance – propecia uk pharmacy
viagra from vipps pharmacy: can buy viagra pharmacy – pharmacy store window
http://mexicopharmacy.cheap/# п»їbest mexican online pharmacies
world pharmacy india best online pharmacy india or world pharmacy india
https://cse.google.gr/url?sa=t&url=https://indianpharmacy.company india pharmacy
indian pharmacies safe mail order pharmacy india and pharmacy website india indian pharmacy
indian pharmacy paypal: indian pharmacy – online shopping pharmacy india
http://indianpharmacy.company/# indian pharmacies safe
australian pharmacy online clindamycin uk pharmacy or people’s pharmacy nexium
http://finalls.ru/forum/away.php?s=https://pharmbig24.com online pharmacy buy viagra
medco pharmacy cialis Kamagra Polo and freedom pharmacy mexican pharmacy online percocet
buying prescription drugs in mexico purple pharmacy mexico price list or buying from online mexican pharmacy
https://maps.google.ht/url?q=https://mexicopharmacy.cheap buying prescription drugs in mexico online
medication from mexico pharmacy reputable mexican pharmacies online and medication from mexico pharmacy buying prescription drugs in mexico online
Online medicine home delivery indian pharmacy online Online medicine home delivery
mexico drug stores pharmacies: mexican mail order pharmacies – reputable mexican pharmacies online
no rx pharmacy: viagra european pharmacy – all rx pharmacy
https://indianpharmacy.company/# п»їlegitimate online pharmacies india
pharmacy novo venlafaxine xr: best online pharmacy – online pharmacy no prescription needed klonopin
india pharmacy mail order best online pharmacy india cheapest online pharmacy india
http://pharmbig24.com/# pharmacy uk
top 10 online pharmacy in india: india pharmacy – india online pharmacy
pharmacies in mexico that ship to usa mexico drug stores pharmacies or buying prescription drugs in mexico online
http://atoo.su/redirect.php?url=http://mexicopharmacy.cheap best online pharmacies in mexico
purple pharmacy mexico price list п»їbest mexican online pharmacies and pharmacies in mexico that ship to usa medicine in mexico pharmacies
buy prescription drugs from india: Online medicine home delivery – top online pharmacy india
https://pharmbig24.online/# prescription discount
world pharmacy india buy prescription drugs from india or best india pharmacy
http://ccasayourworld.com/?URL=indianpharmacy.company best india pharmacy
indian pharmacy paypal online shopping pharmacy india and п»їlegitimate online pharmacies india indian pharmacy online
express pharmacy online cholesterol ketoconazole shampoo pharmacy
buying prescription drugs in mexico online pharmacies in mexico that ship to usa or pharmacies in mexico that ship to usa
http://sat-plus.net/ext_link?url=https://mexicopharmacy.cheap mexican drugstore online
buying from online mexican pharmacy medicine in mexico pharmacies and medicine in mexico pharmacies best online pharmacies in mexico
pharmacy clothing store: provigil pharmacy – cheapest pharmacy viagra
on line pharmacy pharmacy store fixtures or pharmacy methotrexate error
http://marcina.net/info.php?a=generic+cialis triamcinolone cream online pharmacy
provigil pharmacy pharmacy online store and levitra uk pharmacy can i buy viagra at a pharmacy
Atorlip-20: india pharmacy cymbalta – buy nolvadex online pharmacy
india pharmacy mail order: indianpharmacy com – buy medicines online in india
http://mexicopharmacy.cheap/# п»їbest mexican online pharmacies
https://pharmbig24.com/# fred meyer pharmacy hours
I read this post fully regarding the comparison of most up-to-date and previous technologies, it’s amazing article.
Here is my site USA Script Helpers
mexican drugstore online mexico drug stores pharmacies mexican mail order pharmacies
top 10 pharmacies in india: online pharmacy india – mail order pharmacy india
obviously like your website but you need to test the spelling on quite a few of your posts Several of them are rife with spelling problems and I to find it very troublesome to inform the reality on the other hand Ill certainly come back again
buying prescription drugs in mexico online: reputable mexican pharmacies online – buying prescription drugs in mexico
online dog pharmacy: rite aid pharmacy viagra price – 4 corners pharmacy flovent
https://pharmbig24.online/# aarp medicare rx pharmacy directory
mexico pharmacies prescription drugs buying prescription drugs in mexico buying prescription drugs in mexico
Hi there, I enjoy reading all of your article post. I wanted to write a little comment to support
you.
http://indianpharmacy.company/# reputable indian pharmacies
indian pharmacies safe: world pharmacy india – india online pharmacy
online shopping pharmacy india cheapest online pharmacy india or world pharmacy india
https://www.google.com.vn/url?sa=t&url=https://indianpharmacy.company cheapest online pharmacy india
india pharmacy mail order indianpharmacy com and reputable indian pharmacies top online pharmacy india
mexican rx online п»їbest mexican online pharmacies or mexico pharmacies prescription drugs
http://cse.google.bs/url?sa=t&url=http://mexicopharmacy.cheap buying from online mexican pharmacy
mexico drug stores pharmacies mexican drugstore online and mexico drug stores pharmacies п»їbest mexican online pharmacies
mebendazole online pharmacy wegmans pharmacy or rite aid pharmacy store hours
https://www.dasha.com.ua/redirect.php?redir=http://pharmbig24.com/ neurontin online pharmacy
generic viagra online canadiain pharmacy can you buy viagra at a pharmacy and percocet pharmacy topical rx pharmacy tallahassee fl
Thanks to my father who told me about this blog, this website is truly amazing.
п»їbest mexican online pharmacies: medicine in mexico pharmacies – medication from mexico pharmacy
https://mexicopharmacy.cheap/# mexican online pharmacies prescription drugs
mexican rx online: buying from online mexican pharmacy – mexican rx online
mexican mail order pharmacies mexican online pharmacies prescription drugs or mexico drug stores pharmacies
https://www.techjobscafe.com/goto.php?s=Top&goto=https://mexicopharmacy.cheap mexico drug stores pharmacies
medication from mexico pharmacy buying from online mexican pharmacy and mexican drugstore online mexico pharmacies prescription drugs
If some one wants to be updated with newest technologies then he must be pay
a visit this web page and be up to date
daily.
hair loss buy viagra usa pharmacy best retail pharmacy viagra price
I’m not that much of a online reader to be honest
but your sites really nice, keep it up! I’ll go ahead and bookmark your site
to come back in the future. All the best
online pharmacy india: indian pharmacy paypal – reputable indian pharmacies
naturally like your web-site but you need to check the spelling on quite a few of your posts.
Many of them are rife with spelling issues and I find
it very troublesome to tell the reality however I’ll definitely come back
again.
indianpharmacy com: best online pharmacy india – world pharmacy india
http://mexicopharmacy.cheap/# mexico pharmacies prescription drugs
I do not know whether it’s just me or if everyone else encountering issues
with your site. It seems like some of the written text in your posts
are running off the screen. Can someone else please comment and let me know if
this is happening to them too? This could be a issue with my internet browser because I’ve had
this happen before. Many thanks
It’s difficult to find experienced people in this particular subject, however, you seem like you know what you’re talking about!
