In this Python tutorial, we will learn about Python Dictionary and we will also covers different examples related to Python Dictionary. And we will also covers these topics.
- Python dictionary
- Create a dictionary in Python
- Adding element to a dictionary in Python
- Accessing element of dictionary in Python
- Removing element from a dictionary in Python
Python dictionary
In Python dictionary is used to store the values in key value pairs. The dictionary is also defines as a collection of ordered and changeable values. Dictionary are written with curly braces and separated by commas. The dictionary the value can be of any datatype and can be duplicated.
Example:
In the following example build a Python dictionary curly braces and separated by commas. Here we are written Pythontpoint in curly braces separated with commas.
Dic = {1: 'Python', 2: 'T', 3: 'Point'}
print(Dic)
Dic ={1: 'John', 2: 'Smith'}
print(Dic)
Output:
In the following output we can see that the dictionary with key value pair is printed on the screen by using print() function.
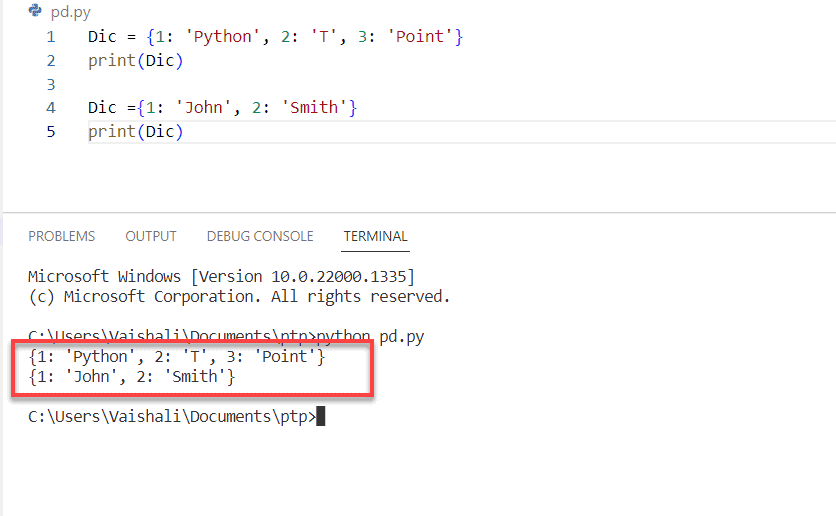
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Create a dictionary in Python
A dictionary in python can be created by placed an elements in the curly brackets and separated by commas. It also carry the pair of values as a key values. The value in dictionary can be duplicate but can not be repeatable.
Examples:
In the following example we are creating a dictionary with integer and mixed keys and also creating an empty dictionary and creating a dictionary with dict() method.
- Dic = {1: ‘Python’, 2: ‘T’, 3: ‘Point’} is used to create dictionary with integer keys.
- print(“\n With the use of Integer Keys create a dictionary: “) is used to print dictionary with the use of integer.
- Dic = {‘Name’: ‘Pythontpoint’, 1: [2, 4, 6, 8]} is used to create dictionary with mixed values.
- Dic = {} is used to create an empty dictionary.
- Dic = dict({1: ‘Python’, 2: ‘T’, 3: ‘Point’}) is used to create a dictionary with the help of dict() function.
# Create a Dictionary with Integer Keys
Dic = {1: 'Python', 2: 'T', 3: 'Point'}
print("\n With the use of Integer Keys create a dictionary: ")
print(Dic)
# Create a Dictionary with mixed keys
Dic = {'Name': 'Pythontpoint', 1: [2, 4, 6, 8]}
print("\n With the use of mixed Keys create dictionary: ")
print(Dic)
# Create an empty Dictionary
Dic = {}
print("Empty Dictionary: ")
print(Dic)
# Creating a Dictionary with dict() method
Dic = dict({1: 'Python', 2: 'T', 3: 'Point'})
print("\n With the use of dict() create dictionary: ")
print(Dic)
Output:
After running the above code we get the following output in which we can see that the dictionary is created with keys and dict() function.
