In this tutorial, we will learn about how to work with data types in Python and we will also cover different examples related to Python Datatypes. And, we will cover these topics
- Python Datatypes
- Python Standard Datatypes
- Python Sequence Datatypes
Python Datatypes
Python is a simple programming language and we use this language in our daily life which makes our life simpler and easier. As we know there are many rules that we follow in our daily life Python also have some rule that we have to follow one of the main rules is a datatype which makes our work simpler.
Datatype is a type of data that informs the compiler or interprets how to deal with data or how the programmer can use the data. The datatype can support the numbers, characters, and booleans.
Python has the following build-in datatypes:
- Text types: str
- Sequence types: int,float
- Numeric types: list,tuples,range
- Set types: set,frozenset
- Boolean types: bool
- Binary types: bytes,bytearray
Code:
In the following code, we will print the value of the data type of variable i. Here we give the value to the variable “i ” as 10 and want the datatype of the variable “i” to be printed on the command prompt.
i = 10
print(type(i))
Output:
After running the above code we get the following output in which we can see that the data type of variable i is printed on the command prompt.

Python Standard Datatypes
As we know data type is a type of data that informs the compiler or interpreter how to deal with data in which form data can be easily run. The standard data type is the data type that is used to define the operation and the storage method.
Python has five data types:
- Numeric
- Sequence
- Bolean
- Set
- Dictionary
Numeric
In Python, the numeric data type is defined as a data type that shows the data has a numeric value. The numeric value is in the form of an integer, floating number, or complex number. These values are represented as int, float, and complex classes in python.
The numeric data type is further divided into three parts:
- Integer
- Float
- Complex Numbers
Integer
In Python integer value is represented as int class. It contains positive and negative whole numbers. The integer value has no limit to how long it can be in python.
Example:
a1 = 5
print("Type of a1: ", type(a1))
Output:
After running the above code we get the following output in which we can see that the type of a1 is printing on the command prompt.

Float
In python, float value is represented by float class. It consists of a real number and is represented by a floating point. It is defined by a decimal point.
Example
b1 = 7.0
print("Type of b1: ", type(b1))
Output:
After running the above code we get the following output in which we can see the type of b1 is printed on the command prompt.

Complex Number
In python complex number is defined by the complex class. It is specified by the real and imaginary parts.
Example:
c1 = 5 + 9j
print("\nType of c1: ", type(c1))
Output:
After running the above code we get the following output in which we can see the type of c1 is printed on the command prompt.

Python Sequence Data type
The sequence data types are further divided into three parts:
- Strings
- Lists
- Tuples
Strings
In python, the string is recognized as a continuous set of characters which is represented with the help of quotation marks(” “). Python string allows both single and double quotes and we can also take the subset of strings by simply using the slice operator( [] ) where its starting index is 0 in the beginning and -1 is the ending index.
Example:
In this example, we can create a string using single quotes, double quotes, and triple quotes.
- Strng = ‘Welcome to the PythonTpoint World’ is used to create a string with single quotes.
- print(Strng) is used to print the string after creating.
- Strng = “Heya I am a PythonTpoint Guide” is used to create a string with double-quotes.
- print(type(Strng)) is used to print the string after creating.
- Strng = ”’I’m a PythonTpoint helpful Guide and I live in a world of “PythonTpoint””’ is used to create a string with triple quotes.
Strng = 'Welcome to the PythonTpoint World'
print("String with the use of Single Quotes: ")
print(Strng)
Strng = "Heya I am a PythonTpoint Guide"
print("\nString with the use of Double Quotes: ")
print(Strng)
print(type(Strng))
Strng = '''I'm a PythonTpoint helpful Guide and I live in a world of "PythonTpoint"'''
print("\nString with the use of Triple Quotes: ")
print(Strng)
print(type(Strng))
Strng = '''PythonTpoint
For
Learner'''
print("\nCreating a multiline String: ")
print(Strng)
Output:
After running the above code we get the following output in which we can see that the string with the use of single, double, and triple quotes is printed on the command prompt.

Lists
In python, the list is the most versatile compound data type which contains items that are separated by commas and enclosed in square braces ( [] ).
Example:
In this example, we are creating a list. This list contains items that are separated by commas and enclosed in square braces.
- list = [“apple”, “banana”, “cherry”] this is the list which contain the useful items.
- print(list) is used to print the list on the command prompt.
list = ["apple", "banana", "cherry"]
print(list)
Output:
After running the above code we get the following output in which we can see that the list which contains items that are separated by commas and enclosed in a square bracket is printing on the command prompt.

Tuples
A tuple is also a data type that is similar to the list. A tuple is defined as which consists of values that are separated by commas. Tuples are enclosed within parentheses.
Example:
In the following example firstly we are creating an empty tuple after creating printing this empty tuple on the screen.
- Tuple = (‘Python’, ‘T’,’Point’) creating a tuple with the use of string.
- list = [1, 2, 4, 5, 6] creating a list with the use of the list.
Tuple = ()
print("Initial empty Tuple: ")
print (Tuple)
Tuple = ('Python', 'T','Point')
print("Tuple with the use of String: ")
print(Tuple)
list = [1, 2, 4, 5, 6]
print("Tuple using List: ")
print(tuple(list))
Tuple = tuple('Pythontpoint')
print("Tuple use of function: ")
print(Tuple)
Tuple = (0, 1, 2, 3)
Tuple1 = ('python', 't','point')
Tuple2 = (Tuple, Tuple1)
print("Tuple with nested tuples: ")
print(Tuple2)
Output:
After running the above code we get the following output in which we can see the firstly empty turtle is created and then tuple with the use of string and after that tuple with the use of the function.