Thanks
Ahaa, its fastidious discussion on the topic of this post at this place at this blog, I have read all that, so now me also commenting here.
straz bet starzbet giris straz bet
casibom: casibom guncel – casibom giris
https://starzbet.shop/# starzbet guncel giris
betine guncel: betine guncel giris – betine promosyon kodu 2024
gate of olympus oyna gates of olympus giris gate of olympus oyna
casibom giris: casibom 158 giris – casibom 158 giris
https://casibom.auction/# casibom
casibom giris casibom guncel giris casibom guncel
dewadora
You made some really good points there. I checked on the internet for additional information about the issue and found most people will go along
with your views on this site.
You have made some really good points there. I looked on the internet for additional information about the issue and found most individuals will go along with your views on this web site.
Gates of Olympus: gates of olympus demo turkce – gate of olympus oyna
https://betine.online/# betine com guncel giris
betine sikayet: betine promosyon kodu – betine giris
gates of olympus demo turkce gates of olympus turkce gates of olympus demo oyna
https://casibom.auction/# casibom guncel
I’m not sure where you are getting your info, but good topic.
I needs to spend some time learning more or understanding more.
Thanks for great information I was looking for this info for my mission.
casibom guncel giris adresi: casibom guncel giris – casibom guncel giris
https://casibom.auction/# casibom guncel giris
gate of olympus oyna gates of olympus oyna demo gates of olympus giris
casibom guncel giris: casibom giris adresi – casibom
http://betine.online/# betine
straz bet starzbet guncel giris or starzbet guvenilir mi
https://couponifier.com/store/starzbet.shop starzbet guvenilir mi
starzbet guvenilir mi starzbet guncel giris and starzbet giris starzbet guncel giris
casibom giris adresi: casibom giris – casibom guncel giris
gates of olympus demo turkce gates of olympus demo turkce gates of olympus oyna demo
casibom giris casibom guncel giris or casibom guncel giris
https://www.google.com.ar/url?q=https://casibom.auction casibom guncel giris adresi
casibom 158 giris casibom guncel giris and casibom guncel giris adresi casibom giris
betine guncel giris betine or betine giris
http://www.kip-k.ru/best/sql.php?=betine.online betine com guncel giris
betine guncel betine guncel and betine com guncel giris betine giris
casibom 158 giris: casibom – casibom giris adresi
http://casibom.auction/# casibom giris
https://starzbet.shop/# starz bet giris
gates of olympus demo oyna: gates of olympus demo oyna – gates of olympus oyna
https://starzbet.shop/# starzbet guvenilir mi
gates of olympus demo turkce gates of olympus demo turkce oyna or gates of olympus oyna
https://www.google.mn/url?q=https://gatesofolympusoyna.online Gates of Olympus
gates of olympus turkce gates of olympus demo turkce and gates of olympus slot gates of olympus demo oyna
straz bet: straz bet – starzbet guncel giris
straz bet starzbet guncel giris starzbet guncel giris
starzbet guvenilir mi: starzbet guncel giris – starzbet
http://gatesofolympusoyna.online/# gates of olympus demo
casibom guncel giris adresi: casibom giris adresi – casibom giris
https://casibom.auction/# casibom guncel giris
betine guncel giris: betine guncel – betine
starzbet guncel giris straz bet starzbet guvenilir mi
http://gatesofolympusoyna.online/# gates of olympus demo
betine com guncel giris betine guncel giris betine guncel giris
When I initially commented I clicked the “Notify me when new comments are added” checkbox and now
each time a comment is added I get several emails with the same comment.
Is there any way you can remove people from that
service? Many thanks!
starzbet guvenilir mi: starzbet guncel giris – starzbet guvenilir mi
https://starzbet.shop/# starzbet guncel giris
betine guncel betine guncel giris or betine promosyon kodu 2024
https://www.lolinez.com/?http://betine.online betine
betine sikayet betine giris and betine betine com guncel giris
straz bet: starzbet guvenilir mi – starzbet guncel giris
starzbet giris starzbet guncel giris starzbet giris
casibom guncel giris: casibom guncel giris – casibom 158 giris
http://casibom.auction/# casibom guncel giris adresi
gates of olympus turkce gates of olympus demo oyna or gates of olympus demo turkce
http://www.opentrad.com/margen.php?direccion=gl-pt&inurl=gatesofolympusoyna.online/ Gates of Olympus
gates of olympus demo turkce gates of olympus demo turkce oyna and gate of olympus oyna gates of olympus oyna demo
starzbet guncel giris starzbet guvenilir mi starzbet guvenilir mi
http://betine.online/# betine com guncel giris
viagra para hombre precio farmacias: viagra precio – viagra online cerca de la coruГ±a
farmacia barata: Cialis precio – farmacias online seguras en espaГ±a
https://tadalafilo.bid/# farmacias online seguras
sildenafilo precio farmacia sildenafilo viagra online cerca de zaragoza
https://farmaciaeu.com/# farmacia online madrid
farmacias online seguras
farmacias online baratas: farmacias online seguras – farmacia online envГo gratis
Зарегистрируйтесь прямо сейчас и получите 100 фриспинов без депозита, чтобы испытать свою удачу в увлекательных играх и повысить свои шансы на крупный выигрыш. рейтинг казино онлайн xztzvrnhoi …
erek erek 99
Quality content is the important to attract the people to pay a visit the site, that’s what this website is providing.
farmacias direct farmacia online envio gratis murcia farmacias online seguras en espaГ±a
I’m extremely impressed with your writing
skills as well as with the layout on your blog. Is this a paid theme or did
you modify it yourself? Either way keep up the nice
quality writing, it is rare to see a great blog like this one these days.
farmacia online 24 horas farmacia online barcelona or farmacias online seguras
https://www.google.ws/url?q=https://farmaciaeu.com::: farmacias online baratas
farmacia online barata y fiable farmacias direct and farmacia online barata farmacias online baratas
farmacia online barata farmacia online barcelona or farmacia online madrid
https://images.google.lu/url?q=https://farmaciaeu.com farmacia barata
п»їfarmacia online espaГ±a farmacia online envГo gratis and farmacias online baratas farmacia online madrid
Howdy I am so grateful I found your site, I really found you
by error, while I was searching on Bing for something else,
Anyhow I am here now and would just like to say thanks for a marvelous post and a all round enjoyable blog (I also love the theme/design), I don’t have
time to go through it all at the minute but I have bookmarked it and also included your RSS feeds, so when I have time I will be back to read more, Please do keep up the fantastic job.
Unquestionably believe that that you said. Your favorite reason appeared to be at the internet the simplest thing to keep in mind of.
I say to you, I definitely get annoyed while people consider
worries that they plainly do not realize about.
You controlled to hit the nail upon the top and outlined
out the whole thing without having side-effects , people can take
a signal. Will probably be back to get more. Thanks
https://tadalafilo.bid/# farmacias online seguras en espaГ±a
farmacia barata
farmacia online madrid: Comprar Cialis sin receta – farmacias online baratas
chord resah jadi luka
I visited many sites however the audio feature for audio songs
existing at this web page is in fact superb.
blockaway
I believe that is one of the so much important information for me.
And i am happy reading your article. But wanna commentary on few common issues, The website style is ideal, the articles is in point of fact excellent
: D. Excellent task, cheers
http://farmaciaeu.com/# farmacia en casa online descuento
farmacias direct
farmacia online 24 horas: farmacia 24 horas – farmacia online espaГ±a envГo internacional
What’s up mates, its great post regarding educationand entirely explained, keep it up all the time.