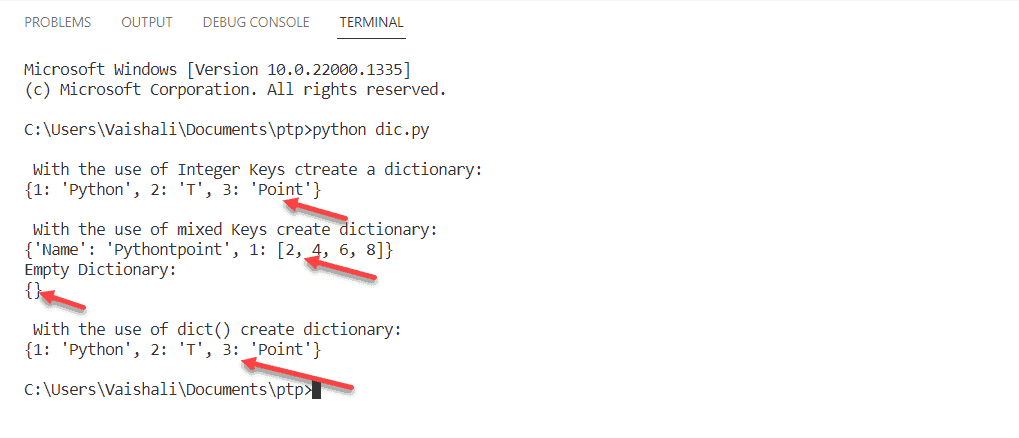
So, with this we have learned how to create a dictionary in python and further we will learn how to create a dictionary in python.
Read: Python List
Adding element to a dictionary in Python
We can also add the elements by different ways. The value can be added to the dictionary along with key and we can also added a nested value to a dictionary and also update a dictionary by using update() method.
Example:
In the following example we are adding the element to a dictionary in python. Firstly we are creating the empty dictionary the adding the elements to the dictionary one at a time after that add the set of values to a single key then update the existing key value after that adding the nested key value to dictionary.
# Create an empty Dictionary
Dic = {}
print("Empty Dictionary: ")
print(Dic)
# Adding elements one at a time
Dic[1] = 'Python'
Dic[2] = 'Tpoint'
print("\nDictionary after adding 2 elements: ")
print(Dic)
# Add the set of values to a single Key
Dic['setofvalues'] = 2, 4, 6
print("\nDictionary after adding 3 elements: ")
print(Dic)
# Update the existing Key's Value
Dic[1] = 'MOSTWELCOME'
print("\nUpdate the key value: ")
print(Dic)
# Adding Nested Key value to Dictionary
Dic[4] = {'Nested': {'0': 'Live', '1': 'Life'}}
print("\nAdd a Nested Key: ")
print(Dic)
Output:
After running the above code we get the following output in which we can see that the elements are added to the dictionary in python.

Read: Python Literals
Accessing element of dictionary in Python
For accessing the elements of a dictionary suggested to as its key values and the key values can be paced inside the square brackets.
Examples:
In the following example we are accessing the elements of dictionary in python by simply uses the key values and these key values are placed inside the square brackets.
# Python program to accessing an element from a Dictionary
access_dic = {121: "John", 122: "Smith", 123: "Keller"}
# prints the acccessing element
print(access_dic[121])
print(access_dic[123])
Output:
In this output, you can see that the values are accessing with the help of key value and printing on the screen.

Read: Python Strings
Removing element from a dictionary in Python
In Python we are using the del statement that remove an element from the dictionary. We are simply using del statement and the key value and those key value can be removed from the dictionary.
Example:
In the following example we are defining a dictionary and then print the original dictionary after that want to remove the element from the above dictionary by using del statement and then print the updated dictionary.
rm_studentid= {101: "Metairie", 102: "Joseph", 103: "Peggy"}
print("Initial Dictionary: ", rm_studentid)
del rm_studentid[102]
print("Updated Dictionary ", rm_studentid)
Output:
After running the above code we will get the following output in which we can see that the initial dictionary and the updated dictionary is printed on the screen.

So, in this tutorial, we have learned about the Python Dictionary and we have covered all these topics.
- Python dictionary
- Create a dictionary in Python
- Adding element to a dictionary in Python
- Accessing element of dictionary in Python
- Removing element from a dictionary in Python
Do follow the following tutorials also:

It was impossible for me to leave your website without expressing my gratitude for the excellent knowledge you give your visitors. Without a doubt, I’ll be checking back frequently to see what updates you’ve made.
It is actually a nice and useful piece of information. I am happy that you simply shared this helpful information with us. Please keep us up to date like this. Thank you for sharing.
Wow amazing blog layout How long have you been blogging for you made blogging look easy The overall look of your web site is magnificent as well as the content
Hi my loved one! I wish to say that this article is amazing, great written and come with almost all important infos. I’d like to peer extra posts like this .
I think you have noted some very interesting details , appreciate it for the post.
Thank you for the auspicious writeup. It in fact was a amusement account it. Look advanced to more added agreeable from you! However, how can we communicate?
