In this tutorial, we will learn about how to make a simple calculator using Python Tkinter and also see the execution done by the calculator.
Python Tkinter Simple Calculator
A calculator is a small electronic device with a keyboard that permits people to do mathematical operations more easily. The calculator will add, subtract, multiply, and square root the numbers more easily.
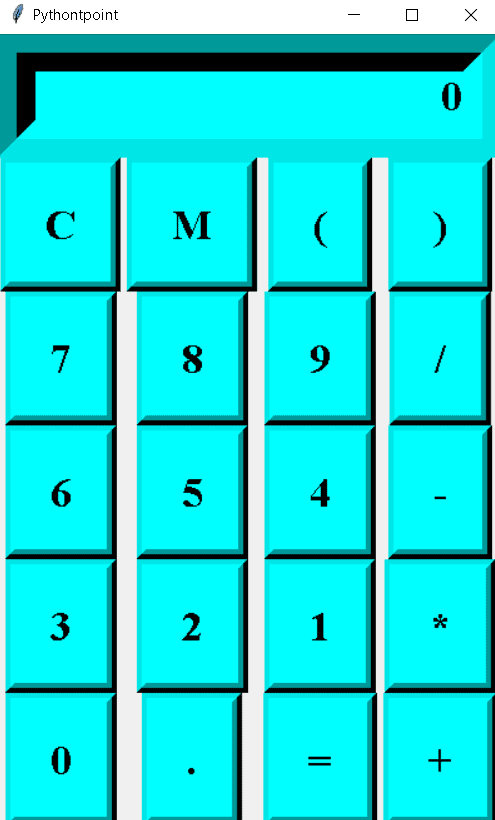
Github Link
Check this code in Repository from Github and you can also fork this code.
Github User Name: PythonT-Point
Block of code:
In this Python Tkinter block of code, we will import the Tkinter module from which we can create a simple calculator.
- def buttonclick(numbers): is used to define the button click function.
- operator=operator + str(numbers) is used as an operator.
- textinput.set(operator) is used to set the text input operator.
from tkinter import *
import tkinter
def buttonclick(numbers):
global operator
operator=operator + str(numbers)
textinput.set(operator)
Block of code:
In this Python Tkinter block of code, we will define the clear function from which we clear the display.
def buttonClearDisplay():
global operator
operator=""
textinput.set("")
Read: Stone Paper Scissor using Python Tkinter
Block of code:
In this block of code, we will define the equal to function. This function is used when the user enters the input and applied some operation after applying the operation they want the exact and display on the screen then they click the equal to the button.
def buttonEqualsInput():
global operator
sumup=str(eval(operator))
textinput.set(sumup)
operators=""
Block of code:
In this block of code, we will create a window and after that give the title to the window and start making the calculator.
calculator = Tk()
calculator.title("Pythontpoint")
operator = ""
textinput = StringVar()
textdisplay = Entry(calculator,font=('Times New Roman', 25,'bold'), textvariable=textinput, bd=30, insertwidth=4,
bg="cyan", justify='right').grid(columnspan=4)
Block of code:
In this Python Tkinter block of code, we create buttons to make a calculator and do some operations with the help of buttons.
- buttonclear=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”C”,bg=”cyan”,command=buttonClearDisplay).grid(row=1,column=”0″) is used to clear the display os the calculator.
- Buttonbraket1=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text(“,bg=”cyan”,command=lambda:buttonclick((“)).grid(row=1,column=”2”) is used to create the open bracket1.
- Buttonbracket2=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times NewRoman’,25,’bold’),text=”)”,bg=”cyan”,command=lambda:buttonclick(“)”)).grid(row=1,column=”3″) is used to create the open bracket2.
- button7=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”7″,bg=”cyan”,command=lambda:buttonclick(7)).grid(row=2,column=”0″) is used to create the number 7 button.
- button8=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”8″,bg=”cyan”,command=lambda:buttonclick(8)).grid(row=2,column=”1″) is used to create the button 8.
- Division=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”/”,bg=”cyan”,command=lambda:buttonclick(“/”)).grid(row=2,column=”3″) is used to create a division button to apply divide operation on the operators.
buttonclear=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="C",bg="cyan",command=buttonClearDisplay).grid(row=1,column="0")
ButtonM=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="M",bg="cyan",).grid(row=1,column="1")
Buttonbraket1=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="(",bg="cyan",command=lambda:buttonclick("(")).grid(row=1,column="2")
Buttonbracket2=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text=")",bg="cyan",command=lambda:buttonclick(")")).grid(row=1,column="3")
button7=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="7",bg="cyan",command=lambda:buttonclick(7)).grid(row=2,column="0")
button8=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="8", bg="cyan",command=lambda:buttonclick(8)).grid(row=2,column="1")
button9=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="9", bg="cyan",command=lambda:buttonclick(9)).grid(row=2,column="2")
Division=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="/",bg="cyan",command=lambda:buttonclick("/")).grid(row=2,column="3")
Block of code:
In this Python Tkinter block of code, we create some buttons from which we can easily do some mathematics operations.
