In this Python tutorial, we will learn How to Make a Dog Fae Using Python Turtle. In this tutorial, we split the code and explain How we can Make a Dog Face by using Python Turtle.
How to Make a Dog Face Using Python Turtle
Now start this project, firstly importing the turtle library such as import turtle as tur.
# How to Make a Dog Face Using Python Turtle
#Imported turtle
import turtle as tur
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
After importing the turtle library we are creating an object for this turtle and also give a title to the screen.
#set the turtle object
turt=tur.Turtle()
tur.title("Pythontpoint")
screen=turt.getscreen()
screen.bgcolor("light yellow")
After that, we are using the function to create the face of the dog. For creating the face, we have to use the following functions.
- turt.pencolor(“tan1”) is used as pen color.
- turt.pensize(3) is used to give the size of the pen.
- turt.goto(30,-30) is used to move the turtle at its accurate position.
- turt.pendown() is used to start drawing on the screen with the help of the turtle.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.circle(45,180) is used to draw the circle on the screen.
- turt.right(90) is used to move the turtle in the right direction.
- turt.forward(55) is used to move the turtle in the forward direction.
- turt.penup() is used to stop the drawing.
- turt.end_fill() is used to stop filling the color in the shape.
# How to Make a Dog Face Using Python Turtle
#Drawing the face of the dog
turt.pencolor("tan1")
turt.color("tan1")
turt.pensize(3)
turt.penup()
turt.setheading(90)
turt.goto(30,-30)
turt.pendown()
turt.begin_fill()
turt.circle(45,180)
turt.right(90)
turt.goto(-45,-30)
turt.circle(35,190)
turt.setheading(0)
turt.forward(55)
turt.penup()
turt.setheading(0)
turt.pendown()
turt.circle(35,170)
turt.penup()
turt.end_fill()
After that, we are using the function to create the tongue of the dog. For creating the tongue we are using the following function.
- turt.pencolor(“Deeppink1”) is used to give the color to the pen.
- turt.goto(-10,-90) is used to move the turtle at its accurate position.
- turt.right(60) is used to move the turtle in the right direction.
- turt.pendown() is used to start the drawing on the screen.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.forward(15) is used to move the turtle in the forward direction.
- turt.left(60) is used to move the turtle in the left direction.
- turt.end_fill() is used to stop filling color.
#Create the tongue of the dog
turt.pencolor("Deeppink1")
turt.color("pink")
turt.setheading(270)
turt.goto(-10,-90)
turt.right(60)
turt.pendown()
turt.begin_fill()
turt.forward(15)
turt.left(60)
turt.forward(10)
turt.circle(10,180)
turt.forward(10)
turt.left(60)
turt.forward(15)
turt.end_fill()
Now we are using the function to make the line on the tongue. For drawing the line on the tongue we are using the following function.
- turt.pensize(1) is used to give the size of the pen.
- turt.pencolor(“DeepPink”) is used to give the color to the pen.
- turt.goto(-13,-90) is used to move the turtle at its accurate position.
- turt.forward(17) is used to move the turtle in the forward direction.
- turt.circle(4,180) is used to make the circle by using a turtle.
- turt.left(90) is used to move the turtle in the left direction.
- turt.pendown() is used to start the drawing on the screen.
#line on the tongue
turt.pensize(1)
turt.pencolor("DeepPink")
turt.goto(-13,-90)
turt.setheading(270)
turt.forward(17)
turt.circle(4,180)
turt.penup()
turt.left(90)
turt.forward(8)
turt.goto(-13,-107)
turt.setheading(270)
turt.left(180)
turt.pendown()
turt.circle(4,-180)
Now we’re using the function to create the right and left ears of the dog. For drawing the ears we are using the following function.
- turt.penup() is used to stop the drawing.
- turt.pencolor(“black”) is used to give the color to the pen.
- turt.pendown() is used to start the drawing on the screen.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.forward(10) is used to move the turtle in the forward direction.
- turt.left(60) is used to move the turtle in the left direction.
- turt.circle(35,145) is used to draw the circle.
- turt.end_fill() is used to stop filling color in the shape.
#right ear
turt.penup()
turt.goto(42,-45)
turt.pencolor("black")
turt.color("sienna")
turt.setheading(0)
turt.pendown()
turt.begin_fill()
turt.forward(10)
turt.left(60)
turt.circle(35,145)
turt.end_fill()
#left ear
turt.penup()
turt.goto(-70,-45)
turt.pendown()
turt.setheading(180)
turt.begin_fill()
turt.forward(10)
turt.left(-240)
turt.circle(35,-145)
turt.end_fill()
turt.penup()
After that, we are using the function to make the eyes of the dog. For drawing the eyes we are using the following function.
- turt.color(“black”) is used to give color to the shape.
- turt.pendown() is used to start the drawing on the screen.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.circle(10,180) is used to draw the shep of the circle.
