In this PythonTkinter tutorial, we will learn about how to create a Todo list in Python which help us to store our important ideas and daily task.
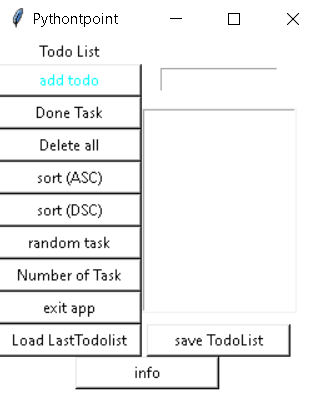
Python Tkinter Todo List
We create a Python Tkinter Todo List we have an interface with an entry box where we can enter the task and also have a button from which we can save our task.
Block of code:
In the following Python Tkinter Todo list block of code, we import some Tkinter modules from which we can create a Todo List.
from tkinter import *
import tkinter
import random
from tkinter import messagebox
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of code:
In the following Python Tkinter Todo List block of code, we will define an update task function in which we can update our task.
- clearlistbox() is used to clear the list box.
- lbltask.insert(“end”, utask) is used to insert the task inside the entry box.
- numtask = len(tasks) is used to describe the length of the task.
def updatetask():
clearlistbox()
for utask in tasks:
lbltask.insert("end", utask)
numtask = len(tasks)
lbldsp_count['text'] = numtask
Read: Python Tkinter temperature converter
Block of code:
In the following block of code, we define a clear list box function in which the task is deleted from the list.
def clearlistbox():
lbltask.delete(0, "end")
Block of code:
In the following block of code, we define the add task function from which we can add our important information to the list.
def addtask():
lbldisplay["text"] = ""
Ntask = txtinput.get()
if Ntask != "":
tasks.append(Ntask)
updatetask()
else:
lbldisplay["text"] = "please enter the text"
txtinput.delete(0, 'end')
Block of code:
In the following block of code, we create a delete all function in which we can delete all the information that we can enter into the list. And also create a delete one function in which we can delete only one information that we are entered.
def deleteall():
conf = messagebox.askquestion(
'delet all??', 'are you sure to delete all task?')
print(conf)
if conf.upper() == "YES":
global tasks
tasks = []
updatetask()
else:
pass
def deleteone():
delt = lbltask.get("active")
if delt in tasks:
tasks.remove(delt)
updatetask()
Read: Python Tkinter Simple Calculator
Block of code:
In the following block of code, we create some functions in which we can sort our task in ascending and descending order. And also generate the number of tasks.
def sortasc():
tasks.sort()
updatetask()
def sortdsc():
tasks.sort(reverse=True)
updatetask()
def randomtsk():
randtask = random.choice(tasks)
lbldisplay["text"] = randtask
def numbertsk():
numtask = len(tasks)
lbldisplay["text"] = numtask
Block of code:
In the following block of code, we create a save function from which we can save our task by simply clicking on the button and our task is saved into the Todolist.
def saveact():
savecon = messagebox.askquestion(
'Save confirmation', 'Save Your Progress?')
if savecon.upper() == "YES":
with open("SaveFile.txt", "w") as filehandle:
for listitem in tasks:
filehandle.write('%s\n' % listitem)
else:
pass
Block of code:
In the following block of code, we create a function load info and load act from which we can load our info into the list and also create a loadact function from which message box is generated if some wrong info enter.
def loadinfo():
messagebox.showinfo(
"info", "This is Todolist \n created by Pythontpoint ",)
def loadact():
loadcon = messagebox.askquestion(
'Save Confirmation', 'save your progress?')
if loadcon.upper() == "YES":
tasks.clear()
with open('SaveFile.txt', 'r') as filereader:
for line in filereader:
currentask = line
tasks.append(currentask)
updatetask()
else:
pass
Block of code:
In the following block of code, we create an exitapp function from which we can exit the app after completing the task.
def exitapp():
confex = messagebox.askquestion(
'Quit confirmation', 'Are you sue you want to quit?')
if confex.upper() == "YES":
wd.destroy()
else:
pass
Code:
In the following code, we will import the tkinter module and create a Todo list in which we can create our notes and add to the list.
from tkinter import *
import tkinter
import random
from tkinter import messagebox
def updatetask():
clearlistbox()
for utask in tasks:
lbltask.insert("end", utask)
numtask = len(tasks)
lbldsp_count['text'] = numtask
def clearlistbox():
lbltask.delete(0, "end")
def addtask():
lbldisplay["text"] = ""
Ntask = txtinput.get()
if Ntask != "":
tasks.append(Ntask)
updatetask()
else:
lbldisplay["text"] = "please enter the text"
txtinput.delete(0, 'end')
def deleteall():
conf = messagebox.askquestion(
'delet all??', 'are you sure to delete all task?')