- subtraction=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=””,bg=”cyan”,command=lambda:buttonclick(“”)).grid(row=3,column=”3″) is used to subract the two numbers and get the exact answer.
- Multiplication=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”*”,bg=”cyan”,command=lambda:buttonclick(“*”)).grid(row=4,column=”3″) is used to do some multiplication operations.
- Dot=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’, 25,’bold’),text=”.”,bg=”cyan”,command=lambda:buttonclick(“.”)).grid(row=5,column=”1″) is used to put the dot between the numbers.
- Addition=Button(calculator,padx=16,pady=16,bd=8, fg=”black”,font=(‘Times New Roman’,25,’bold’),text=”+”,bg=”cyan”,command=lambda:buttonclick(“+”)).grid(row=5,column=”3″) is used to do some addition operation.
button6=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="6",bg="cyan",command=lambda:buttonclick(6)).grid(row=3,column="0")
button5=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="5",bg="cyan",command=lambda:buttonclick(5)).grid(row=3,column="1")
button4=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="4",bg="cyan",command=lambda:buttonclick(4)).grid(row=3,column="2")
subtraction=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="-",bg="cyan",command=lambda:buttonclick("-")).grid(row=3,column="3")
button3=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="3",bg="cyan",command=lambda:buttonclick(3)).grid(row=4,column="0")
button2=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="2",bg="cyan",command=lambda:buttonclick(2)).grid(row=4,column="1")
button1=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="1",bg="cyan",command=lambda:buttonclick(1)).grid(row=4,column="2")
Multiplication=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="*",bg="cyan",command=lambda:buttonclick("*")).grid(row=4,column="3")
Button0=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="0",bg="cyan",command=lambda:buttonclick(0)).grid(row=5,column="0")
Dot=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text=".",bg="cyan",command=lambda:buttonclick(".")).grid(row=5,column="1")
Equal=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="=",bg="cyan",command=buttonEqualsInput).grid(row=5,column="2")
Addition=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="+",bg="cyan",command=lambda:buttonclick("+")).grid(row=5,column="3")
Code:
In the following code, we create some functions from which we can make a Python Tkinter calculator for easy calculation which makes the user’s work easier.
from tkinter import *
import tkinter
def buttonclick(numbers):
global operator
operator=operator + str(numbers)
textinput.set(operator)
def buttonClearDisplay():
global operator
operator=""
textinput.set("")
def buttonEqualsInput():
global operator
sumup=str(eval(operator))
textinput.set(sumup)
operators=""
calculator = Tk()
calculator.title("Pythontpoint")
operator = ""
textinput = StringVar()
textdisplay = Entry(calculator,font=('Times New Roman', 25,'bold'), textvariable=textinput, bd=30, insertwidth=4,
bg="cyan", justify='right').grid(columnspan=4)
buttonclear=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="C",bg="cyan",command=buttonClearDisplay).grid(row=1,column="0")
ButtonM=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="M",bg="cyan",).grid(row=1,column="1")
Buttonbraket1=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="(",bg="cyan",command=lambda:buttonclick("(")).grid(row=1,column="2")
Buttonbracket2=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text=")",bg="cyan",command=lambda:buttonclick(")")).grid(row=1,column="3")
button7=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="7",bg="cyan",command=lambda:buttonclick(7)).grid(row=2,column="0")
button8=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="8", bg="cyan",command=lambda:buttonclick(8)).grid(row=2,column="1")
button9=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="9", bg="cyan",command=lambda:buttonclick(9)).grid(row=2,column="2")
Division=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="/",bg="cyan",command=lambda:buttonclick("/")).grid(row=2,column="3")
button6=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="6",bg="cyan",command=lambda:buttonclick(6)).grid(row=3,column="0")
button5=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="5",bg="cyan",command=lambda:buttonclick(5)).grid(row=3,column="1")
button4=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="4",bg="cyan",command=lambda:buttonclick(4)).grid(row=3,column="2")
subtraction=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="-",bg="cyan",command=lambda:buttonclick("-")).grid(row=3,column="3")
button3=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="3",bg="cyan",command=lambda:buttonclick(3)).grid(row=4,column="0")
button2=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="2",bg="cyan",command=lambda:buttonclick(2)).grid(row=4,column="1")
button1=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="1",bg="cyan",command=lambda:buttonclick(1)).grid(row=4,column="2")
Multiplication=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="*",bg="cyan",command=lambda:buttonclick("*")).grid(row=4,column="3")
Button0=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="0",bg="cyan",command=lambda:buttonclick(0)).grid(row=5,column="0")
Dot=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text=".",bg="cyan",command=lambda:buttonclick(".")).grid(row=5,column="1")
Equal=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="=",bg="cyan",command=buttonEqualsInput).grid(row=5,column="2")
Addition=Button(calculator,padx=16,pady=16,bd=8, fg="black",font=('Times New Roman', 25,'bold'),
text="+",bg="cyan",command=lambda:buttonclick("+")).grid(row=5,column="3")
calculator.mainloop()
Output:
After running the above code we get the following output in which we can see that a calculator is created from which the mathematical operation can be done more easily.