- turt.forward(5) is used to move the turtle in the forward direction.
- turt.end_fill() is used to stop filling color in the shape.
- turt.penup() is used to stop the drawing.
# How to Make a Dog Face Using Python Turtle
#eyes
turt.pencolor("black")
turt.color("black")
turt.goto(-5,-45)
turt.setheading(270)
turt.pendown()
turt.begin_fill()
turt.circle(10,180)
turt.forward(5)
turt.circle(10,180)
turt.forward(5)
turt.end_fill()
turt.penup()
turt.pencolor("black")
turt.color("black")
turt.goto(-45,-45)
turt.setheading(270)
turt.pendown()
turt.begin_fill()
turt.circle(10,180)
turt.forward(5)
turt.circle(10,180)
turt.forward(5)
turt.end_fill()
turt.penup()
Now, we are using the function to make the inner white dots in the eyes of the dog. For drawing the inner white dots in the eyes we are using the following function.
- turt.color(“white”) is used to give the white color to the dots.
- turt.goto(-2,-44) is used to move the turtle to its accurate position.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.circle(6) is used to draw the shape of the circle.
# Inner white dots in the eye
turt.color("white")
turt.goto(-2,-44)
turt.begin_fill()
turt.circle(6)
turt.end_fill()
turt.color("white")
turt.goto(-40,-44)
turt.begin_fill()
turt.circle(6)
turt.end_fill()
Now, we are using the function to make the nose of the dog. For drawing the nose we are using the following function.
- turt.color(“black”) is used to give the black color to the nose.
- turt.goto(-25,-75) is used to move the turtle at its accurate position.
- turt.begin_fill() is used to start filling the color in the shape.
- turt.circle(2) is used to draw the shape of the circle.
- turt.hideturtle() is used to hide the turtle on the screen.
# How to Make a Dog Face Using Python Turtle
#Nose of the dog
turt.color("black")
turt.goto(-25,-75)
turt.begin_fill()
turt.circle(10)
turt.end_fill()
turt.goto(-14,-73)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.goto(-20,-73)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.goto(-17,-77)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.hideturtle()
tur.done()
After splitting the code and explaining How to Make a Dog Face Using Python Turtle. Now we will see how the output looks like after running the whole code.
# How to Make a Dog Face Using Python Turtle
# How to Make a Dog Face Using Python Turtle
#Imported turtle
import turtle as tur
#set the turtle object
turt=tur.Turtle()
tur.title("Pythontpoint")
screen=turt.getscreen()
screen.bgcolor("light yellow")
#Drawing the face of the dog
turt.pencolor("tan1")
turt.color("tan1")
turt.pensize(3)
turt.penup()
turt.setheading(90)
turt.goto(30,-30)
turt.pendown()
turt.begin_fill()
turt.circle(45,180)
turt.right(90)
turt.goto(-45,-30)
turt.circle(35,190)
turt.setheading(0)
turt.forward(55)
turt.penup()
turt.setheading(0)
turt.pendown()
turt.circle(35,170)
turt.penup()
turt.end_fill()
#Create the tongue of the dog
turt.pencolor("Deeppink1")
turt.color("pink")
turt.setheading(270)
turt.goto(-10,-90)
turt.right(60)
turt.pendown()
turt.begin_fill()
turt.forward(15)
turt.left(60)
turt.forward(10)
turt.circle(10,180)
turt.forward(10)
turt.left(60)
turt.forward(15)
turt.end_fill()
#line on the tongue
turt.pensize(1)
turt.pencolor("DeepPink")
turt.goto(-13,-90)
turt.setheading(270)
turt.forward(17)
turt.circle(4,180)
turt.penup()
turt.left(90)
turt.forward(8)
turt.goto(-13,-107)
turt.setheading(270)
turt.left(180)
turt.pendown()
turt.circle(4,-180)
#right ear
turt.penup()
turt.goto(42,-45)
turt.pencolor("black")
turt.color("sienna")
turt.setheading(0)
turt.pendown()
turt.begin_fill()
turt.forward(10)
turt.left(60)
turt.circle(35,145)
turt.end_fill()
#left ear
turt.penup()
turt.goto(-70,-45)
turt.pendown()
turt.setheading(180)
turt.begin_fill()
turt.forward(10)
turt.left(-240)
turt.circle(35,-145)
turt.end_fill()
turt.penup()
#eyes
turt.pencolor("black")
turt.color("black")
turt.goto(-5,-45)
turt.setheading(270)
turt.pendown()
turt.begin_fill()
turt.circle(10,180)
turt.forward(5)
turt.circle(10,180)
turt.forward(5)
turt.end_fill()
turt.penup()
turt.pencolor("black")
turt.color("black")
turt.goto(-45,-45)
turt.setheading(270)
turt.pendown()
turt.begin_fill()
turt.circle(10,180)
turt.forward(5)
turt.circle(10,180)
turt.forward(5)
turt.end_fill()
turt.penup()
# Inner white dots in the eye
turt.color("white")
turt.goto(-2,-44)
turt.begin_fill()
turt.circle(6)
turt.end_fill()
turt.color("white")
turt.goto(-40,-44)
turt.begin_fill()
turt.circle(6)
turt.end_fill()
#Nose of the dog
turt.color("black")
turt.goto(-25,-75)
turt.begin_fill()
turt.circle(10)
turt.end_fill()
turt.goto(-14,-73)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.goto(-20,-73)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.goto(-17,-77)
turt.color("white")
turt.begin_fill()
turt.circle(2)
turt.end_fill()
turt.hideturtle()
tur.done()
After running the above code we get the following output in which we can see that the face of the dog is beautifully drawn on the screen.