There are actually a whole lot of particulars like that to take into consideration. That is a nice level to convey up. I supply the thoughts above as common inspiration but clearly there are questions just like the one you deliver up where the most important thing can be working in sincere good faith. I don?t know if greatest practices have emerged round things like that, however I am positive that your job is clearly identified as a fair game. Each girls and boys feel the impact of just a second’s pleasure, for the remainder of their lives.
You really make it seem really easy along with your presentation but I to find this matter to be actually something that I feel I might by no means understand. It kind of feels too complex and very extensive for me. I’m looking ahead for your next post, I’ll try to get the hold of it!
Hello Neat post Theres an issue together with your site in internet explorer would check this IE still is the marketplace chief and a large element of other folks will leave out your magnificent writing due to this problem
I think this is one of the most vital info for me. And i am glad reading your article. But wanna remark on few general things, The site style is great, the articles is really great : D. Good job, cheers
I really enjoy looking at on this web site, it contains wonderful content.
Wow wonderful blog layout How long have you been blogging for you make blogging look easy The overall look of your site is great as well as the content
My admiration for your creations is the same as your own opinion. The visual presentation is tasteful, while the written content is sophisticated. You’re skeptical, despite the fact that you are aware of it. I’m confident that you’ll be able to resolve this problem quickly and effectively.
I have recently started a web site, the information you offer on this website has helped me greatly. Thank you for all of your time & work.
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Great line up. We will be linking to this great article on our site. Keep up the good writing.
It is really a great and helpful piece of information. I am glad that you shared this useful info with us. Please stay us informed like this. Thanks for sharing.
We’re a group of volunteers and starting a new scheme in our community. Your web site provided us with valuable info to work on. You have done an impressive job and our entire community will be thankful to you.
I do love the manner in which you have framed this concern and it really does present me personally a lot of fodder for consideration. On the other hand, because of everything that I have seen, I really wish when the responses stack on that men and women continue to be on issue and not get started upon a soap box involving the news du jour. All the same, thank you for this superb piece and whilst I do not go along with the idea in totality, I value your perspective.
Your work has captivated me just as much as it has captivated you. The visual display is elegant, and the written content is impressive. Nevertheless, you seem concerned about the possibility of delivering something that may be viewed as dubious. I agree that you’ll be able to address this issue promptly.
Hi my family member! I wish to say that this post is amazing, great written and include almost all vital infos. I would like to peer more posts like this .
Normalmente eu não leio artigos em blogs, mas gostaria de dizer que este artigo me forçou a tentar fazê-lo. Seu estilo de escrita me surpreendeu. Obrigado, ótima postagem
Site fantástico Muitas informações úteis aqui, estou enviando para alguns amigos e também compartilhando deliciosas E, claro, obrigado pelo seu suor
La weekly Awesome! Its genuinely remarkable post, I have got much clear idea regarding from this post . La weekly
Real Estate I’m often to blogging and i really appreciate your content. The article has actually peaks my interest. I’m going to bookmark your web site and maintain checking for brand spanking new information.
Real Estate There is definately a lot to find out about this subject. I like all the points you made
Real Estate There is definately a lot to find out about this subject. I like all the points you made
Your blog is a true hidden gem on the internet. Your thoughtful analysis and engaging writing style set you apart from the crowd. Keep up the excellent work!
I’d always want to be update on new posts on this site, saved to favorites! .
Nice post. I learn something more challenging on different blogs everyday. It will always be stimulating to read content from other writers and practice a little something from their store. I’d prefer to use some with the content on my blog whether you don’t mind. Natually I’ll give you a link on your web blog. Thanks for sharing.
Wonderful web site Lots of useful info here Im sending it to a few friends ans additionally sharing in delicious And obviously thanks to your effort
My brother suggested I might like this blog. He was totally right. This post actually made my day. You can not imagine simply how much time I had spent for this information! Thanks!
Techarp You’re so awesome! I don’t believe I have read a single thing like that before. So great to find someone with some original thoughts on this topic. Really.. thank you for starting this up. This website is something that is needed on the internet, someone with a little originality!
Simply Sseven You’re so awesome! I don’t believe I have read a single thing like that before. So great to find someone with some original thoughts on this topic. Really.. thank you for starting this up. This website is something that is needed on the internet, someone with a little originality!
mulantogel
Right now it seems like Expression Engine is the preferred blogging platform out
there right now. (from what I’ve read) Is that what you are using on your blog?