Here is my homepage กัญชาทางการแพทย์
farmacia online barata y fiable farmacia online barcelona or п»їfarmacia online espaГ±a
http://www.grandhotelnizza.it/gallery/imagevue/phpinfo.php?a=tadalafil without a doctor’s prescription farmacia online espaГ±a envГo internacional
farmacia online espaГ±a envГo internacional farmacia online espaГ±a envГo internacional and farmacia online barcelona farmacia online 24 horas
comprar viagra contrareembolso 48 horas sildenafilo cinfa 100 mg precio farmacia or sildenafilo sandoz 100 mg precio
https://cse.google.co.je/url?q=https://sildenafilo.men sildenafilo cinfa precio
viagra para hombre venta libre comprar sildenafilo cinfa 100 mg espaГ±a and comprar viagra online en andorra comprar viagra en espaГ±a envio urgente contrareembolso
viagra online spedizione gratuita: miglior sito per comprare viagra online – viagra consegna in 24 ore pagamento alla consegna
birutoto birutoto
birutoto birutoto
You are so interesting! I don’t suppose I’ve read through something like that before.
So good to discover someone with a few genuine thoughts on this subject matter.
Seriously.. thank you for starting this up. This website is something that’s needed on the web, someone with a bit of
originality!
This is a topic that is close to my heart…
Thank you! Exactly where are your contact details though?
farmaci senza ricetta elenco farmacia online migliore Farmacia online miglior prezzo
of course like your web site however you need to test the spelling on quite
a few of your posts. A number of them are rife with spelling problems and I to
find it very bothersome to tell the reality on the other hand
I will certainly come again again.
п»їFarmacia online migliore: Farmacie on line spedizione gratuita – acquisto farmaci con ricetta
farmaci senza ricetta elenco: Cialis generico farmacia – Farmacie on line spedizione gratuita
A fascinating discussion is definitely worth comment.
I believe that you should publish more on this subject matter, it may not be a taboo matter but usually people
don’t talk about these subjects. To the next!
Cheers!!
acquisto farmaci con ricetta Cialis generico 5 mg prezzo Farmacie online sicure
cialis farmacia senza ricetta: acquisto viagra – viagra online in 2 giorni
Heya i’m for the first time here. I came across this board and I
find It truly useful & it helped me out much. I hope to give something
back and aid others like you helped me.
top farmacia online Farmacia online piu conveniente top farmacia online
farmacie online autorizzate elenco: Brufen 600 prezzo con ricetta – Farmacia online miglior prezzo
You can certainly see your skills in the work you write. The
arena hopes for more passionate writers such as you who are not afraid to say how they believe.
At all times go after your heart.
I just could not leave your site before suggesting that I really loved the standard information a person supply
on your visitors? Is gonna be back incessantly to check up on new posts
comprare farmaci online con ricetta acquisto farmaci con ricetta or Farmacia online piГ№ conveniente
http://www.sprang.net/url?q=https://farmaciait.men top farmacia online
farmacie online affidabili comprare farmaci online con ricetta and farmacia online senza ricetta farmacie online autorizzate elenco
farmacia online senza ricetta: comprare farmaci online all’estero – farmaci senza ricetta elenco
farmacie online autorizzate elenco Brufen 600 senza ricetta acquisto farmaci con ricetta
п»їFarmacia online migliore Ibuprofene 600 prezzo senza ricetta farmacia online senza ricetta
siti sicuri per comprare viagra online acquisto viagra alternativa al viagra senza ricetta in farmacia
Hello, this weekend is fastidious for me, for the reason that this occasion i am reading this great informative article here at my house.
viagra acquisto in contrassegno in italia viagra consegna in 24 ore pagamento alla consegna or viagra generico sandoz
https://images.google.com.vn/url?sa=t&url=https://sildenafilit.pro alternativa al viagra senza ricetta in farmacia
cialis farmacia senza ricetta cialis farmacia senza ricetta and viagra generico sandoz viagra online spedizione gratuita
farmacie online affidabili: BRUFEN 600 acquisto online – Farmacia online piГ№ conveniente
п»їFarmacia online migliore Farmacia online piГ№ conveniente or comprare farmaci online con ricetta
http://www.weedy.be/2-uncategorised/1320-redirect2?url=http://farmaciait.men Farmacia online miglior prezzo
farmacia online piГ№ conveniente Farmacia online piГ№ conveniente and farmacia online acquistare farmaci senza ricetta
Farmacia online miglior prezzo: Farmacia online piu conveniente – acquisto farmaci con ricetta
farmacie online sicure Cialis generico farmacia comprare farmaci online con ricetta
Farmacia online piГ№ conveniente BRUFEN 600 prezzo in farmacia migliori farmacie online 2024
farmacie online sicure: Ibuprofene 600 generico prezzo – farmacie online sicure
farmacia online senza ricetta BRUFEN 600 bustine prezzo farmacia online senza ricetta
Currently it appears like Expression Engine is the preferred blogging platform available right now.
(from what I’ve read) Is that what you’re using on your
blog?
Farmacie online sicure Cialis generico 5 mg prezzo farmacie online autorizzate elenco
migliori farmacie online 2024: Ibuprofene 600 generico prezzo – п»їFarmacia online migliore
alternativa al viagra senza ricetta in farmacia: viagra – esiste il viagra generico in farmacia
viagra subito pillole per erezione in farmacia senza ricetta or miglior sito per comprare viagra online
http://englmaier.de/url?q=https://sildenafilit.pro miglior sito per comprare viagra online
viagra online spedizione gratuita cialis farmacia senza ricetta and miglior sito per comprare viagra online viagra consegna in 24 ore pagamento alla consegna
Greetings! Very useful advice in this particular
article! It is the little changes that make the most significant changes.
Many thanks for sharing!
When someone writes an piece of writing he/she keeps the thought of a user in his/her mind that how a user can know it.
Thus that’s why this post is great. Thanks!
п»їFarmacia online migliore Cialis generico 20 mg 8 compresse prezzo Farmacie on line spedizione gratuita
farmacia online piГ№ conveniente acquisto farmaci con ricetta or comprare farmaci online con ricetta
https://cse.google.com.br/url?sa=t&url=https://farmaciait.men farmaci senza ricetta elenco
Farmacia online piГ№ conveniente migliori farmacie online 2024 and farmacie online autorizzate elenco top farmacia online
Farmacia online piГ№ conveniente farmacia online migliore migliori farmacie online 2024
acquisto farmaci con ricetta: BRUFEN 600 bustine prezzo – migliori farmacie online 2024
These are really enormous ideas in about
blogging. You have touched some nice factors here. Any way
keep up wrinting.
ventolin 200: buy albuterol inhaler – cheap ventolin
ventolin inhalador: Buy Albuterol inhaler online – ventolin 4mg
prednisone 20mg by mail order: apo prednisone – prednisone daily
ventolin drug: Ventolin inhaler – ventolin prescription discount
prednisone 40 mg daily: prednisone cost in india – buy prednisone online without a script
neurontin 100mg cost generic gabapentin or neurontin 100 mg tablets
https://www.google.com.ng/url?sa=t&url=http://gabapentin.site neurontin 300 mg buy
neurontin 400 mg neurontin cap and neurontin neurontin price uk
rybelsus cost: rybelsus – Buy compounded semaglutide online
Bu soba, içindeki yakıtın yanmasıyla oluşan ısıyı doğrudan çevresine yayar ve aynı zamanda suyun ısınmasını sağlar.
neurontin online: neurontin 900 – gabapentin 300
Pretty nice post. I simply stumbled upon your weblog and wanted to say that I have really loved surfing around your
blog posts. After all I will be subscribing on your rss feed
and I’m hoping you write once more soon!