So, in this tutorial, we have illustrated How to Make a Dog Face Using Python Turtle. Moreover, we have also discussed the whole code used in this tutorial.
Read some more tutorials related to Python Turtle.
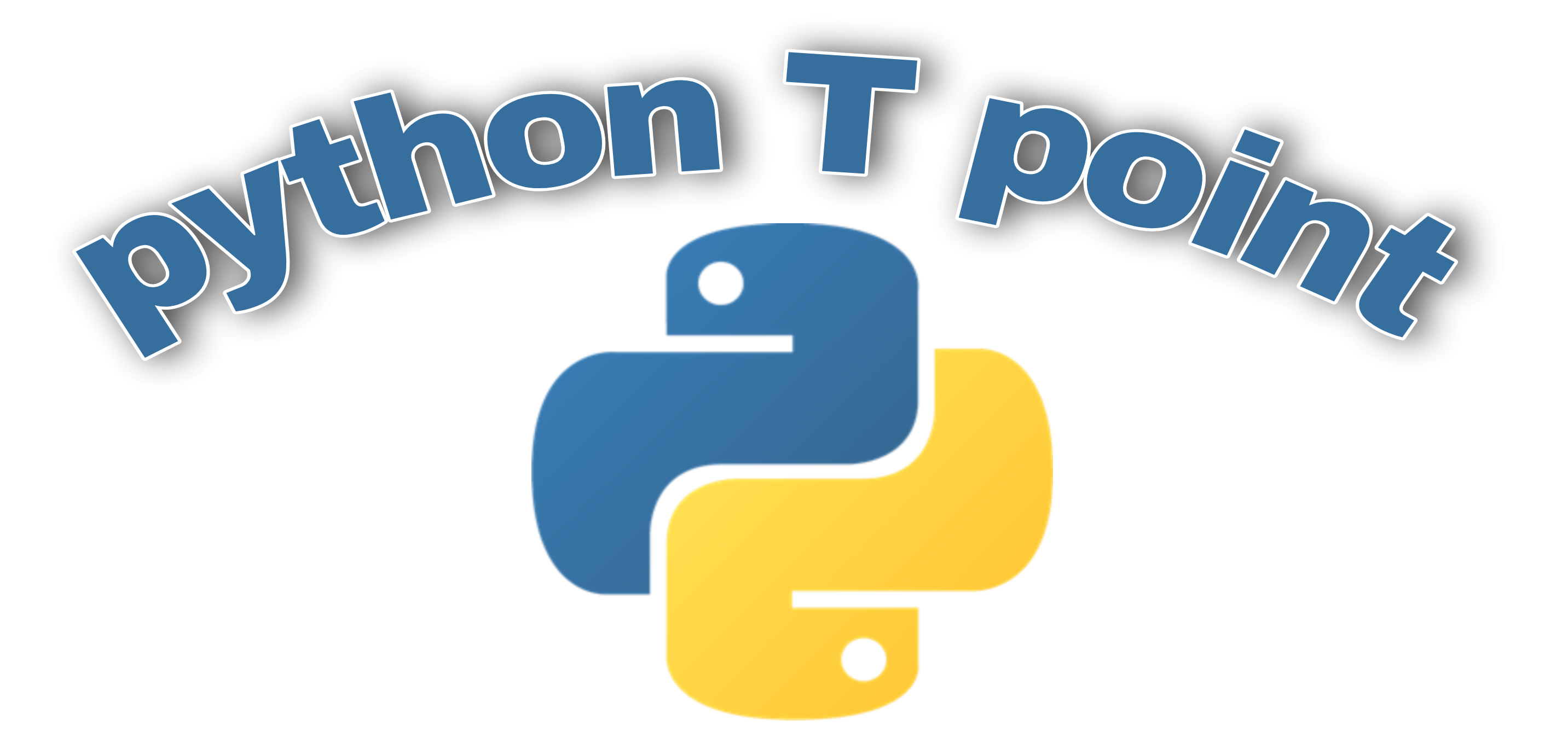
4 thoughts on “How to Make a Dog Face Using Python Turtle”
Comments are closed.