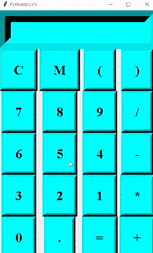
So, in this tutorial, we have illustrated how to make Python Tkinter a simple calculator. Moreover, we have also discussed the whole code used in this tutorial.
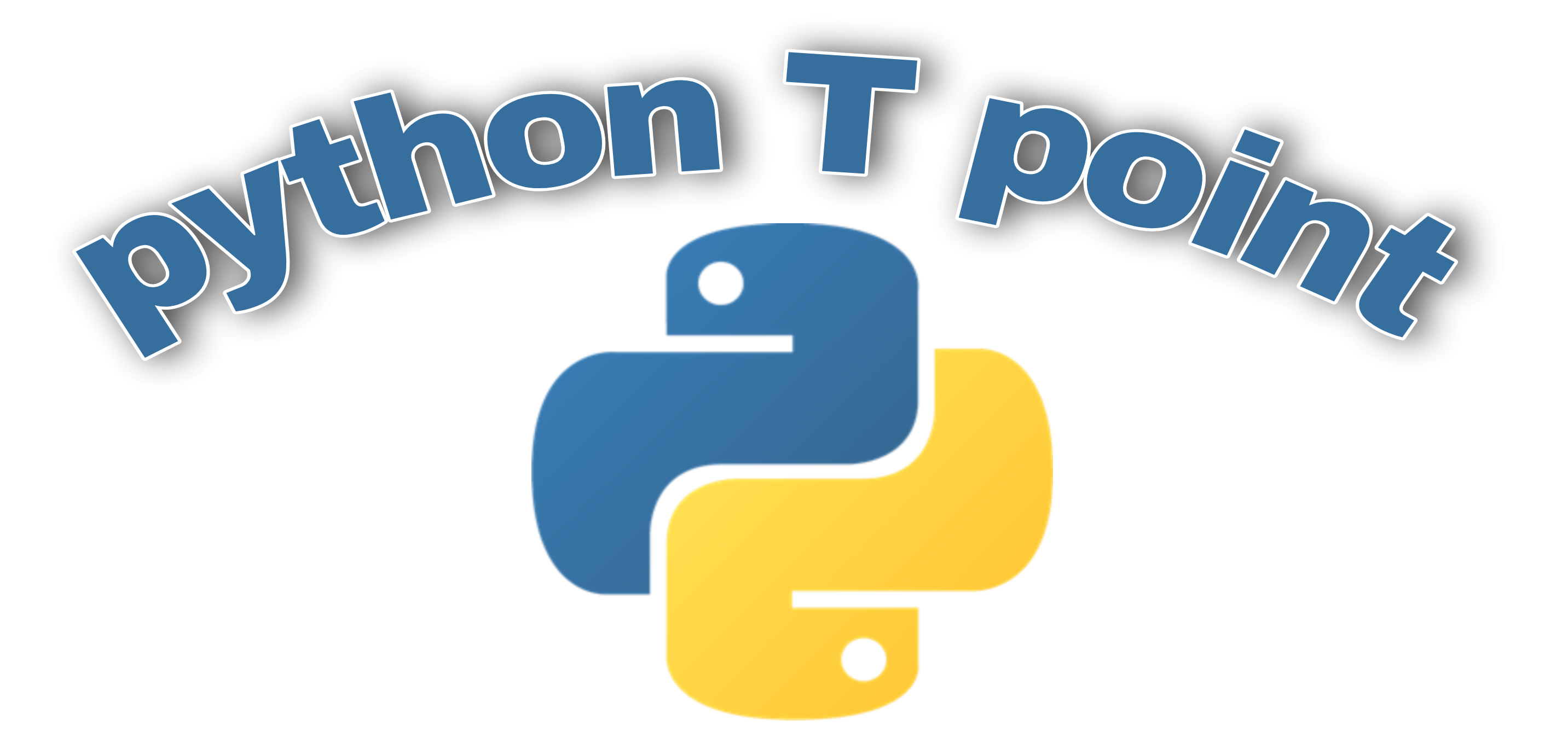
Comments are closed.