You’ve made some really good points there. I checked on the
net for additional information about the issue and found most people will go along with your views on this website.
I have read so many content on the topic of the blogger lovers however
this paragraph is truly a fastidious article, keep it up.
Greetings, I do believe your website could be having web browser compatibility problems.
When I take a look at your website in Safari, it looks fine but when opening in I.E.,
it has some overlapping issues. I just wanted to provide you with a quick heads up!
Besides that, great site!
Family Dollar I just like the helpful information you provide in your articles
Thank you for the good writeup. It in fact was a amusement account it.
Look advanced to more added agreeable from
you! By the way, how can we communicate?
Viagra homme sans ordonnance belgique viagra en ligne Viagra homme prix en pharmacie sans ordonnance
بلاگ خودرویی در وب سایت نوربرت پرفورمنس که یک وب سایت فوق العاده عالی
و معتبر میباشد
Your mode of explaining everything in this post is genuinely nice, every one be capable of without
difficulty understand it, Thanks a lot.
pharmacie en ligne pas cher cialis sans ordonnance Achat mГ©dicament en ligne fiable
At this time I am going to do my breakfast, after having my breakfast
coming over again to read additional news.
http://clssansordonnance.icu/# pharmacies en ligne certifiГ©es
Achat mГ©dicament en ligne fiable: cialis generique – pharmacie en ligne sans ordonnance
I simply couldn’t leave your website prior to suggesting that I actually loved the standard
info a person supply in your guests? Is going to be again often in order to check out new posts
Le gГ©nГ©rique de Viagra Viagra generique en pharmacie Quand une femme prend du Viagra homme
Sweet blog! I found it while surfing around on Yahoo News.
Do you have any tips on how to get listed in Yahoo News?
I’ve been trying for a while but I never seem to get there!
Appreciate it
Watch Indian Porn gay porn videos for free, here on Pornhub.com.
Discover the growing collection of high quality Most Relevant gay XXX
movies and clips. सुंदर भारतीय समलैंगिक पुरुष हॉट ब्लोजॉब और हार्डकोर वीडियो
में स्टार हैं, और अधिकांश समलैंगिक शौकिया हैं
जो लंड के साथ मस्ती करते हैं। सेक्सी गुदा मैथुन और
उँगलियों से चुदाई देखें
I used to be able to find good advice from your blog articles.
اویل کش (Oil Catch Can) یک قطعه مهم در سیستمهای موتور
است که به منظور جلوگیری از ورود بخارات روغن و گازهای غیرسوختی به سیستم ورودی
هوا و محفظه احتراق طراحی شده
است. این دستگاه بهخصوص در خودروهای
با موتورهای قدرتمند، تقویتشده و مسابقهای مورد استفاده قرار میگیرد.
در ادامه به بررسی جزئیات کامل اویل کش،
نحوه عملکرد، مزایا، معایب و کاربردهای آن خواهیم پرداخت.
نوربرت پرفورمنس
Thanks for sharing your thoughts about ngentot nungging. Regards
Woah! I’m really digging the template/theme of this website.
It’s simple, yet effective. A lot of times it’s challenging to get that “perfect balance” between usability and visual appeal.
I must say you’ve done a great job with this. In addition,
the blog loads very quick for me on Opera. Excellent Blog!
This blog was… how do you say it? Relevant!! Finally I have found something which helped me.
Kudos!
Valuable info. Fortunate me I discovered your website
by chance, and I’m stunned why this twist of fate didn’t took
place in advance! I bookmarked it.
This paragraph is really a fastidious one it helps new the web users, who are wishing in favor of blogging.
I have been surfing online more than 2 hours today, yet I never found any interesting article like yours.
It’s pretty worth enough for me. Personally, if all webmasters and bloggers made good content as you
did, the net will be much more useful than ever
before.
I’m gone to convey my little brother, that he should
also go to see this weblog on regular basis to take updated from most recent reports.
What a data of un-ambiguity and preserveness of valuable familiarity concerning unexpected
feelings.
A person necessarily assist to make seriously posts I would state.
This is the first time I frequented your web page and to this
point? I surprised with the analysis you made to make this particular submit incredible.
Magnificent activity!
It’s very straightforward to find out any topic on web as compared to books, as I found this article at this
web site.
slot demo slot demo slot demo
Hi there I am so delighted I found your webpage, I really found you by mistake, while I
was researching on Digg for something else, Nonetheless I am here now
and would just like to say many thanks for a incredible post and a all round interesting blog (I
also love the theme/design), I don’t have time to read it all
at the moment but I have saved it and also added your RSS feeds,
so when I have time I will be back to read much more, Please do keep
up the superb job.
منیفولد تقویتی xu7 تولید شده توسط نوربرت پرفورمنس با کیفیت عالی
Hello, all the time i used to check blog posts here
early in the dawn, for the reason that i love to gain knowledge
of more and more.
Hi there would you mind letting me know which web
host you’re utilizing? I’ve loaded your blog in 3 completely different internet browsers and I must say
this blog loads a lot faster then most. Can you recommend a good web hosting provider at a reasonable price?
Many thanks, I appreciate it!
Hey there would you mind letting me know which web host you’re using?
I’ve loaded your blog in 3 completely different browsers and
I must say this blog loads a lot quicker then most.
Can you recommend a good hosting provider at a fair price?
Many thanks, I appreciate it!
After I originally left a comment I appear to
have clicked on the -Notify me when new comments are
added- checkbox and now each time a comment is added
I get 4 emails with the exact same comment. Perhaps there is a way you
are able to remove me from that service? Thank you!
Good site you’ve got here.. It’s difficult to find good quality writing like
yours nowadays. I truly appreciate people like you! Take care!!
Aw, this was a very nice post. Spending some time and actual effort to make a really
good article… but what can I say… I put things off a whole
lot and never seem to get anything done.
Normally I do not read post on blogs, however I would like to
say that this write-up very pressured me to try
and do so! Your writing taste has been amazed me.
Thanks, very nice article.
Hey there would you mind stating which blog platform
you’re working with? I’m looking to start my own blog
soon but I’m having a hard time making a decision between BlogEngine/Wordpress/B2evolution and Drupal.
The reason I ask is because your layout seems different then most
blogs and I’m looking for something unique.
P.S Apologies for getting off-topic but I had to
ask!
It’s very effortless to find out any topic on web as compared to textbooks, as I found this post at
this website.
If some one needs to be updated with latest technologies therefore he must
be pay a quick visit this site and be up to date all
the time.
My brother recommended I might like this blog. He was totally
right. This post actually made my day. You can not imagine just how much time I had spent for
this information! Thanks!
Thank you for the auspicious writeup. It in fact was
a amusement account it. Look advanced to far added agreeable from you!
By the way, how can we communicate?