Hi, I think your site might be having browser compatibility issues. When I look at your website in Safari, it looks fine but when opening in Internet Explorer, it has some overlapping. I just wanted to give you a quick heads up! Other then that, fantastic blog!
idlixmusicallydownpgbetpurislotbro138visa4dpulau88protogelsurgaplayhoktotopandora88operatotompoidbromo77pulsa777rajawd777onic4dzeus138jpslottumi123slot88kuwolestogelsensa138virus4didcash88mpo500topcer88casaprizetribun855sogoslotkarirtotogorila4dstake88instaslot88pvjbetsavefrom tiktokdetik288233 leyuanmpo2121giga5000snack video downloaderliveomekpantaislotelanggamebabyslotsupermpoliga788ombak1238278 slot
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: сервисные центры по ремонту техники в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
anichin
Hi, I do think this is a great web site. I stumbledupon it 😉 I am going to revisit once
again since I book-marked it. Money and freedom is the greatest way to change, may you
be rich and continue to guide other people.
Профессиональный сервисный центр по ремонту фототехники в Москве.
Мы предлагаем: ремонт студийных вспышек
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Подробнее на сайте сервисного центра remont-vspyshek-realm.ru
Профессиональный сервисный центр по ремонту компьютероной техники в Москве.
Мы предлагаем: ремонт блоков компьютера
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту компьютерных блоков питания в Москве.
Мы предлагаем: ремонт блока питания цена
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Если кто ищет место, где можно выгодно купить раковины и ванны, рекомендую один интернет-магазин, который недавно открыл для себя. Они предлагают большой выбор сантехники и аксессуаров для ванной комнаты. Ассортимент включает различные модели, так что можно подобрать под любой стиль и размер помещения.
Мне нужно было купить раковину , и они предложили несколько отличных вариантов. Цены приятно удивили, а качество товаров на высшем уровне. Также понравилось, что они предлагают услуги профессиональной установки. Доставка была быстрой, и всё прошло гладко. Теперь моя ванная комната выглядит просто великолепно!
Хочу поделиться своим опытом ремонта телефона в этом сервисном центре. Остался очень доволен качеством работы и скоростью обслуживания. Если ищете надёжное место для ремонта, обратитесь сюда: ремонт телефона поблизости.
<a href=”https://remont-kondicionerov-wik.ru”>ремонт кондиционеров на дому в москве</a>
tafsir mimpi 3d abjad tafsir mimpi 3d abjad tafsir mimpi 3d
abjad tafsir mimpi 3d abjad (https://northernfortplayhouse.com/)
Hello there! Quick question that’s entirely off
topic. Do you know how to make your site mobile friendly? My blog looks weird when viewing from my iphone.
I’m trying to find a template or plugin that might be
able to fix this issue. If you have any recommendations, please share.
Thanks!
mcm2 bank mandiri
Yes! Finally someone writes about live draw hk.
Ищите лучшее онлайн казино? В этом топе представлены надежные платформы для игр на деньги и быстрым выводом на карту. С лицензией, официальные зеркала. Бонусы и фриспины. казино топ онлайн cacnjrrnxg … resol 1 онлайн казино топ-89085 | res4 онлайн казино топ-89085 | | demo5 онлайн казино топ-89085 | telegraph что такое онлайн казино онлайн казино топ-89085
Профессиональный сервисный центр по ремонту компьютероной техники в Москве.
Мы предлагаем: качественный ремонт компьютеров
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту фото техники от зеркальных до цифровых фотоаппаратов.
Мы предлагаем: проектор ремонт
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту камер видео наблюдения по Москве.
Мы предлагаем: ремонт камер наблюдения
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: сервис центры бытовой техники нижний новгород
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: сервисные центры в перми
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Если вы искали где отремонтировать сломаную технику, обратите внимание – ремонт техники в тюмени
demo slot pg demo slot pg demo slot pg demo
slot pg
When I initially commented I clicked the
“Notify me when new comments are added” checkbox and now each
time a comment is added I get three e-mails with the same comment.
Is there any way you can remove people from that service?
Thank you!
Если вы искали где отремонтировать сломаную технику, обратите внимание – профи услуги
kdg789 kdg789 kdg789 kdg789
Профессиональный сервисный центр по ремонту парогенераторов в Москве.
Мы предлагаем: надежный сервис ремонта парогенераторов
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
hondatoto hondatoto hondatoto hondatoto
Hurrah! After all I got a website from where
I be capable of truly obtain useful information concerning my study and
knowledge.
Если вы искали где отремонтировать сломаную технику, обратите внимание – профи ремонт
texas88 texas88 texas88 texas88 texas88
I do not even know the way I stopped up here,
however I thought this submit was once good.
I do not know who you’re but certainly you are going to a well-known blogger when you aren’t
already. Cheers!
ремонт техники профи в самаре
slot138 slot138 slot138 slot138 slot138
Magnificent website. Plenty of helpful information here.