print(conf)
if conf.upper() == "YES":
global tasks
tasks = []
updatetask()
else:
pass
def deleteone():
delt = lbltask.get("active")
if delt in tasks:
tasks.remove(delt)
updatetask()
def sortasc():
tasks.sort()
updatetask()
def sortdsc():
tasks.sort(reverse=True)
updatetask()
def randomtsk():
randtask = random.choice(tasks)
lbldisplay["text"] = randtask
def numbertsk():
numtask = len(tasks)
lbldisplay["text"] = numtask
def saveact():
savecon = messagebox.askquestion(
'Save confirmation', 'Save Your Progress?')
if savecon.upper() == "YES":
with open("SaveFile.txt", "w") as filehandle:
for listitem in tasks:
filehandle.write('%s\n' % listitem)
else:
pass
def loadinfo():
messagebox.showinfo(
"info", "This is Todolist \n created by Pythontpoint ",)
def loadact():
loadcon = messagebox.askquestion(
'Save Confirmation', 'save your progress?')
if loadcon.upper() == "YES":
tasks.clear()
with open('SaveFile.txt', 'r') as filereader:
for line in filereader:
currentask = line
tasks.append(currentask)
updatetask()
else:
pass
def exitapp():
confex = messagebox.askquestion(
'Quit confirmation', 'Are you sue you want to quit?')
if confex.upper() == "YES":
wd.destroy()
else:
pass
wd = tkinter.Tk()
wd.configure(bg="white")
wd.title("Pythontpoint")
wd.geometry("260x300")
tasks = []
lbltitle = tkinter.Label(wd, text="Todo List", bg="white")
lbltitle.grid(row=0, column=0)
lbldisplay = tkinter.Label(wd, text="", bg="white")
lbldisplay.grid(row=0, column=1)
lbldsp_count = tkinter.Label(wd, text="", bg="white")
lbldsp_count.grid(row=0, column=3)
txtinput = tkinter.Entry(wd, width=15)
txtinput.grid(row=1, column=1)
txtadd_button = tkinter.Button(
wd, text="add todo", bg="white", fg="cyan", width=15, command=addtask)
txtadd_button.grid(row=1, column=0)
delonebutton = tkinter.Button(
wd, text="Done Task", bg="white", width=15, command=deleteone)
delonebutton.grid(row=2, column=0)
delallbutton = tkinter.Button(
wd, text="Delete all", bg="white", width=15, command=deleteall)
delallbutton.grid(row=3, column=0)
sortasc = tkinter.Button(wd, text="sort (ASC)",
bg="White", width=15, command=sortasc)
sortasc.grid(row=4, column=0)
sortdsc = tkinter.Button(wd, text="sort (DSC)",
bg="White", width=15, command=sortdsc)
sortdsc.grid(row=5, column=0)
randombutton = tkinter.Button(
wd, text="random task", bg="White", width=15, command=randomtsk)
randombutton.grid(row=6, column=0)
numbertsk = tkinter.Button(
wd, text="Number of Task", bg="white", width=15, command=numbertsk)
numbertsk.grid(row=7, column=0)
exitbutton = tkinter.Button(wd, text="exit app",
bg="white", width=15, command=exitapp)
exitbutton.grid(row=8, column=0)
savebutton = tkinter.Button(
wd, text="save TodoList", bg="white", width=15, command=saveact)
savebutton.grid(row=10, column=1)
loadbutton = tkinter.Button(
wd, text="Load LastTodolist", bg="white", width=15, command=loadact)
loadbutton.grid(row=10, column=0)
infobutton = tkinter.Button(
wd, text="info", bg="white", width=15, command=loadinfo)
infobutton.grid(row=11, column=0, columnspan=2)
lbltask = tkinter.Listbox(wd)
lbltask.grid(row=2, column=1, rowspan=7)
# main loop
wd.mainloop()
Output:
After running the above code we get the following output in which we can see that the Todo list is created on the screen.
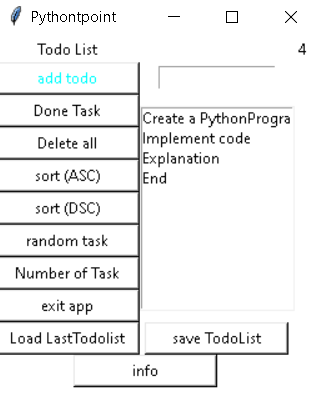