لوازم موتوری اصلی و تقویت شده در وب سایت نوربرت پرفورمنس با بهترین و عالی ترین
کیفیت موجود در جهان
Do you have any video of that? I’d like to find out some additional information.
Wonderful blog! I found it while surfing around on Yahoo News.
Do you have any tips on how to get listed in Yahoo News?
I’ve been trying for a while but I never seem to get there!
Cheers
I do trust all of the concepts you’ve introduced in your post.
They are really convincing and can certainly work.
Nonetheless, the posts are very quick for newbies. May you please lengthen them
a bit from next time? Thanks for the post.
Very nice post. I simply stumbled upon your blog and wished to say that I have truly enjoyed surfing around your blog posts.
After all I’ll be subscribing in your rss feed and I am hoping
you write once more soon!
Hello there, You have done a great job. I’ll definitely digg
it and personally suggest to my friends. I am sure they’ll be benefited from this website.
Quality content is the crucial to be a focus for the visitors to pay a visit the web site, that’s what this website is providing.
I was curious if you ever considered changing the structure of your blog?
Its very well written; I love what youve got to say.
But maybe you could a little more in the way of
content so people could connect with it better. Youve
got an awful lot of text for only having 1 or two images.
Maybe you could space it out better?
What’s up, just wanted to tell you, I liked this post.
It was funny. Keep on posting!
hey there and thank you for your information – I’ve definitely picked
up something new from right here. I did however expertise
several technical points using this website, since
I experienced to reload the web site many times previous to I could get
it to load correctly. I had been wondering if your web hosting is OK?
Not that I’m complaining, but sluggish loading instances times will sometimes affect your placement in google and can damage your quality score if advertising and marketing with Adwords.
Well I’m adding this RSS to my email and could look out for a lot
more of your respective intriguing content. Make sure you update this again very soon.
Yes! Finally someone writes about เข้าสู่เกม.
Hey! Would you mind if I share your blog with my myspace group?
There’s a lot of folks that I think would really enjoy your content.
Please let me know. Many thanks
My partner and I stumbled over here from
a different web address and thought I might as well check things out.
I like what I see so now i’m following you.
Look forward to going over your web page repeatedly.
Hey there, You’ve done a fantastic job. I’ll certainly digg it and personally suggest to my friends.
I’m confident they’ll be benefited from this website.
I wanted to thank you for this fantastic read!!
I definitely enjoyed every little bit of it. I have got you book marked
to check out new things you post…
I am really thankful to the owner of this web site
who has shared this impressive paragraph at here.
I have to thank you for the efforts you’ve put in writing this site.
I’m hoping to view the same high-grade blog posts
by you in the future as well. In fact, your creative writing abilities has encouraged me to get my own site now 😉
Hey there just wanted to give you a quick heads up. The text in your content seem to be running off the screen in Safari.
I’m not sure if this is a format issue or something
to do with web browser compatibility but I figured I’d post to let you know.
The design and style look great though! Hope you get the issue solved soon. Thanks
Fine material Thanks!
It’s really a cool and useful piece of info. I am happy that you just shared this helpful info with
us. Please stay us up to date like this. Thank you for sharing.
I’ve been surfing online more than 2 hours today, yet I never found any
interesting article like yours. It is pretty worth enough for
me. In my view, if all web owners and bloggers made good content
as you did, the net will be much more useful than ever before.
Thanks for sharing your thoughts. I really appreciate your
efforts and I will be waiting for your further write ups thanks once again.
Attractive section of content. I just stumbled upon your site and in accession capital to assert that I acquire in fact enjoyed account
your blog posts. Anyway I’ll be subscribing to your augment and even I achievement you access
consistently rapidly.
Thanks for sharing your thoughts about เกมG2G MAJOR.
Regards
After I initially left a comment I seem to have clicked on the -Notify me when new comments are added- checkbox and from now on each time a comment is added I receive 4 emails with the exact same comment.
Is there a means you can remove me from that service?
Many thanks!
I like what you guys tend to be up too. This kind of clever work and reporting!
Keep up the fantastic works guys I’ve incorporated you guys
to blogroll.
Good post. I am dealing with some of these issues as well..
Hello friends, pleasant article and nice urging commented at this place, I am truly enjoying by these.
It’s going to be ending of mine day, except before finish I am reading this fantastic post to increase my know-how.
You can definitely see your enthusiasm within the work
you write. The arena hopes for more passionate writers such as you who aren’t afraid to say how they believe.
All the time go after your heart.
This is a very good tip particularly to those fresh to the blogosphere.
Short but very precise info… Thank you for sharing this one.
A must read article!
hi!,I really like your writing very so much! proportion we communicate more approximately your article
on AOL? I need an expert in this space to resolve my problem.
May be that is you! Having a look forward to see you.
I know this web page presents quality dependent content
and other material, is there any other site which offers these stuff in quality?
Have you ever thought about writing an ebook
or guest authoring on other websites? I have a blog centered on the
same information you discuss and would really like to
have you share some stories/information. I know my viewers would appreciate your
work. If you’re even remotely interested, feel free to shoot me an email.
Hi, I would like to subscribe for this weblog to obtain most up-to-date
updates, therefore where can i do it please help out.
Magnificent website. Lots of helpful information here.
I am sending it to some friends ans also sharing in delicious.
And of course, thank you to your sweat!
We are a group of volunteers and opening a new scheme in our community.
Your website provided us with valuable info to work on. You have done an impressive job and our whole community will be grateful to you.
I would like to thank you for the efforts you have put
in writing this blog. I really hope to view the same high-grade content by you later on as
well. In fact, your creative writing abilities has encouraged me
to get my own website now 😉
This post is actually a nice one it helps new web visitors, who are wishing in favor of blogging.
I think this is one of the most significant information for me.
And i am glad reading your article. But wanna remark on some general things, The website style is
great, the articles is really excellent : D. Good job, cheers
Why users still make use of to read news papers when in this technological globe
everything is presented on net?
Wow, superb blog format! How lengthy have you ever been blogging for?
you made blogging look easy. The whole look of your web site
is excellent, let alone the content material!
pelangi88 pelangi88 pelangi88
of course like your web site however you have to test the spelling on quite a few of your posts.
A number of them are rife with spelling problems and I to find it very troublesome to tell the reality then again I’ll certainly come again again.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Article writing is also a excitement, if you be familiar with afterward you can write otherwise it is difficult to write.
Why visitors still use to read news papers when in this
technological globe the whole thing is accessible on web?
Thanks for the auspicious writeup. It in fact was once a entertainment account it.
Look complicated to far introduced agreeable from you! By the way,
how can we be in contact?
Feel free to visit my blog :: หลักสูตรดำน้ำ
Hi! Do you know if they make any plugins to protect against hackers?
I’m kinda paranoid about losing everything I’ve worked hard on. Any tips?
I was wondering if you ever thought of changing the page layout of your blog?
Its very well written; I love what youve got
to say. But maybe you could a little more in the way of content so people could
connect with it better. Youve got an awful lot of text for only having one or 2 images.
Maybe you could space it out better?
Hi, of course this piece of writing is actually good and I have learned lot of things from it about blogging.
thanks.
Hello friends, its great piece of writing regarding cultureand
entirely defined, keep it up all the time.
Aw, this was an exceptionally nice post. Finding the time and
actual effort to produce a great article… but what can I
say… I hesitate a lot and never manage to get anything done.