I am sending it to a few buddies ans additionally sharing in delicious.
And obviously, thanks to your sweat!
ремонт кондиционеров на дому в москве
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем:сервисные центры по ремонту техники в ростове на дону
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
сервисный центре предлагает ремонт телевизора москва – телемастер на дом москва
Профессиональный сервисный центр по ремонту компьютеров и ноутбуков в Москве.
Мы предлагаем: ремонт macbook
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Если вы искали где отремонтировать сломаную технику, обратите внимание – ремонт цифровой техники воронеж
Профессиональный сервисный центр по ремонту кондиционеров в Москве.
Мы предлагаем: сервисный центр кондиционеров
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту моноблоков в Москве.
Мы предлагаем: замена экрана моноблока цена
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту гироскутеров в Москве.
Мы предлагаем: ремонт гироскутера на дому
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
win88 win88 win88
I’m no longer certain the place you’re getting your info, but good topic.
I must spend a while finding out much more or figuring out more.
Thanks for wonderful information I used to be in search of this info for my mission.
Профессиональный сервисный центр по ремонту плоттеров в Москве.
Мы предлагаем: цены на ремонт плоттеров
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту объективов в Москве.
Мы предлагаем: ремонт объективов в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту сетевых хранилищ в Москве.
Мы предлагаем: цены на ремонт сетевых хранилищ
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: сервисные центры в волгограде
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту планшетов в Москве.
Мы предлагаем: замена экрана планшета цена
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: ремонт крупногабаритной техники в воронеже
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту моноблоков iMac в Москве.
Мы предлагаем: сервис по ремонту аймаков
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Sky Scarlet I very delighted to find this internet site on bing, just what I was searching for as well saved to fav
dodb buzz Great information shared.. really enjoyed reading this post thank you author for sharing this post .. appreciated
Masalqseen I just like the helpful information you provide in your articles
Профессиональный сервисный центр ремонт смартфонов в москве сервис смартфонов в ростове
Профессиональный сервисный центр по ремонту сотовых телефонов в Москве.
Мы предлагаем: ремонт телефонов недорого
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем: ремонт крупногабаритной техники в барнауле
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту сотовых телефонов в Москве.
Мы предлагаем: сервис ноутбуков
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
bola88 bola88
bola88
Hi! This is kind of off topic but I need some help from an established blog.
Is it hard to set up your own blog? I’m not very
techincal but I can figure things out pretty fast.
I’m thinking about making my own but I’m not sure where to begin.
Do you have any ideas or suggestions? Many thanks
Профессиональный сервисный центр по ремонту духовых шкафов в Москве.
Мы предлагаем: замена дверцы духового шкафа
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Полезный сервис быстрого загона ссылок сайта в индексация поисковой системы – быстрая индексация ссылок
Wow, superb blog layout! How long have you been blogging for?
you made blogging look easy. The overall look of your site is magnificent, let alone the content!
Keep up the fantastic work! Kalorifer Sobası odun, kömür, pelet gibi yakıtlarla çalışan ve ısıtma işlevi gören bir soba türüdür. Kalorifer Sobası içindeki yakıtın yanmasıyla oluşan ısıyı doğrudan çevresine yayar ve aynı zamanda suyun ısınmasını sağlar.
Thinker Pedia Hi there to all, for the reason that I am genuinely keen of reading this website’s post to be updated on a regular basis. It carries pleasant stuff.
Thinker Pedia I just like the helpful information you provide in your articles
Профессиональный сервисный центр по ремонту сотовых телефонов в Москве.
Мы предлагаем: ноутбук ремонт
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Профессиональный сервисный центр по ремонту моноблоков iMac в Москве.
Мы предлагаем: ремонт imac москва
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Sportsurge I do not even understand how I ended up here, but I assumed this publish used to be great
срочный ремонт телефонов рядом
Link exchange is nothing else except it is just placing the other person’s blog link on your page at proper place and other person will
also do similar for you.
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
Тут можно преобрести сейф огнестойкий в москве сейф жаростойкий
Тут можно преобрести сейфы для оружия пистолетные магазин оружейных сейфов
Тут можно преобрести шкаф оружейный купить сейф для оружия от производителя
Тут можно преобрести сейф под ружье купить оружейные шкафы и сейфы
Тут можно преобрести несгораемый сейф огнестойкий сейф купить
I genuinely enjoy studying on this site, it contains excellent articles. “When a man’s willing and eager, the gods join in.” by Aeschylus.
Your article helped me a lot, is there any more related content? Thanks!