Boolean
In the Boolean data type, there are two built-in values True or False. They store the True or False data values as a single byte.
Example:
print(type(True))
print(type(False))
Output:
After running the above code we get the following output in which we can see the class of the data values.

Set
Set is defined as an unordered collection data type that is mutable and has no duplicate element. In Set, the order of an element is undefined and consists of the various elements.
Example:
In this example, we are creating a set after creating this set printing on the command prompt.
- set = set([“Python”, “t”, “point”]) creating a set.
- print(“Initial set”) is used to printing the initial set.
set = set(["Python", "t", "point"])
print("Initial set")
print(set)
# Accessing element using
# for loop
print("Elements of set: ")
for x in set:
print(x, end =" ")
# Checking the element
# using in keyword
print("Pythontpoint" in set)
Output:
After running the above code we get the following output in which we can see the initial set is printed on the screen.

Dictionary
Dictionary is an unordered collection of data values. It stores data value in the form of key-value pair and does not allow duplicated values.
Examples:
In this example, we will create a dictionary that stores data value in the form of key pair and does not allow duplicate values.
Dict = {1: 'Python', 'name': 't', 3: 'Point'}
print("Access an element using key:")
print(Dict[1])
print("Access an element using get:")
print(Dict.get(3))
Output:
After running the above code we get the following output in which we can see a dictionary is created and an element is accessed using a key.

So, in this tutorial, we discussed Python Datatypes and we have also covered different examples related to its implementation. Here is the list of examples that we have covered.
- Python Datatypes
- Standard Datatypes
- Sequence Datatype
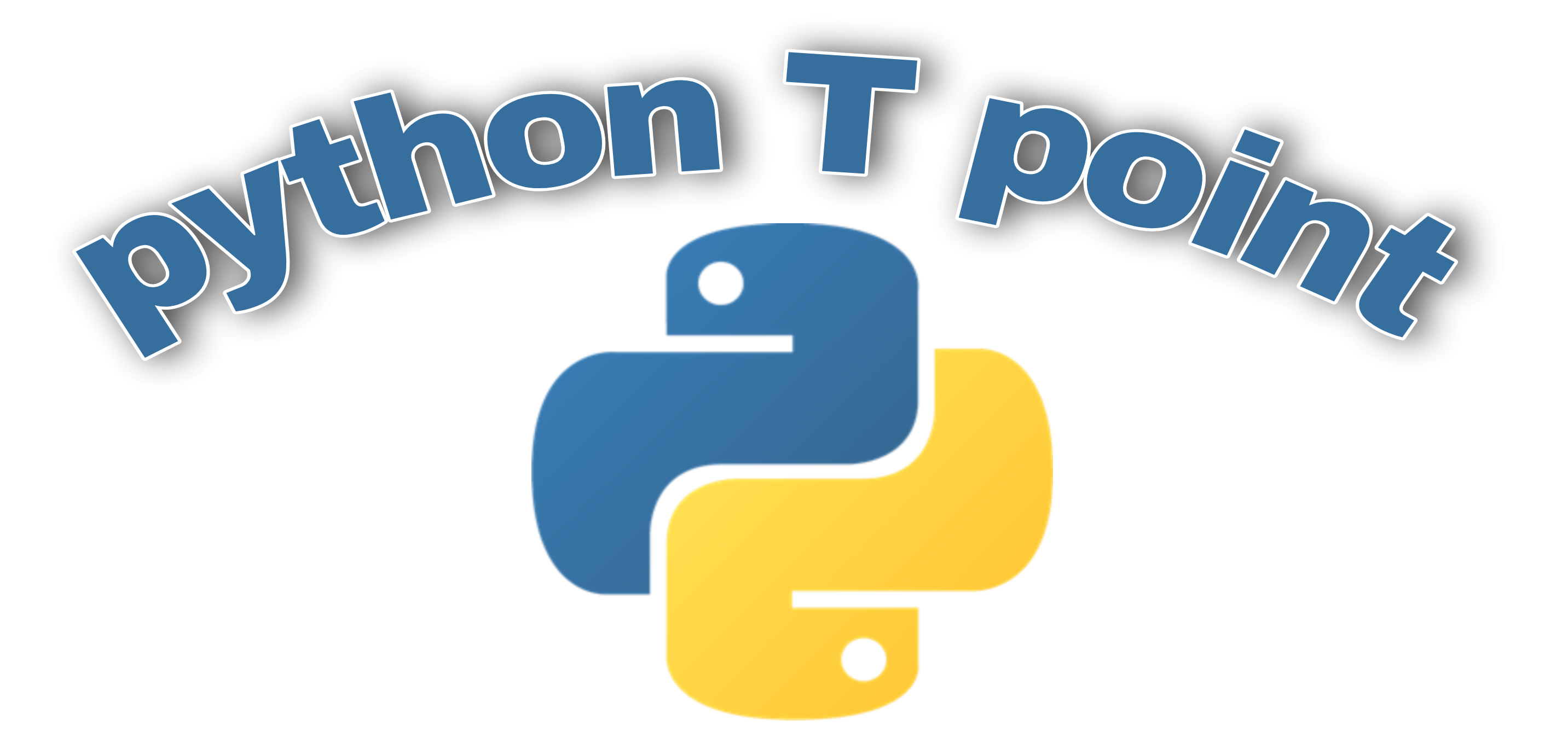
1 thought on “Python Datatypes”
Comments are closed.