Hello! I could have sworn I’ve been to this website before but after reading
through some of the post I realized it’s new to me.
Anyways, I’m definitely delighted I found it and I’ll be bookmarking and checking back often!
I think the admin of this site is actually working hard for his web
page, because here every material is quality based stuff.
Here is my web site … คอร์สเรียนดำน้ำลึก
Can you tell us more about this? I’d like to find out some additional information.
I know this if off topic but I’m looking into starting my own weblog and was wondering what all is needed to get setup?
I’m assuming having a blog like yours would cost a pretty
penny? I’m not very web savvy so I’m not 100% positive.
Any recommendations or advice would be greatly appreciated.
Thanks
you’re in reality a good webmaster. The site loading speed is amazing.
It sort of feels that you’re doing any distinctive trick.
Moreover, The contents are masterwork. you
have performed a excellent job on this subject!
Fran Candelera I am truly thankful to the owner of this web site who has shared this fantastic piece of writing at at this place.
I have been browsing on-line more than three hours today, but I
by no means found any interesting article like yours.
It is pretty value sufficient for me. In my view, if all web owners
and bloggers made good content as you did, the net will be a lot more
useful than ever before.
It’s difficult to find well-informed people in this particular topic, but you seem like you know what you’re talking
about! Thanks
It’s impressive that you are getting thoughts from this article as well as
from our dialogue made at this time.
Feel free to visit my webpage :: หลักสูตรดำน้ำ
I am regular reader, how are you everybody? This article posted at
this website is truly good.
Wow, fantastic blog format! How long have you
ever been blogging for? you make blogging glance easy.
The entire glance of your web site is excellent, let alone the content material!
Fantastic website. Lots of useful information here. I am sending it to several friends ans also sharing in delicious.
And obviously, thanks on your sweat!
Thank you, I’ve just been looking for information about this subject for a while and yours is the
greatest I have discovered so far. However,
what in regards to the bottom line? Are you certain concerning the source?
Heya! I just wanted to ask if you ever have any problems with
hackers? My last blog (wordpress) was hacked and I ended up losing several weeks of hard work due to no back up.
Do you have any methods to prevent hackers?
I’m not that much of a online reader to be honest
but your blogs really nice, keep it up! I’ll go ahead and bookmark your website to
come back later. Many thanks
It’s not my first time to visit this site, i am visiting this site dailly and get
nice information from here daily.
I’m impressed, I must say. Seldom do I encounter a blog that’s both equally educative and
interesting, and let me tell you, you’ve hit the nail on the head.
The issue is an issue that too few people are speaking intelligently about.
I’m very happy that I came across this in my search for something concerning this.
Wow, this piece of writing is nice, my younger sister is analyzing these kinds of things, so I am going to convey
her.
Informative article, exactly what I needed.
Good answer back in return of this issue with firm arguments and telling the whole thing regarding that.
hey there and thank you for your information – I have certainly picked up anything new from right here.
I did however expertise some technical points using this site, since I experienced to reload the site lots of times previous to I could
get it to load correctly. I had been wondering if your web
host is OK? Not that I am complaining, but sluggish loading instances times will often affect your placement in google and
could damage your quality score if ads and marketing with Adwords.
Well I’m adding this RSS to my e-mail and could look out for much
more of your respective interesting content.
Ensure that you update this again very soon.
Thanks for sharing your info. I truly appreciate your efforts and I will be waiting
for your next write ups thanks once again.
Keep on writing, great job!
Right away I am ready to do my breakfast, after having my breakfast coming over again to read other news.
What a data of un-ambiguity and preserveness of precious
knowledge on the topic of unpredicted feelings.
agen138 agen138 agen138
Hi! Do you know if they make any plugins to assist with Search
Engine Optimization? I’m trying to get my blog to rank for some targeted keywords but I’m not seeing very good gains.
If you know of any please share. Cheers!
Hi! This post could not be written any better!
Reading this post reminds me of my previous room mate!
He always kept talking about this. I will forward this article to him.
Fairly certain he will have a good read. Many
thanks for sharing!
bookmarked!!, I love your website!
What’s up mates, good paragraph and pleasant urging commented here,
I am genuinely enjoying by these.
At this time it appears like Expression Engine is the top
blogging platform out there right now. (from what I’ve read) Is
that what you’re using on your blog?
I have fun with, cause I found just what I used to be having a look for.
You have ended my four day long hunt! God Bless you man. Have
a great day. Bye
What’s up mates, how is all, and what you want to say regarding this post, in my
view its really remarkable in support of me.
Thank you, I’ve recently been looking for info approximately this subject for a long time
and yours is the best I have came upon till now.
But, what about the bottom line? Are you positive in regards to the
supply?
I blog often and I truly thank you for your content. This great
article has really peaked my interest. I’m going to book mark your website and keep checking for
new information about once per week. I opted in for your RSS
feed too.
My brother recommended I might like this blog. He was totally right.
This post actually made my day. You can not imagine just how
much time I had spent for this info! Thanks!
Your style is really unique in comparison to
other folks I’ve read stuff from. I appreciate you for posting when you have
the opportunity, Guess I will just bookmark this page.
خرید استخر بادی اینتکس کودک و بزرگ
ارزان قیمت
اینتکس ایران
Hello Dear, are you in fact visiting this site on a regular basis,
if so after that you will without doubt get pleasant experience.
Feel free to visit my web blog – คอร์สเรียนดำน้ำลึก
Please let me know if you’re looking for a author for your blog.
You have some really great articles and I feel I would
be a good asset. If you ever want to take some of the load off,
I’d absolutely love to write some content for your blog in exchange
for a link back to mine. Please shoot me an e-mail if
interested. Thanks!
You have made some really good points there. I checked on the net for more information about the issue and found
most people will go along with your views on this web site.
Attractive component of content. I simply stumbled upon your blog and in accession capital to assert that I get actually loved account
your blog posts. Anyway I’ll be subscribing in your feeds and even I
success you get entry to persistently rapidly.
Blue Techker naturally like your web site however you need to take a look at the spelling on several of your posts. A number of them are rife with spelling problems and I find it very bothersome to tell the truth on the other hand I will surely come again again.
Thank you, I’ve just been looking for info approximately this topic for ages and
yours is the greatest I have came upon till
now. But, what about the bottom line? Are you positive concerning the supply?
Do you mind if I quote a couple of your articles as long as I provide credit and sources back to your site?
My blog site is in the exact same area of interest as yours and my users would genuinely benefit from
some of the information you present here. Please let me know if this
okay with you. Cheers!
Great post. I was checking constantly this weblog and I am inspired!
Extremely useful information specially the final part 🙂 I care for such information much.
I was seeking this particular information for a long time.
Thanks and good luck.
Someone necessarily assist to make severely articles I would
state. This is the very first time I frequented your website page and thus
far? I surprised with the analysis you made to make this actual publish incredible.
Wonderful task!
Hey there! I know this is kinda off topic but I’d figured I’d ask.
Would you be interested in trading links or maybe guest
authoring a blog post or vice-versa? My site covers a lot of the same subjects as yours and I
feel we could greatly benefit from each other. If you are interested feel
free to shoot me an email. I look forward to hearing from
you! Awesome blog by the way!
Hello everyone, it’s my first pay a visit at this web
page, and article is actually fruitful for me, keep
up posting these types of content.
If some one wishes to be updated with most recent technologies afterward he must
be go to see this web page and be up to date all the time.
Hello! I realize this is kind of off-topic but I needed
to ask. Does running a well-established website
like yours take a large amount of work? I’m
completely new to writing a blog but I do write in my diary daily.
I’d like to start a blog so I can easily share my own experience and views online.
Please let me know if you have any ideas or tips for new aspiring bloggers.
Appreciate it!
Nice post. I was checking continuously this blog and I
am impressed! Very helpful information particularly the
last part 🙂 I care for such information a lot. I was seeking this particular info for a
very long time. Thank you and best of luck.
Thinker Pedia I appreciate you sharing this blog post. Thanks Again. Cool.
I always used to read paragraph in news papers but now as I
am a user of web thus from now I am using net for posts, thanks to web.
This is my first time pay a quick visit at here and i
am actually pleassant to read everthing at alone place.
Jinx Manga I truly appreciate your technique of writing a blog. I added it to my bookmark site list and will
It’s hard to find well-informed people for this subject,
but you sound like you know what you’re talking about!
Thanks
hi!,I really like your writing very much! percentage we keep up a correspondence more
approximately your article on AOL? I require a specialist in this house to resolve my
problem. Maybe that is you! Looking ahead to peer you.
Hey I know this is off topic but I was wondering if you knew
of any widgets I could add to my blog that automatically
tweet my newest twitter updates. I’ve been looking for a plug-in like this for quite
some time and was hoping maybe you would have some
experience with something like this. Please let me know if you run into anything.
I truly enjoy reading your blog and I look forward to your new
updates.
Feel free to surf to my page … คอร์สเรียนดำน้ำลึก
I pay a quick visit every day some web sites and websites to read posts, except this web
site presents feature based writing.
It’s awesome to go to see this web page and reading the views
of all colleagues concerning this post, while I am also zealous of getting experience.
Feel free to visit my webpage: Smith Wesson MP 15-22 Sport Rimfire Rifle
Hello, I log on to your blogs daily. Your writing style is awesome, keep it up!
What’s up to all, it’s truly a fastidious for me to go to see this web
site, it consists of useful Information.
Heya are using WordPress for your blog platform?
I’m new to the blog world but I’m trying to get started and create my own. Do you need any html coding
expertise to make your own blog? Any help would be really
appreciated!
FinTech ZoomUs Hi there to all, for the reason that I am genuinely keen of reading this website’s post to be updated on a regular basis. It carries pleasant stuff.
Heya i’m for the first time here. I came across this board and I find It truly useful & it helped me out much.
I hope to give something back and help others like you helped me.
You’ve made some really good points there. I looked on the web to learn more about the issue and found most people will go along with your views
on this web site.
My brother recommended I may like this website.
He was totally right. This post truly made my day.
You cann’t believe simply how a lot time I had spent for this info!
Thank you!
If you would like to grow your knowledge simply keep visiting
this website and be updated with the newest news update
posted here.
Outstanding quest there. What happened after? Take care!
Hurrah, that’s what I was seeking for, what a material!
present here at this webpage, thanks admin of this web site.
Hi there, I do think your blog could be having internet browser compatibility issues.
When I take a look at your website in Safari, it looks
fine however when opening in I.E., it’s got some overlapping issues.
I simply wanted to provide you with a quick heads up!
Other than that, fantastic site!
Just wish to say your article is as amazing.
The clearness in your post is simply excellent and
i can assume you’re an expert on this subject. Fine with your permission allow me to grab
your RSS feed to keep updated with forthcoming post. Thanks a million and please continue the gratifying work.
You’re so awesome! I do not suppose I have read through
a single thing like that before. So nice to find someone with genuine thoughts on this
topic. Seriously.. thanks for starting this up.
This web site is one thing that is needed on the web, someone with a
little originality!
I just like the valuable information you supply for your articles.
I will bookmark your weblog and take a look at once more right here regularly.
I am relatively sure I will learn lots of new stuff proper right here!
Best of luck for the next!
My web page; หลักสูตรดำน้ำ
My brother suggested I may like this web site.
He was totally right. This post truly made my day. You can not consider just how
so much time I had spent for this information! Thanks!
I for all time emailed this weblog post page to all my
contacts, for the reason that if like to read it next my contacts will too.
It’s awesome designed for me to have a site, which is helpful designed for my know-how.
thanks admin
https://kchephoto.com/
What i do not understood is in reality how you are now not actually much more smartly-appreciated than you may
be right now. You are so intelligent. You already know
thus significantly in terms of this topic, produced me personally consider it from a lot of numerous angles.
Its like men and women are not fascinated until it is something to do with Girl gaga!
Your individual stuffs excellent. All the time maintain it up!
Hello, just wanted to say, I liked this
post. It was inspiring. Keep on posting!
I’ve been browsing on-line more than three hours today, but I by no
means found any interesting article like yours. It’s pretty
value sufficient for me. Personally, if all website owners and bloggers made excellent
content as you did, the net shall be much more useful than ever
before.
https://kchephoto.com/
Highly descriptive article, I liked that bit. Will there be a part 2?
I was suggested this web site by my cousin. I am not sure
whether this post is written by him as nobody else know such detailed about my trouble.
You’re amazing! Thanks!
What a data of un-ambiguity and preserveness of precious
knowledge on the topic of unexpected emotions.
Hi colleagues, its wonderful paragraph regarding tutoringand entirely explained, keep it up
all the time.
I’d like to thank you for the efforts you’ve put in writing this
website. I’m hoping to view the same high-grade blog posts from you later on as
well. In truth, your creative writing abilities has encouraged me to get my own, personal site now
😉
https://paitohkwarna.com/
Hello! I know this is kinda off topic nevertheless I’d figured I’d ask.
Would you be interested in trading links or maybe guest authoring a blog
article or vice-versa? My website goes over a lot of the same subjects as
yours and I feel we could greatly benefit from each
other. If you’re interested feel free to shoot me an email.
I look forward to hearing from you! Fantastic blog by the
way!
Here is my website :: คอร์สเรียนดำน้ำลึก
A person necessarily assist to make severely posts I’d state.
That is the very first time I frequented your website page and so far?
I amazed with the analysis you made to make this actual
put up amazing. Excellent task!
my homepage :: SEO Services
I just like the helpful info you provide in your articles.
I will bookmark your blog and test once more here
regularly. I am reasonably sure I will be informed many
new stuff proper here! Good luck for the next!
I blog often and I truly appreciate your content.
The article has truly peaked my interest. I’m going to take a note of your website and keep checking for new details about once per week.
I subscribed to your Feed too.
My brother suggested I might like this web site.
He was once totally right. This put up actually made my day.
You cann’t imagine just how so much time I
had spent for this info! Thanks!
This is the right web site for anybody who hopes to find out about this topic.
You understand so much its almost tough to argue with you (not
that I personally will need to…HaHa). You definitely put a fresh spin on a topic which
has been written about for many years. Great stuff, just excellent!
Ridiculous story there. What happened after? Take care!
Nice weblog right here! Also your website quite
a bit up fast! What host are you the use
of? Can I am getting your affiliate hyperlink for your host?
I want my website loaded up as quickly as yours lol
I couldn’t resist commenting. Exceptionally well
written!
Greetings from Florida! I’m bored to death at work so I decided to browse your website on my iphone during
lunch break. I enjoy the knowledge you provide here and can’t wait to take a look when I get home.
I’m shocked at how quick your blog loaded on my phone ..
I’m not even using WIFI, just 3G .. Anyways, good site!
This paragraph will assist the internet visitors for setting
up new web site or even a blog from start to end.
My partner and I stumbled over here from a different web page
and thought I might as well check things out. I like what I see so now i am following you.
Look forward to going over your web page repeatedly.
Excellent way of describing, and fastidious article to
obtain data regarding my presentation topic, which i am going to present in college.
It’s going to be end of mine day, but before ending I am reading this wonderful
article to improve my know-how.
An impressive share! I’ve just forwarded this onto a friend who was
conducting a little homework on this. And he actually ordered me
breakfast because I discovered it for him… lol. So
allow me to reword this…. Thanks for the meal!! But yeah, thanks for
spending time to talk about this issue here on your internet site.
You’ve made some decent points there. I checked on the net to find out more
about the issue and found most people will go along
with your views on this site.
Hurrah! Finally I got a website from where I can genuinely obtain helpful information regarding my study and knowledge.
Thanks for sharing your thoughts about สมัครSAGAME66.
Regards
If some one wishes to be updated with most recent technologies therefore he must
be visit this site and be up to date every day.
Inspiring quest there. What occurred after?
Good luck!
Stop by my web page – Pinoy SEO Services
Have you ever considered writing an e-book or guest authoring on other websites?
I have a blog based upon on the same information you discuss and would love
to have you share some stories/information. I know
my subscribers would enjoy your work. If you’re even remotely interested, feel free to send me an e-mail.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
I every time emailed this webpage post page to all my contacts, because if
like to read it afterward my friends will too.
Hello, all the time i used to check blog posts here early in the dawn,
as i like to learn more and more.
Also visit my homepage – ถ่ายพรีเวดดิ้ง
Hello there, I discovered your web site
by way of Google at the same time as searching for a similar
matter, your website got here up, it appears to be like good.
I’ve bookmarked it in my google bookmarks.
Hi there, simply changed into aware of your weblog through Google, and located that it is really informative.
I am gonna watch out for brussels. I will appreciate for those who continue this in future.
A lot of folks will probably be benefited from your writing.
Cheers!
Hi, i think that i saw you visited my blog thus i came to “return the favor”.I’m
trying to find things to improve my web site!I suppose its
ok to use a few of your ideas!!
Hello! I simply wish to give you a huge thumbs up for the great information you have here
on this post. I will be returning to your blog for more soon.
Ahaa, its pleasant discussion on the topic of this piece
of writing at this place at this website, I have read all that, so now me also commenting at this place.
Appreciate this post. Will try it out.
My web page; ถ่ายพรีเวดดิ้ง
Nice post. I learn something new and challenging on websites I stumbleupon everyday.
It’s always interesting to read through articles from other writers and
use a little something from other web sites.
Your style is really unique compared to other folks
I’ve read stuff from. Thank you for posting when you have the opportunity, Guess I’ll just
bookmark this web site.
At this time it appears like WordPress is the top blogging platform available
right now. (from what I’ve read) Is that what you are using on your blog?
Yes! Finally something about SAบาคาร่า.
I’ve been browsing online more than 2 hours today, yet I never found any interesting
article like yours. It is pretty worth enough for me.
In my view, if all website owners and bloggers made good content as you did, the net will be much more useful than ever before.
I’m really impressed together with your writing skills and
also with the layout for your weblog. Is this a paid subject
or did you customize it yourself? Either way stay up the nice quality writing, it is uncommon to look a great blog like this one nowadays..
Great article. I am experiencing some of these issues as well..
Your article helped me a lot, is there any more related content? Thanks!
Greetings! Very helpful advice within this post!
It’s the little changes that will make the greatest changes.
Many thanks for sharing!
My brother suggested I may like this website. He was entirely right.
This submit truly made my day. You cann’t believe simply how so much time I had spent for this info!
Thanks!
At this moment I am going away to do my breakfast, after having my breakfast coming over again to read further news.
It’s difficult to find educated people in this particular subject, but you seem
like you know what you’re talking about! Thanks
For the reason that the admin of this website is working, no doubt
very shortly it will be famous, due to its quality contents.
Hi! This is kind of off topic but I need some guidance
from an established blog. Is it hard to set up your own blog?
I’m not very techincal but I can figure things out pretty
fast. I’m thinking about setting up my own but I’m not sure where to start.
Do you have any tips or suggestions? Thanks
Hello! I know this is somewhat off topic but I was wondering which blog platform are you using for this site?
I’m getting tired of WordPress because I’ve had issues with hackers
and I’m looking at alternatives for another
platform. I would be great if you could point me in the direction of a good platform.
Link exchange is nothing else however it is simply placing the other person’s blog link on your page at
suitable place and other person will also do similar in favor
of you.
https://kchephoto.com/
I’ve been surfing online more than 3 hours today, yet I never found any interesting article like
yours. It is pretty worth enough for me. In my view, if
all web owners and bloggers made good content as you
did, the internet will be much more useful than ever before.
Wow, this article is pleasant, my younger sister is
analyzing these kinds of things, therefore I am
going to inform her.
My web-site ถ่ายพรีเวดดิ้ง
Great post.
Why visitors still make use of to read news papers when in this technological globe everything is
presented on web?
Wow, this piece of writing is pleasant, my younger sister is
analyzing these kinds of things, so I am going to tell her.
With havin so much content do you ever run into any issues
of plagorism or copyright violation? My website has a lot
of completely unique content I’ve either authored
myself or outsourced but it appears a lot of it is popping it up all over the
internet without my authorization. Do you know any techniques to help stop content from being stolen? I’d definitely appreciate it.
I visited various websites but the audio feature for audio songs existing at this website is really excellent.
Also visit my web blog – web page
I absolutely love your blog.. Great colors & theme.
Did you create this site yourself? Please reply
back as I’m attempting to create my own personal website and would like to know where you got this from or what the theme is named.
Kudos!
Just want to say your article is as astonishing. The clearness
in your post is simply nice and i can assume you are an expert on this subject.
Well with your permission let me to grab your feed
to keep up to date with forthcoming post. Thanks a million and please carry on the gratifying work.
Hello! I could have sworn I’ve been to this blog before but after browsing through some of the post I
realized it’s new to me. Anyways, I’m definitely delighted I found it and I’ll be book-marking and checking back frequently!
Fantastic beat ! I would like to apprentice while you amend your site,
how could i subscribe for a blog site? The account aided me a acceptable deal.
I had been a little bit acquainted of this your broadcast provided bright clear concept
I quite like reading through an article that will make people think.
Also, thank you for allowing for me to comment!
Touche. Solid arguments. Keep up the great effort.
I was able to find good info from your content.
Hello! I understand this is kind of off-topic however
I had to ask. Does operating a well-established website such as
yours require a lot of work? I’m brand new to blogging but I
do write in my diary everyday. I’d like to start a blog so I will be able to
share my own experience and views online. Please let me know if you have any recommendations or tips for new aspiring bloggers.
Thankyou!
I delight in, lead to I discovered exactly what I was
taking a look for. You have ended my 4 day long hunt!
God Bless you man. Have a nice day. Bye